代码示例:
public static void main(String[] args) throws InterruptedException {
ExecutorService executor = Executors.newFixedThreadPool(4);
Semaphore semaphore = new Semaphore(2);
try {
CompletableFuture<String> futureA = CompletableFuture.supplyAsync(() -> {
try {
semaphore.acquire(); // 获取信号量,限制并发数
// 调用外部接口 A
System.out.println("A进入车库");
Thread.sleep(9000);
System.out.println("A离开车位");
return "A";
} catch (InterruptedException e) {
throw new RuntimeException(e);
} finally {
semaphore.release(); // 释放信号量
}
}, executor);
CompletableFuture<String> futureB = CompletableFuture.supplyAsync(() -> {
try {
semaphore.acquire(); // 获取信号量,限制并发数
// 调用外部接口 B
System.out.println("B进入车库");
Thread.sleep(9000);
System.out.println("B离开车位");
return "B";
} catch (InterruptedException e) {
throw new RuntimeException(e);
} finally {
semaphore.release(); // 释放信号量
}
}, executor);
CompletableFuture<String> futureC = CompletableFuture.supplyAsync(() -> {
try {
semaphore.acquire(); // 获取信号量,限制并发数
// 调用外部接口 C
System.out.println("C进入车库");
Thread.sleep(9000);
System.out.println("C离开车位");
return "C";
} catch (InterruptedException e) {
throw new RuntimeException(e);
} finally {
semaphore.release(); // 释放信号量
}
}, executor);
CompletableFuture<String> futureD = CompletableFuture.supplyAsync(() -> {
try {
semaphore.acquire(); // 获取信号量,限制并发数
// 调用外部接口 D
System.out.println("D进入车库");
Thread.sleep(9000);
System.out.println("D离开车位");
return "D";
} catch (InterruptedException e) {
throw new RuntimeException(e);
} finally {
semaphore.release(); // 释放信号量
}
}, executor);
CompletableFuture<Void> combinedFuture = CompletableFuture.allOf(futureA, futureB, futureC, futureD);
combinedFuture.thenAccept(result -> {
// 汇总数据
String dataA = futureA.join();
String dataB = futureB.join();
String dataC = futureC.join();
String dataD = futureD.join();
String combinedData = dataA + dataB + dataC + dataD;
System.out.println(combinedData);
});
} catch (Exception exception) {
exception.printStackTrace();
}
executor.shutdown();
}
打印结果:
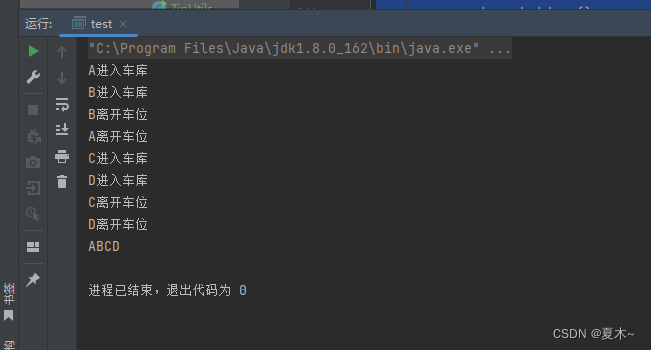