目录
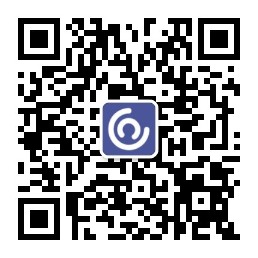
一、基础知识
1.Python 与java,C++的主要区别
与 C++ 和 Java 不同,Python 中变量没有类型,更谈不上声明变量类型,变量只是对象的引用,而 Python 的所有类型都是对象,包括函数、模块、数字、字符串、列表、元组、字典等。
如下所示,定义变量 a 并赋值,同一个变量在同一段程序中三次被赋值不同的类型,因为变量没有类型,它可以指向任何类型。
a = 12
print("a=", a)
a = "ABCDE"
print("a=", a)
a = 1 + 4j
print("a=", a)
执行结果: a= 12 a= ABCDE a= 1+4j
2.通过命令运行 Python 脚本:
运行Python 脚本的方法很多,除了IDE,还可以直接以命令的方式运行。
Windows 系统:
进入 Python 脚本 test.py 所在路径下,键入命令:Python test.py。
注意:Python 首字母大写。
Linux 或 Unix 系统:
进入 Python 脚本 test.py 所在路径下,键入命令:python test.py。
注意:Python 首字母小写。
3.数字
Python 提供了三种数字类型,即 int(整型),float(浮点型),complex(复数)。
a.数字类型转换
Python 的三种数字类型可以进行相互转换,转换方式为:数字类型+圆括号,比如:
a = 123
b = 3.1415926
print("int(b)=",int(b))
print("float(a)=",float(a))
print("complex(a)=",complex(a))
print("complex(a,b)=",complex(a,b))
b.常用的数学函数
Python 提供了丰富的数学函数以降低编程实现的难度,介绍一些常用的函数:
import math
#求绝对值:abs(x)
print("abs(-12)=",abs(-12))
#向上取整:ceil(x)
print("ceil(3.1415)=",math.ceil(3.1415))
#向下取整:floor(x)
print("floor(3.678)=",math.floor(3.678))
#四舍五入:round(x)
print("round(3.678)=",round(3.678))
#乘方运算:pow(x,y),x的y次方
print("pow(3,4)=",pow(3,4))
#求平方根:sqrt(x)
print("sqrt(144)=",math.sqrt(144))
4.运算符
Python 运算符大致可分为7种,即算术运算符、比较运算符、赋值运算符、逻辑运算符、位运算符、成员运算符以及身份运算符。
1.算术运算符
算术运算有7种:加(+)、减(-)、乘(*)、除(/)、取模(%)、幂运算(**)和取整预算(//)。
2.比较运算符
6种比较运算符:>(大于),<(小于),==(等于),!=(不等于),>=(大于等于),<=(小于等于)。
3.赋值运算
将简单的赋值运算(=)与算术运算结合:+=、-=、*=、/=、%=、**=、//=。
4.逻辑运算
3种逻辑运算符分别为:and(与),or(或),not(非)
5.位运算
6种:
&:按位与运算符,|:按位或运算符,^:按位异或运算符,~:按位取反运算符,>>
:右移动运算符,<<
:左移动运算符
6.成员运算
变量 in 数据结构 (数据结构接下来会讲)
7.身份运算符
包含两个运算符:is 和 is not,分别用于判断两个标识符是否引用自一个对象。关于存储单元的比较,涉及到对内存模型的理解,暂不做详述。
二、数据结构
1.字符串
1.创建字符串
创建字符串非常简单,只需要为变量赋值即可。
str1 = "Hello world!"
str2 = "ABCDEFG"
2.访问字符串中的元素
一个字符串可以看成若干个字符元素组成,Python 中可以通过索引来访问字符串中的元素,也可以访问子串。
str1 = "Hello world!"
