1218. Longest definite difference subsequence
1218. Longest definite difference subsequence
Topic description:
Give you an integer array arr
and an integer difference
, please find and return the longest one in arr
The length of an arithmetic subsequence in which the difference between adjacent elements is equal to difference
.
Subsequence is derived from arr
by deleting some elements or not deleting any elements without changing the order of the remaining elements. sequence
Example 1:
Input:arr = [1,2,3,4], difference = 1
Output: 4
Explanation: The longest arithmetic subsequence is [1,2,3,4].
Example 2:
Input:arr = [1,3,5,7], difference = 1
Output: 1
Explanation: The longest arithmetic subsequence is any single element.
Example 3:
Input:arr = [1,5,7,8,5,3,4,2,1], difference = -2
Output: 4
Explanation: The longest arithmetic subsequence is [7,5,3,1].
hint:
1 <= arr.length <= 105
-104 <= arr[i], difference <= 104
Problem-solving ideas:
Algorithm idea:
This question is somewhat similar to
300. Longest Increasing Subsequence
, but if you read the question carefully, you will find that this question < /span>
Gauda
arr.lenght
10^5
, use
O(N^2)
< a i=4>lcs
Model meeting super time.
So, what information does it have about
300. The longest increasing subsequence
? It's a fixed difference. Before, we only knew to increment, not before
What should a number be? Now that we can calculate what the previous number is, we can use numerical values to define it
dp
array of
value and form a state transition. In this way, existing information is effectively used.
1.
Status display:
dp[i]
means: all subsequences ending with the element at position
i
, the length of the longest arithmetic subsequence.
2.
State transfer process:
For
dp[i]
, the value of the previous definite difference subsequence is set to
arr[i] - difference
. Just find one of the above numbers
The word is the length of the final difference sequence
dp[arr[i] - difference]
, then add
is the knoti , that is,
1
The length of the tail sequence of fixed differences.
Therefore, you can choose to use a hash table for optimization. We can bind "element,
dp[j]
" and put it into the hash table. Very
There is no need to create or
dp
arrays, and do dynamic programming directly in the hash table.
3.
Initialization:
At the beginning, you need to put the first element into the hash table,
hash[arr[0]] = 1
.
4.
Filling order:
According to the "State Transition Equation", the order of filling out the form should be "from left to right".
5.
Reply:
According to the "status representation", return the maximum value in the entire
dp
table
Solution code:
class Solution
{
public:
int longestSubsequence(vector<int>& arr, int difference)
{
// 创建⼀个哈希表
unordered_map<int, int> hash; // {arr[i], dp[i]}
hash[arr[0]] = 1; // 初始化
int ret = 1;
for(int i = 1; i < arr.size(); i++)
{
hash[arr[i]] = hash[arr[i] - difference] + 1;
ret = max(ret, hash[arr[i]]);
}
return ret;
}
};
873. The length of the longest Fibonacci subsequence
873. The length of the longest Fibonacci subsequence
Topic description:
If the sequence X_1, X_2, ..., X_n
satisfies the following conditions, it is said to be Fibonacci :< /span>
n >= 3
- For all
i + 2 <= n
, there is X_i + X_{i+1} = X_{i+2}
Given astrictly increasing array of positive integers forming the sequence arr , find arrThe length of the longest Fibonacci subsequence in . If one does not exist, return 0 .
