If a machine can save only 3 significant digits, the float numbers 12300 and 12358.9 are considered equal since they are both saved as 0 with simple chopping. Now given the number of significant digits on a machine and two float numbers, you are supposed to tell if they are treated equal in that machine.
Input Specification:
Each input file contains one test case which gives three numbers N, A and B, where N (<) is the number of significant digits, and A and B are the two float numbers to be compared. Each float number is non-negative, no greater than 1, and that its total digit number is less than 100.
Output Specification:
For each test case, print in a line YES
if the two numbers are treated equal, and then the number in the standard form 0.d[1]...d[N]*10^k
(d[1]
>0 unless the number is 0); or NO
if they are not treated equal, and then the two numbers in their standard form. All the terms must be separated by a space, with no extra space at the end of a line.
Note: Simple chopping is assumed without rounding.
Sample Input 1:
3 12300 12358.9
Sample Output 1:
YES 0.123*10^5
Sample Input 2:
3 120 128
Sample Output 2:
NO 0.120*10^3 0.128*10^3
题意:
给出两个数,问:将他们写成保留N (<100)位小数的科学计数法 0.d[1]...d[N]*10^k
(d[1]
>0 unless the number is 0); 后,是否相同。若相等,输出YES,并转换结果; 如果不相等,输出NO,并给出两个数的转换结果。
题解:
本题的思路难想而且情况判断非常复杂。
题目要求科学计数法时,两个数是否相等。因此只要判断科学技术法时的本体部分以及指数部分是否相等即可。
对于数据来说,要分为大于 1 与小于 1 来判断,要考虑各种情况下的数字,比如:0000, 000.00, 00123.4, 0.012, 0.00 等
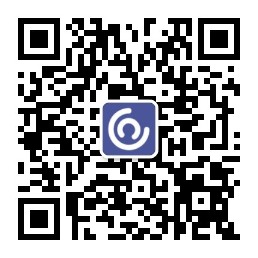
一开始没有考虑太多的异常情况,拿了19分,第二天看了题解的注意点重做,还是只有21分,
第三天理了理思路,其实,只要关注,格式化好后的小数点要点在哪里,次数是多少就可以了,在此基础上,特判0,去掉前导零。比较的时候注意不要超限,输出不够0补齐。
自己编的测试样例:
2 0.0015 0000.001520000 YES 0.15*10^-2
3 010.25 0010.23 YES 0.102*10^2
5 0.1 00.100 YES 0.10000*10^0
10 000.0012 0000000.0012000000000 YES 0.1200000000*10^-2
3 0 0.000 YES 0.000*10^0
AC代码:
#include<iostream> #include<string> #include<cstring> #include<cmath> #include<algorithm> using namespace std; int n; char a[105]; char b[105]; int main(){ cin>>n>>a>>b; int la=strlen(a); int lb=strlen(b); int sta=-1,stb=-1; int isa0=0,isb0=0;//特判是不是0 //从第一个不是0的数开始 for(int i=0;i<la;i++){ if(a[i]!='.'&&a[i]!='0'){ sta=i; break; } } for(int i=0;i<lb;i++){ if(b[i]!='.'&&b[i]!='0'){ stb=i; break; } } if(sta==-1) isa0=1; if(stb==-1) isb0=1; //小数点前有几位 int ia=0,ib=0; int f=0; for(int i=0;i<la;i++){ if(a[i]=='.') break; if(a[i]!='0') f=1; if(f){ ia++; } } f=0; for(int i=0;i<lb;i++){ if(b[i]=='.') break; if(b[i]!='0') f=1; if(f){ ib++; } } //cout<<"sta:"<<sta<<" ia:"<<ia<<" ha:"<<ha<<endl; //cout<<"stb:"<<stb<<" ib:"<<ib<<" hb:"<<hb<<endl; //比较 f=1; int pa=sta,pb=stb; if(isa0!=isb0 || ia!=ib) f=0; for(int i=0;i<n;i++){ if(pa+i>=la||pb+i>=lb) break; if(a[pa]=='.') pa++; if(b[pb]=='.') pb++; //cout<<"pa "<<pa+i<<" a[pa] "<<a[pa+i]<<endl; //cout<<"pb "<<pa+i<<" b[pb] "<<b[pa+i]<<endl; if(a[pa+i]!=b[pb+i]){ f=0; break; } } if(isa0==1&&isa0==isb0) f=1;//如果两个都是0 //输出 if(f){ cout<<"YES 0."; if(isa0==1){//0的特判 for(int i=1;i<=n;i++) cout<<"0"; cout<<"*10^0"; }else{ pa=sta; for(int i=0;i<n;i++){ if(pa+i>=la) { cout<<"0"; continue; } if(a[pa+i]=='.') pa++; if(pa+i>=la) cout<<"0"; else cout<<a[pa+i]; } cout<<"*10^"; if(ia!=0) cout<<ia;//次数>=于0 else{//次数<0 int k=-1; for(int i=0;i<la;i++){ if(a[i]=='.'){ k=i; break; } } cout<<k-sta+1;//.的位置-开始的位置+1 } } }else{//同上 cout<<"NO 0."; if(isa0==1){ for(int i=1;i<=n;i++) cout<<"0"; cout<<"*10^0 "; }else{ for(int i=0;i<n;i++){ if(pa+i>=la) { cout<<"0"; continue; } if(a[pa+i]=='.') pa++; if(pa+i>=la) cout<<"0"; else cout<<a[pa+i]; } cout<<"*10^"; if(ia!=0) cout<<ia<<" 0."; else{ int k=-1; for(int i=0;i<la;i++){ if(a[i]=='.'){ k=i; break; } } cout<<k-sta+1<<" 0."; } } if(isb0==1){ for(int i=1;i<=n;i++) cout<<"0"; cout<<"*10^0"; }else{ for(int i=0;i<n;i++){ if(pb+i>=lb) { cout<<"0"; continue; } if(b[pb+i]=='.') pb++; if(pb+i>=lb) cout<<"0"; else cout<<b[pb+i]; } cout<<"*10^"; if(ib!=0) cout<<ib; else{ int k=-1; for(int i=0;i<lb;i++){ if(b[i]=='.'){ k=i; break; } } cout<<k-stb+1; } } } return 0; }