- jdbc持久化配置及使用journal的方式见activemq,配置完成后便可以实现jdbc数据库持久化.
- zookeeper+activemq集群配置
- 需要导入的jar包
<dependency>
<groupId>org.apache.activemq</groupId>
<artifactId>activemq-all</artifactId>
<version>5.15.9</version>
</dependency>
<dependency>
<groupId>org.apache.xbean</groupId>
<artifactId>xbean-spring</artifactId>
<version>3.16</version>
</dependency>
- 使用queue消息生产者编码
package com.atguigu.activemq.queue;
import lombok.extern.log4j.Log4j;
import org.apache.activemq.ActiveMQConnectionFactory;
import javax.jms.*;
@Log4j
public class JmsProduce {
private static final String ACTIVEMQ_URL = "failover:(tcp://192.168.145.3:61616,tcp://192.168.145.3:61617,tcp://192.168.145.3:61618)?randomize=false";
private static final String QUEUE_NAME = "queue-cluster";
public static void main(String[] args) throws JMSException {
ActiveMQConnectionFactory activeMQConnectionFactory = new ActiveMQConnectionFactory(ACTIVEMQ_URL);
Connection connection = activeMQConnectionFactory.createConnection();
connection.start();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = session.createQueue(QUEUE_NAME);
MessageProducer messageProducer = session.createProducer(queue);
for(int i = 1; i <= 3; i++){
TextMessage textMessage = session.createTextMessage("cluster msg---" + i);
messageProducer.send(textMessage);
}
messageProducer.close();
session.close();
connection.close();
log.info("******消息发送到MQ完成");
}
}
- 使用queue消息消费者编码
package com.atguigu.activemq.queue;
import lombok.extern.slf4j.Slf4j;
import org.apache.activemq.ActiveMQConnectionFactory;
import javax.jms.*;
import java.io.IOException;
@Slf4j
public class JmsConsumer {
private static final String ACTIVEMQ_URL = "failover:(tcp://192.168.145.3:61616,tcp://192.168.145.3:61617,tcp://192.168.145.3:61618)?randomize=false";
private static final String QUEUE_NAME = "queue-cluster";
public static void main(String[] args) throws JMSException, IOException {
ActiveMQConnectionFactory activeMQConnectionFactory = new ActiveMQConnectionFactory(ACTIVEMQ_URL);
Connection connection = activeMQConnectionFactory.createConnection();
connection.start();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = session.createQueue(QUEUE_NAME);
MessageConsumer messageConsumer = session.createConsumer(queue);
messageConsumer.setMessageListener(new MessageListener() {
@Override
public void onMessage(Message message) {
if(message instanceof TextMessage){
TextMessage textMessage = (TextMessage)message;
try {
log.info(textMessage.getText());
} catch (JMSException e) {
e.printStackTrace();
}
}
}
});
System.in.read();
messageConsumer.close();
session.close();
connection.close();
}
}
- 使用topic消息生产者编码(单机/集群+非持久化)
package com.atguigu.activemq.topic;
import lombok.extern.slf4j.Slf4j;
import org.apache.activemq.ActiveMQConnectionFactory;
import javax.jms.*;
@Slf4j
public class JmsProducer_topic {
private static final String ACTIVEMQ_URL = "failover:(tcp://192.168.145.3:61616,tcp://192.168.145.3:61617,tcp://192.168.145.3:61618)?randomize=false";
private static final String TOPIC_NAME = "topic-cluster";
public static void main(String[] args) throws JMSException {
ActiveMQConnectionFactory activeMQConnectionFactory = new ActiveMQConnectionFactory(ACTIVEMQ_URL);
Connection connection = activeMQConnectionFactory.createConnection();
connection.start();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Topic topic = session.createTopic(TOPIC_NAME);
MessageProducer messageProducer = session.createProducer(topic);
for(int i=1; i <= 6; i++){
TextMessage textMessage = session.createTextMessage("TOPIC MESSAGE---" + i);
messageProducer.send(textMessage);
}
messageProducer.close();
session.close();
connection.close();
log.info("******topic_name消息发布到mq完成");
}
}
- 使用topic消息消费者编码(单机/集群+非持久化)
