1、反转字符串
使用切片操作对字符串进行反转,这是比较直接有效的方式。 这也可以用来检测回文数。
str = "Hello World"
print("反转后字符串是:", str[::-1])
输出:
反转后字符串是: dlroW olleH
2、字符串列表组成单个字符串
使用join方法将字符串列表组成单个字符串。
list = ["Hello", "world", "Ok", "Bye!"]
combined_string = " ".join(list)
print(combined_string)
输出:
Hello world Ok Bye!
3、返回字典缺失键的默认值
字典中的get方法用于返回指定键的值,如果键不在字典中返回默认值 None 或者设置的默认值。
dict = {1:'one', 2:'two', 4:'four'}
#returning three as default value
print(dict.get(3, 'three'))
print("原始字典:", dict)
输出:
three
原始字典: {1: 'one', 2: 'two', 4: 'four'}
4、交换两个变量的值
在不使用临时变量的前提下,交换两个变量的值。
a, b = 5, 10
# 方法1
a, b = b, a
# 方法2
def swap(a,b):
return b,a
swap(a,b)
5、正则表达式
正则表达式用来匹配处理字符串,python中的re模块提供了全部的正则功能。
import re
text = "The rain in spain"
result = re.search("rain", text)
print(True if result else False)
输出:
True
6、筛选值
python中的filter方法可以用来进行值的筛选。
my_list = [0,1,2,3,6,7,9,11]
result = filter(lambda x: x % 2!=0, my_list)
print(list(result))
输出:
扫描二维码关注公众号,回复:
17278320 查看本文章
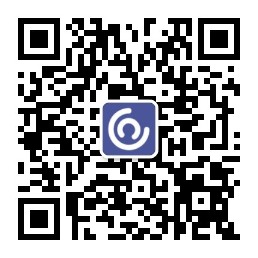
[1, 3, 7, 9, 11]
7、统计字频
判断字符串每个元素出现的次数,可以用collections模块中的Counter方法来实现,非常简洁。
from collections import Counter
result = Counter('banana')
print(result)
输出:
Counter({'a': 3, 'n': 2, 'b': 1})
8、变量的内存占用
如何输出python中变量的内存占用大小,可以通过sys模块来实现。
import sys
var1 = 15
list1 = [1,2,3,4,5]
print(sys.getsizeof(var1))
print(sys.getsizeof(list1))
输出:
28
104
9、链式函数调用
在一行代码中调用多个函数。
def add(a, b):
return a + b
def subtract(a, b):
return a - b
a, b = 5, 10
print((add if b > a else subtract)(a,b))
输出:
15
10、从列表中删除重复项
删除列表中重复项一般可以通过遍历来筛选去重,或者直接使用集合方法。
list1 = [1,2,3,3,4,'John', 'Ana', 'Mark', 'John']
# 方法1
def remove_duplicate(list_value):
return list(set(list_value))
print(remove_duplicate(list1))
# 方法2
result = []
[result.append(x) for x in list1 if x not in result]
print(result)
输出:
[1, 2, 3, 4, 'Ana', 'John', 'Mark']
[1, 2, 3, 4, 'John', 'Ana', 'Mark']