#include <stdio.h>
#include <ctype.h>
#define MAX_KEY 11
#define MAX_LEN 100
#define WORD 1
struct key
{
char *word;
int count;
} keytab[MAX_KEY] =
{
{“auto”, 0},
{“break”, 0},
{“case”, 0},
{“char”, 0},
{“const”, 0},
{“continue”, 0},
{“default”, 0},
{“unsigned”, 0},
{“void”, 0},
{“volatile”,},
{“while”, 0},
};
int state;
enum
{
CONST = 0X01,
SAID1 = 0X02,
SAID2 = 0X04,
FIRST = 0X08,
};
int getword(char *word, int max_len);
void state_deal(int type);
int binsearch(char *word);
main()
{
char word[MAX_LEN];
int type;
int n;
while ((type = getword(word, MAX_LEN)) != EOF)
{
state_deal(type);
if (state == 0 && type == WORD)
if ((n = binsearch(word)) != EOF)
keytab[n].count++;
}
for (n = 0; n < MAX_KEY; n++)
{
if (keytab[n].count)
printf("%s:\t%d\n", keytab[n].word, keytab[n].count);
}
return 0;
}
int getch(void);
void ungetch(int c);
int getword(char *word, int max_len)
{
int c, i = 0;
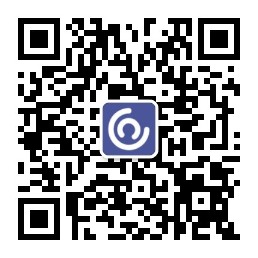
while ((c = getch()) == ' ' || c == '\t');
if (!isalpha(c) && c != '_')
return c;
while (isalnum(c) || c == '_')
{
word[i++] = c;
c = getch();
}
word[i] = '\0';
if (c != EOF)
ungetch(c);
return WORD;
}
#define MAX_BUFF 100
char buff[MAX_BUFF];
int buffp;
int getch(void)
{
return buffp > 0 ? buff[–buffp] : getchar();
}
void ungetch(int c)
{
if (buffp < MAX_BUFF)
buff[buffp++] = c;
else
printf(“ungetch: the buff is full!\n”);
}
void state_deal(int type)
{
static int marks, c;
if ((state & (SAID1 | SAID2 | FIRST)) == 0 && type == '\"')
marks++;
if (marks % 2 != 0)
state |= CONST;
else
state &= ~CONST;
if ((state & CONST) == 0 && c == '/' && type == '/')
state |= SAID1;
if ((state & SAID1) && type == '\n')
state &= ~SAID1;
if ((state & CONST) == 0 && c == '/' && type == '*')
state |= SAID2;
if ((state & SAID2) && c == '*' && type == '/')
state &= ~SAID2;
if (type == '#')
state |= FIRST;
if ((state & FIRST) && type == '\n')
state &= ~FIRST;
c = type;
}
int binsearch(char *word)
{
int cond;
int low, high, mid;
low = 0;
high = MAX_KEY - 1;
while (low <= high)
{
mid = (low + high) / 2;
if ((cond = strcmp(word, keytab[mid].word)) > 0)
low = mid + 1;
else if (cond < 0)
high = mid - 1;
else
return mid;
}
return EOF;
}