package com.atguigu.activemq.topic;
import lombok.extern.slf4j.Slf4j;
import org.apache.activemq.ActiveMQConnectionFactory;
import javax.jms.*;
import java.io.IOException;
@Slf4j
public class JmsConsumer_topic {
private static final String ACTIVEMQ_URL = "failover:(tcp://192.168.145.3:61616,tcp://192.168.145.3:61617,tcp://192.168.145.3:61618)?randomize=false";
private static final String TOPIC_NAME = "topic-cluster";
public static void main(String[] args) throws JMSException, IOException {
log.info("3号消费者");
ActiveMQConnectionFactory activeMQConnectionFactory = new ActiveMQConnectionFactory(ACTIVEMQ_URL);
Connection connection = activeMQConnectionFactory.createConnection();
connection.start();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Topic topic = session.createTopic(TOPIC_NAME);
MessageConsumer consumer = session.createConsumer(topic);
consumer.setMessageListener(new MessageListener() {
@Override
public void onMessage(Message message) {
if(message instanceof TextMessage){
TextMessage textMessage = (TextMessage)message;
try {
log.info("*******消费者接收到消息:" + textMessage.getText());
} catch (JMSException e) {
e.printStackTrace();
}
}
}
});
System.in.read();
consumer.close();
session.close();
connection.close();
}
}
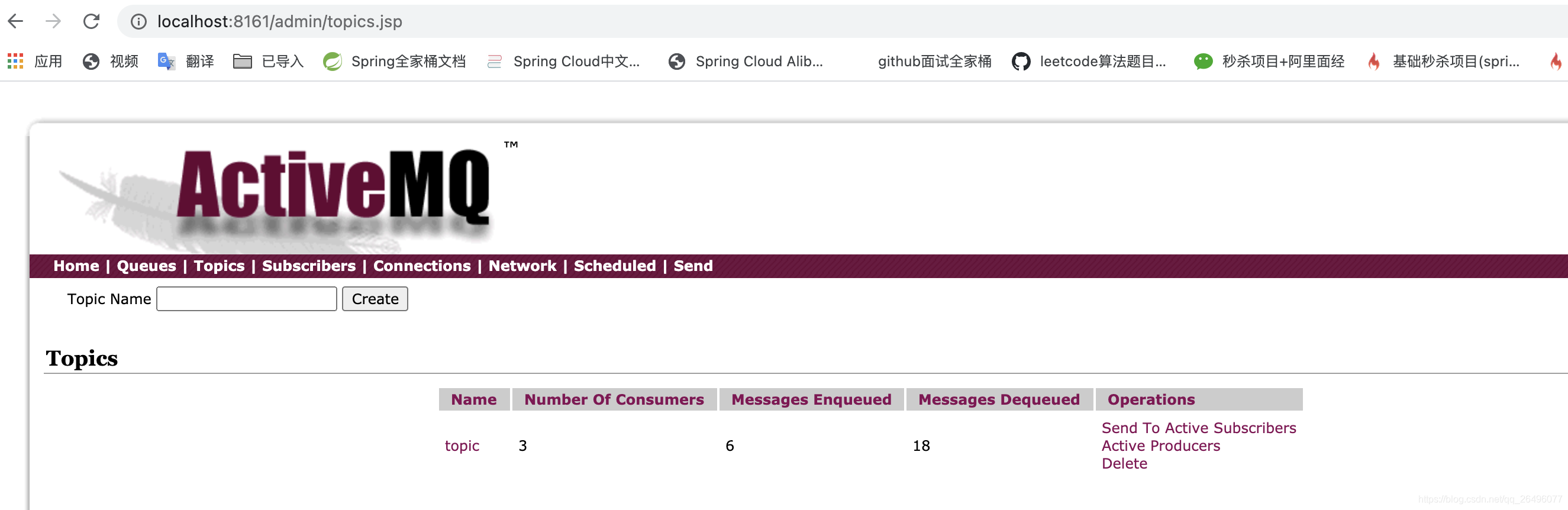
- 使用topic消息生产者编码(单机/集群+持久化)
package com.atguigu.activemq.topic;
import lombok.extern.slf4j.Slf4j;
import org.apache.activemq.ActiveMQConnectionFactory;
import javax.jms.*;
@Slf4j
public class JmsProducer_topic_persist {
private static final String ACTIVEMQ_URL = "failover:(tcp://192.168.145.3:61616,tcp://192.168.145.3:61617,tcp://192.168.145.3:61618)?randomize=false";
private static final String TOPIC_NAME = "topic-cluster";
public static void main(String[] args) throws JMSException {
ActiveMQConnectionFactory activeMQConnectionFactory = new ActiveMQConnectionFactory(ACTIVEMQ_URL);
Connection connection = activeMQConnectionFactory.createConnection();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Topic topic = session.createTopic(TOPIC_NAME);
MessageProducer messageProducer = session.createProducer(topic);
messageProducer.setDeliveryMode(DeliveryMode.PERSISTENT);
connection.start();
for(int i=1; i <= 6; i++){
TextMessage textMessage = session.createTextMessage("TOPIC MESSAGE---" + i);
messageProducer.send(textMessage);
}
messageProducer.close();
session.close();
connection.close();
log.info("******topic_name消息发布到mq完成");
}
}
- 使用topic消息消费者编码(单机/集群+持久化)
package com.atguigu.activemq.topic;
import lombok.extern.slf4j.Slf4j;
import org.apache.activemq.ActiveMQConnectionFactory;
import javax.jms.*;
import java.io.IOException;
@Slf4j
public class JmsConsumer_topic_persist {
private static final String ACTIVEMQ_URL = "failover:(tcp://192.168.145.3:61616,tcp://192.168.145.3:61617,tcp://192.168.145.3:61618)?randomize=false";
private static final String TOPIC_NAME = "topic-cluster";
public static void main(String[] args) throws JMSException, IOException {
log.info("******持久化z3");
ActiveMQConnectionFactory activeMQConnectionFactory = new ActiveMQConnectionFactory(ACTIVEMQ_URL);
Connection connection = activeMQConnectionFactory.createConnection();
connection.setClientID("z3");
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Topic topic = session.createTopic(TOPIC_NAME);
TopicSubscriber topicSubscriber = session.createDurableSubscriber(topic, "remark...");
connection.start();
Message message = topicSubscriber.receive();
while(message != null){
TextMessage textMessage = (TextMessage)message;
log.info("******收到的持久化topic: " + textMessage.getText());
message = topicSubscriber.receive(5000L);
}
session.close();
connection.close();
}
}
- 使用topic持久化,一定要先运行一次消费者,相当于订阅,然后再运行生产者发送消息,这时不论消费者是否在线都会接收到,不在线的话,下次连接的时候会把没有收到的消息都接收下来.