#访问字符串中的单个字符或者子串
print('str1:',str1)
print('str1[0]:',str1[0])
print('str1[0:4]:',str1[0:5])
print('str1[5:9]:',str1[6:11])
执行结果:
str1: Hello world!
str1[0]: H
str1[0:4]: Hello
str1[5:9]: world
3.修改字符串
Python 字符串修改需要通过内建 replace(old,new) 方法,不支持直接通过索引对已有内容的修改,此外,还支持对字符串拼接。如下例子:
str1 = "Hi!"
str2 = "Jack"
#通过拼接修改字符串
str1 = str1 + str2
print('After splicing str1:',str1)
#通过替换修改字符串:replace(old,new)
str1 = str1.replace(str2, "Tom")
print('After replacement str1:',str1)
执行结果:
After splicing str1: Hi!Jack
After replacement str1: Hi!Tom
4.字符串运算符
字符串是一种非常灵活的数据结构,可以进行拼接、剪切、复制等操作。如下例子:
str1 = "Hello world!"
str2 = "Jack"
#字符串拼接
print('str1+str2:',str1+str2)
#字符串截取
print('str1[0:6]:',str1[0:6])
#字符串复制
print('str2*3:',str2*3)
#成员运算:判断一个字符串是否包含某成员
print('world in str1?','world' in str1)
print('word in str1?','word' in str1)
执行结果:
str1+str2: Hello world!Jack
str1[0:6]: Hello
str2*2: JackJackJack
world in str1? True
word in str1? False
5.字符串格式化
和 C/C++ 一样,Python 也支持字符串的格式化输出,格式化的语法与 C 语言基本是一样的。
#格式化为十进制:%d
print('PI is approximately equal to %d (I)'%(3.1415926))
#格式化字符串:%s
print('PI is approximately equal to %s (II)'%(3.1415926))
#格式化浮点数字,可指定小数点后的精度,默认为6位小数:%f
print('PI is approximately equal to %f (III)'%(3.1415926))
#格式化浮点数字,指定n位小数:%.nf
print('PI is approximately equal to %.2f (IV)'%(3.1415926))
#用科学计数法格式化浮点数:%e
print('PI is approximately equal to %e (V)'%(3.1415926))
#格式化为十进制:%d
print('The road is about %d meters long (VI)'%(1234))
#格式化无符号八进制数:%d
print('The road is about %o meters long (VII)'%(1234))
#格式化无符号十六进制数:%x
print('The road is about %x meters long (VIII)'%(1234))
执行结果:
PI is approximately equal to 3 (I)
PI is approximately equal to 3.1415926 (II)
PI is approximately equal to 3.141593 (III)
PI is approximately equal to 3.14 (IV)
PI is approximately equal to 3.141593e+00 (V)
The road is about 1234 meters long (VI)
The road is about 2322 meters long (VII)
The road is about 4d2 meters long (VIII)
6.字符串内建函数
Python 为字符串提供了40余个内建函数,介绍常用的几个方法。
str1 = " Hello world! Hello"
str2 = "Hello"
#返回字符串的长度:len(str)
print('str1的长度:',len(str1))
#对字符串进行分割:split(str),分割符为str,此处以空格进行分割
print('str1以空格分割的结果:',str1.split(' '))
#删除字符串字符串首尾的空格
print('str1删除首尾空格:',str1.strip())
#count(str2, beg>=0,end<=len(str1))
#返回 str2 在 str1 里面出现的次数,如果 beg 或者 end 指定则返回指定范围内 str2出现的次数
print('str2在str1中出现的次数:',str1.count(str2))
print('str2在str1中出现的次数(指定范围):',str1.count(str2,10,20))
#检查字符串是否以 obj 结束,如果beg 或者 end 指定则检查指定的范围内是否以 obj 结束
#如果是,返回 True,否则返回 False.