(Recall that the subsequence is derived from the original sequence arr which is derived from Delete any number of elements (or none) in arr without changing the order of the remaining elements. For example, [3, 5, 8]
is [3, 4, 5, 6, 7, 8]
a subsequence)
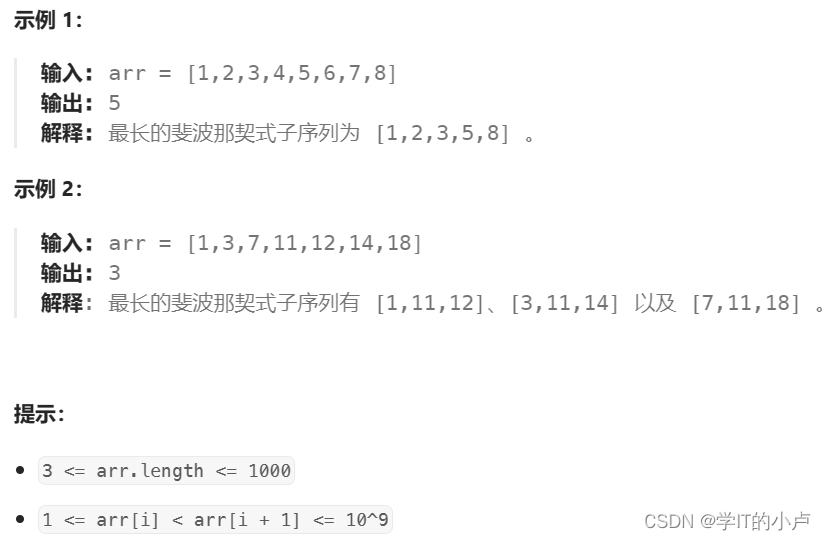
Problem-solving ideas:
Algorithm idea:
1.
Status display:
For linear
dp
, we can use "experience + problem requirements" to define the state representation:
i.
End with a certain position, blah blah;
ii.
Start from a certain location, blah blah.
Here we choose a more commonly used method, ending with a certain position and combining the requirements of the topic to define a status representation:
dp[i]
means: "all subsequences ending with the element at
i
”, the length of the longest Fibonacci subsequence.
But there is a very fatal problem here, that is, we cannot determine what the Fibonacci sequence at the end of i looks like. This will lead to
As a result, we cannot derive the state transition equation, so the state representation we define needs to be able to determine a Fibonacci sequence.
According to the characteristics of the Fibonacci sequence, we only need to know the last two elements in the sequence to determine what the sequence looks like.
Therefore, we modify our status representation as:
dp[i][j]
means:
i
position and
j The element at position
is the longest Fibonacci subsequence among all the subsequences at the end
Ordinal length. Determine below
i < j
.
2.
State transfer process:
Suppose
nums[i] = b, nums[j] = c
, then the first element of this sequence is
. Our roots
a = c - b
a
's information:
i.
a
Existence, lower post
k
,
a < Since then
i positional element
The length of the longest Fibonacci subsequence, and then add the elements at the
j
position. So
dp[i][j] =
dp[k][i] + 1
;
ii.
a
existence, but is
b < a < c < a i=4>: At this time, the two elements are playing themselves,
dp[i][j] = 2 ;
iii.
a
Non-existence: At this time, both the elements are self-playing,
dp[i][j] = 2
.
In summary, the state transfer process can be discussed on a case-by-case basis.
Optimization point: We found that in the state transition process, we need to determine the subscript of the
a
element. Therefore we can
dp
Before, bind all "elements + subscripts" together and put them into the hash table.
3.
Initialization:
can initialize all values in the table to
2
.
4.
Filling order:
a.
Fix the last number first;
b.
Then enumerate the second to last number.
5.
Reply:
Because we don’t know who the final result ends with, we return the maximum value in the
dp
table
ret
.
However,
ret
possible
3
, 小于
3
's explanation does not exist.
So it needs to be judged.
Solution code:
class Solution {
public:
int lenLongestFibSubseq(vector<int>& arr) {
int n=arr.size();
unordered_map<int,int>hash;
vector<vector<int>>dp(n,vector<int>(n,2));
for(int i=0;i<n;i++)
hash[arr[i]]=i;
int ret=2;
for(int j=2;j<n;j++)
{
for(int i=1;i<j;i++)
{
int a=arr[j]-arr[i];
if(a<arr[i]&&hash.count(a))
dp[i][j]=dp[hash[a]][i]+1;
ret=max(ret,dp[i][j]);
}
}
return ret<3?0:ret;
}
};