设计控制台应用程序,声明一个书本类Book,包含如下五个私有字段:书名,书的页数,书的作者(可能有多个作者,使用List<string>存储,看课本81页自学List<T>类),书的价格(单位为元),书的出版年份(用DateTime结构表示);类包含:书名、书的页数(必须大于0,在set访问器里控制这个约束)、书的价格(必须大于0)三个属性;设计其构造函数,包含默认构造函数和带有参数的构造函数;设计方法-返回书的价格(单位美元),假设1美元=6.886人民币元,将6.886表示为类的静态成员;定义输出书的信息的方法(使用this关键字),并在main函数中构造书本对象,并输出其信息;将私有字段改为public和protected,在main函数里面尝试直接访问字段信息,看会发生什么结果。
代码如下
using System;
using System.Collections.Generic;//使用List<T>,引入命名空间
using System.Linq;
using System.Text;
namespace 书本
{
class Program
{
static void Main(string[] args)
{
List<String> author2 = new List<string>();
author2.Add("one");
author2.Add("two");
Book b = new Book("格林童话", 12, author2, 22.3, System.DateTime.Now);
b.message();
Console.Read();
}
}
//书本类
public class Book{
private string bookname;
private int pagecount;
private List<string> author;//作者可以有多个
private double price;
private DateTime year;
public static double dollar = 6.886;//静态成员
public string BookName
{
set
{
bookname = value;
}
get
{
return bookname;
}
}
public int PageCount
{
set
{
if (value <= 0)
{
return;//价格小于等于0时不做处理
}
pagecount = value;
}
get
{
return pagecount;
}
}
public double Price
{
set
{
if(value <=0)
{
return;//价格小于等于0时不做处理
}
price = value;
}
get
{
return price;
}
}
public Book() { }
public Book(string bookname, int pagecount, List<String> author, double price, DateTime year)
{
this.bookname = bookname;
this.pagecount = pagecount;
this.author = author;
this.price = price;
this.year = year;
}
//返回书的价格美元
public double getPrice()
{
return price / dollar;
}
//输出书的信息
public void message()
{
Console.WriteLine("书名:"+this.BookName+"\n页数:"+this.PageCount+"\n作者:");
foreach (string s in author)
{
Console.WriteLine(s+" ");
}
Console.WriteLine("价格:"+this.getPrice().ToString("F2")+"美元\n出版年份:"+this.year.Year.ToString()+"年");
}
}
}
运行结果
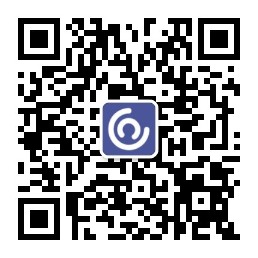