print('str1是否以 str2结尾:',str1.endswith(str2))
print('str1是否以 str2结尾(指定范围):',str1.endswith(str2,10,19))
#检测 str2是否包含在字符串str1中,如果指定范围 beg 和 end ,则检查是否包含在指定范围内,
#如果包含返回开始的索引值,否则返回-1
print('str1中是否包含str2:',str1.find(str2))
print('str1中是否包含str2(指定范围):',str1.find(str2,10,20))
执行结果:
str1的长度: 20
str1以空格分割的结果: ['', '', 'Hello', 'world!', 'Hello']
str1删除首尾空格: Hello world! Hello
str2在str1中出现的次数: 2
str2在str1中出现的次数(指定范围): 1
str1是否以 str2结尾: True
str1是否以 str2结尾(指定范围): False
str1中是否包含str2: 2
str1中是否包含str2(指定范围): 15
2.列表
列表是 Python 最基本,也是最重要的一种数据结构。列表用方括号 []
定义,方括号括起的部分就是一个列表。Python 为列表提供了很多非常有用的内建方法,使用起来非常简单。
创建列表
#随意创建3个列表,同一个列表中可以存放任意基本数据类型
list1 = [3.14, 5.96, 1897, 2020, "China",3+4j];
list2 = [1, 2, 3, 4, 5 ];
list3 = ["BeiJing", "ShangHai", "NanJing", "GuangZhou"];
list4 = []
如上所示,列表中的数据项可以是不同的数据类型,如 list1,同时包含浮点型、整型、长整型、字符串和复数;也可以创建一个空列表,如 list4。
访问列表中的元素
通过索引下标访问列表中的元素,可以一次访问一个或多个元素。
#通过索引下标访问列表中的单个元素
print("list1[2]: ",list1[2])
print("list2[2]: ",list2[2])
print("list3[2]: ",list3[2])
#通过索引下标一次访问多个元素
print("list1[0:2]:",list1[0:2])
执行结果:
list1[2]: 1897
list2[2]: 3
list3[2]: NanJing
list1[0:2]: [3.14, 5.96]
更改列表中的元素
list1 = [3.14, 5.96, 1897, 2020, "China",3+4j];
print("before list1[3]: ",list1[3])
list1[3] = "Change"
print("after list1[3]: ",list1[3])
执行结果:
before list1[3]: 2020
after list1[3]: Change
删除列表中的元素
Python 提供三种删除列表中元素的方法:del、remove(obj)、clear(),分别用于删除列表中的元素和清空列表。如下例子:
list1 = [3.14, 5.96, 1897, 2020, "China",3+4j];
print("Before the operation:",list1)
del list1[3]
list1.remove(3+4j)
print("After deleting the element:",list1)
list1.clear()
print("After the emptying of the list:",list1)
执行结果:
Before the operation: [3.14, 5.96, 1897, 2020, 'China', (3+4j)]
After deleting the element: [3.14, 5.96, 1897, 'China']
After the emptying of the list: []
向列表中添加新元素
有两个内建方法可以用于添加新元素:
- append(object):添加新元素将新元素添加到列表末尾;
- insert(index,object):插入新元素到指定索引位置。
如下例子:
list1 = [3.14, 5.96, 1897, 2020, "China",3+4j];
print("before list1: ",list1)
list1.append("New Ele-I")
list1.insert(2, "New Ele-II")
print("after list1: ",list1)
执行结果:
before list1: [3.14, 5.96, 1897, 2020, 'China', (3+4j)]
after list1: [3.14, 5.96, 'New Ele-II', 1897, 2020, 'China', (3+4j), 'New Ele-I']
列表常用内建方法
上面是关于列表的“增、删、改、查”基本操作,除此之外,Python 列表还有很多内建函数和有趣的操作。
list1 = [2012, 1949, 1897, 2050, 1945, 1949];
#求列表长度,即列表中元素的个数:len(obj)
print("The length of the list: ", len(list1))
#求列表中元素的最大值:max(list)
print("The largest element in the list: ", max(list1))
#求列表中元素的最小值:min(list)
print("The smallest element in the list: ", min(list1))
#统计某个元素在列表中出现的次数:list.count(obj)
print("The number of times 1949 appears: ", list1.count(1949))
#从列表中找出某个值第一个匹配项的索引位置:list.index(obj)
print("The index of the first location of 1949: ", list1.index(1949))
#复制一个已有的表:list.copy()
list2 = list1.copy()
print("list2:", list2)
#反转一个已有的表:list.reverse()
list1.reverse()
print("After reversing :", list1)
执行结果:
The length of the list: 6
The largest element in the list: 2050
The smallest element in the list: 1897
The number of times 1949 appears: 2
The index of the first location of 1949: 1
list2: [2012, 1949, 1897, 2050, 1945, 1949]
After reversing : [1949, 1945, 2050, 1897, 1949, 2012]
列表常用操作
为了高效的使用列表,Python 支持一些常用的操作:列表拼接、列表乘法、迭代、嵌套等。
list1 = [1,2,3,4]
list2 = [5,6,"BeiJin",7,8]
#列表拼接
print("list1 + list2:",list1 + list2)
#列表乘法
print("list1*2:",list1*2)
#判断元素是否存在于列表中
print("3 in list1?",3 in list1)
print("100 in list1?",100 in list1)
#迭代
for element in list1:
print(element)
#列表嵌套
list3 = [list1, list2]
print("list3:", list3)
执行结果:
list1 + list2: [1, 2, 3, 4, 5, 6, 'BeiJin', 7, 8]
list1*2: [1, 2, 3, 4, 1, 2, 3, 4]
3 in list1? True
100 in list1? False
1
2
3
4
list3: [[1, 2, 3, 4], [5, 6, 'BeiJin', 7, 8]]
3.栈
在上面“列表”小节中,讲到了列表有两个内建函数:
- append(obj):添加元素到列表尾部;
- pop():从列表尾部取出元素。
不难理解,通过 append(obj) 函数最后添加的元素,可以通过 pop() 函数最先取出来,也就是“后进先出”,这种数据结构称为“栈”。利用列表的两个内建方法,很容易实现“栈”这种数据结构。
如下例子:
#初始化一个列表
stack1 = [1, 2, 3, 4, 5 ]
#向表尾添加元素
stack1.append(123)
print("stack1:",stack1)
#从表尾取出元素
print("stack1.pop():",stack1.pop())
执行结果:
('stack1:', [1, 2, 3, 4, 5, 123])
('stack1.pop():', 123)
4.元组
元组是与列表类似的一种数据结构。Python 的元组与列表有很多类似的地方,但区别也是很明显的:
- 定义方式不一样:列表采用方括号
[]
,元组采用圆括号()
; - 元组中的元素不能改变,元组一旦创建就不能再对其中的元素进行增、删、改,只能访问。
创建元组
#创建3个元组,和列表类似,同一个元组中可以存放任意数据类型
tuple1 = ()
tuple2 = (12,)
tuple3 = (1, 2, 3, 4, 5)
tuple4 = (3.14, 5.96, 1897, 2020, "China",3+4j)
tuple5 = 4,5,6,7
如上所示,元组和列表类似,同一个元组中可以没有数据,也可以有多种数据,非常灵活。
不过需要注意:
- 如果元组只有一个元素,那么这个元素后面要加逗号,如 tuple2,否则将被认为是一个基本数据类型,而不是元组;
- 创建元组,可以没有括号,元素之间用逗号分隔开即可。
访问元组中的元素
元组和列表都是一种序列,因此,也可以通过索引来访问,方法是一样的,如下例子:
tuple1 = (3.14, 5.96, 1897, 2020, "China",3+4j)
print("tuple1[2]:",tuple1[2])
print("tuple1[1:3]:",tuple1[1:3])
执行结果:
tuple1[2]: 1897
tuple1[1:3]: (5.96, 1897)
元组相关的常用内建方法
tuple1 = (3.14, 5.96, 1897, 2020, 1949)
#求元组长度,即元组中元素的个数:len(tuple)
print("The length of the tuple: ", len(tuple1))
#求元组中元素的最大值:max(tuple)
print("The largest element in the tuple: ", max(tuple1))
#求元组中元素的最小值:min(tuple)
print("The smallest element in the tuple: ", min(tuple1))
#统计某个元素在元组中出现的次数:tuple.count(obj)
print("The number of times 1949 appears: ", tuple1.count(1949))
#从元组中找出某个值第一个匹配项的索引位置:tuple.index(obj)
print("The index of the first location of 1949: ", tuple1.index(1949))
执行结果:
The length of the tuple: 5
The largest element in the tuple: 2020
The smallest element in the tuple: 3.14
The number of times 1949 appears: 1
The index of the first location of 1949: 4
元组常用的操作
元组常用的运算操作与列表相同,包括:元组拼接、元组乘法、迭代、嵌套等。简要举例如下:
tuple1 = (1,2,3,4)
tuple2 = (5,6,"BeiJin",7,8)
#元组拼接
print("tuple1 + tuple2:",tuple1 + tuple2)
#元组乘法
print("tuple1*2:",tuple1*2)
#判断元素是否存在于元组中
print("3 in tuple1?",3 in tuple1)
print("100 in tuple1?",100 in tuple1)
#迭代
for element in tuple1:
print(element)
#元组嵌套
tuple3 = [tuple1, tuple2]
print("tuple3:", tuple3)
执行结果:
tuple1 + tuple2: (1, 2, 3, 4, 5, 6, 'BeiJin', 7, 8)
tuple1*2: (1, 2, 3, 4, 1, 2, 3, 4)
3 in tuple1? True
100 in tuple1? False
1
2
3
4
tuple3: [(1, 2, 3, 4), (5, 6, 'BeiJin', 7, 8)]
5.字典
Python 的字典可以理解为一种映射表,存储 key-value(键值对)类型数据的容器。关于字典有三点需要注意:
- 同一个字典中,键必须是唯一的,不存在两个相同的键,键的值不能改变,数据类型可以是数字,字符串或者元组;
- 同一个字典中,值不必唯一,值可以是任意数据类型;
- 字典定义采用花括号 {},键值之间用冒号隔开,键值对之间用逗号隔开;
创建字典
#创建几个字典
dict1 = {"ID0012":"ZhangSan", "ID0019":"LiSi", "ID0015":"WangWu"}
dict2 = {1:"BeiJin",3:"ShangHai",5:"HangZhou"}
dict3 = {"Excellent":100, "Good":80, "Pass":60, "Fail":50}
#字典也可以嵌套
dict4 = {"A":["LiTong","XiaoMing"], "B":["XiaoHong","XiaoHua"]}
访问字典中的元素
与列表和元组不同,访问字典中的元素不能通过索引,字典中键和值是成对的,而键是唯一的,那么,通过键就可以访问对应的值。
访问有两种方式:
- 直接通过键来访问;
- 通过内建 get(key) 方法访问。
如下例子:
dict1 = {"Excellent":100, "Good":80, "Pass":60, "Fail":50}
dict2 = {1:"BeiJin",3:"ShangHai",5:"HangZhou"}
print("dict1['Good']:", dict1['Good'])
print("dict2[1]:", dict2[1])
print("dict1.get('Good'):", dict1.get('Good'))
执行结果:
dict1['Good']: 80
dict2[1]: BeiJin
dict1.get('Good'): 80
修改字典中的元素
dict1 = {"Excellent":100, "Good":80, "Pass":60, "Fail":50}
dict1['Good'] = 85
print("dict1['Good']:", dict1.get('Good'))
执行结果:
dict1['Good']: 85
删除字典中的元素或字典
和列表一样,可以通过 del 语句删除字典中的元素或者删除整个字典,可以通过内建方法 clear() 清空字典,还可以通过内建方法 pop(key) 删除指定的元素,如下例子:
dict1 = {"Excellent":100, "Good":80, "Pass":60, "Fail":50}
#删除字典中的一个元素
del dict1['Good']
print("dict1:", dict1)
#使用内建方法pop(key)删除指定元素
dict1.pop('Pass')
print("dict1:", dict1)
#清空字典
dict1.clear()
print("dict1:", dict1)
#删除字典
del dict1
执行结果:
dict1: {'Excellent': 100, 'Pass': 60, 'Fail': 50}
dict1: {'Excellent': 100, 'Fail': 50}
dict1: {}
字典的常用内建方法
dict1 = {"Excellent":100, "Good":80, "Pass":60, "Fail":50}
#计算字典的长度
print("The length of the dict1: ", len(dict1))
#获取字典中所有的键
print("Get all the keys in dict1:\n", dict1.keys())
#获取字典中所有的值
print("Get all the values in dict1:\n", dict1.values())
#获取字典中所有的键值对
print("Get all the key-value pairs in dict1:\n", dict1.items())
执行结果:
The length of the dict1: 4
Get all the keys in dict1:
dict_keys(['Excellent', 'Good', 'Pass', 'Fail'])
Get all the values in dict1:
dict_values([100, 80, 60, 50])
Get all the key-value pairs in dict1:
dict_items([('Excellent', 100), ('Good', 80), ('Pass', 60), ('Fail', 50)])
6.集合
集合(set)是一个数学概念,是由一个或多个确定的元素所构成的整体。
集合具有三个特点:
- 确定性,集合中的元素必须是确定的;
- 互异性,集合中的元素互不相同;
- 无序性,集合中的元素没有先后之分。
根据集合的特点,Python 中集合的基本功能包括关系测试和消除重复元素。
创建集合
可以用大括号({})创建集合。但需要注意,如果要创建一个空集合,必须用 set() 而不是 {} ;后者创建一个空的字典。
country1 = {'China', 'America', 'German'}
country2 = set('China')
country3 = set()
print("country1:",country1)
print("country2:",country2)
print("country3:",country3)
执行结果:
('country1:', set(['German', 'America', 'China']))
('country2:', set(['i', 'h', 'C', 'a', 'n']))
('country3:', set([]))
操作集合中的元素
我们可以访问集合中的元素,也可增加,移除元素。
setA = {'A', 'B', 'C'}
print("setA:",setA)
setA.add('D')
print("setA.add('D'):",setA)
setA.add('A')
print("setA.add('A'):",setA)
setA.remove('A')
print("setA.remove('A'):",setA)
执行结果:
('setA:', set(['A', 'C', 'B']))
("setA.add('D'):", set(['A', 'C', 'B', 'D']))
("setA.add('A'):", set(['A', 'C', 'B', 'D']))
("setA.remove('A'):", set(['C', 'B', 'D']))
集合运算
对于集合,我们可以计算交集、并集、补集。
setA = {'A', 'B', 'C'}
setB = {'D', 'C', 'E'}
#交集
print("setA & setB:",setA&setB)
#并集
print("setA | setB:",setA|setB)
#差集
print("setA - setB:",setA-setB)
执行结果:
('setA & setB:', set(['C']))
('setA | setB:', set(['A', 'C', 'B', 'E', 'D']))
('setA - setB:', set(['A', 'B']))
7.队列
前面讲到可以直接将列表作为栈使用。Python 中有专门的队列模块 Queue,本节介绍一种用列表实现的队列,如下例子:
from collections import deque
#基于列表初始化一个队列
queue = deque(['A','B','C'])
#队尾添加元素
queue.append('D')
print('queue',queue)
#队头出列
queue.popleft()
print('queue',queue)
#队头出列
queue.popleft()
print('queue',queue)
执行结果:
('queue', deque(['A', 'B', 'C', 'D']))
('queue', deque(['B', 'C', 'D']))
('queue', deque(['C', 'D']))