http://acm.hdu.edu.cn/showproblem.php?pid=2007
#include<iostream> #include<cstdio> using namespace std; int main() { int m,n; while(cin>>m>>n) { long long sum_odd=0,sum_even=0; if(m>n)//题干中没说m一定小于n; swap(m,n); while(m<=n) { if(m%2==0) sum_even+=m*m; else sum_odd+=m*m*m; m++; } printf("%lld %lld\n",sum_even,sum_odd); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2013
#include<iostream> #include<cstdio> using namespace std; long long record[40];//整数型结果用long long,防止数值越界,养成习惯; int n; void sum(int x) { if(x==-1) return ; record[x]=2*(record[x+1]+1); sum(x-1); } int main() { int n; while(cin>>n) { record[n]=1; sum(n-1); printf("%lld\n",record[1]); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2015
//一开始把题干理解错了,“如果最后不足m个,则以实际数量求平均值。”,这句话的意思是,求剩余不足m个数的平均值 //假设n=5,m=3 {1,2,3,4,5} 前三个数满足条件,最后剩余两个数,输出(4+5)/(n%m)即(4+5)/2; #include<iostream> #include<cstdio> using namespace std; int record[110]; int main() { int n,m; long long average; while(~scanf("%d%d",&n,&m)) { int even_number=2; for(int i=1;i<=n;i++,even_number+=2) record[i]=even_number; bool flag=0; int sum=n/m; int i=1; while(sum--) { int k=m; average=0; while(k--) average+=record[i++]; if(flag==0) { printf("%lld",average/m); flag=1; } else printf(" %lld",average/m); } if(n%m!=0) { average=0; int T=n%m; int i=n; while(T--) average+=record[i--]; printf(" %lld",average/(n%m)); } printf("\n"); //注意输出格式,每行数最后一个数据后无空格; } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2024
//合法的标识符,由字母数字下划线组成,第一个字符不能是数字 #include<iostream> #include<cstdio> #include<cstring> #include<algorithm> using namespace std; char words[50]; bool flag; void is_legal() { int len=strlen(words); if(!isalpha(words[0])&&words[0]!='_') { flag=false; return ; } for(int i=1;i<len;i++) { if(isalpha(words[i])) continue; if(words[i]=='_') continue; if(words[i]<='9'&&words[i]>='0') continue; flag=false; return ; } flag=true; } int main() { int n; cin>>n; getchar(); while(n--) { gets(words); is_legal(); if(flag) printf("yes\n"); else printf("no\n"); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2026
#include<iostream> #include<cstdio> #include<cstring> using namespace std; int main() { char words[110]; while(gets(words)) { int len=strlen(words); words[0]=toupper(words[0]); for(int i=1;i<len;i++) { if(words[i-1]==' ') words[i]=toupper(words[i]);//(tolower/toupper)实现字母的大小写转换 } printf("%s\n",words); } return 0; }
tolower,toupper函数,借鉴大神博客
https://blog.csdn.net/laozhuxinlu/article/details/51539737
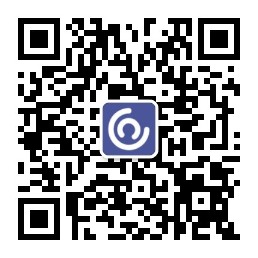
http://acm.hdu.edu.cn/showproblem.php?pid=2030
/*每个汉字机内码使用二个字节,每个字节最高位一位为 1 。计算机中,补码第一位是符号位, 1 表示为 负数,所以,汉字机内码的每个字节 表示的十进制数都是负数,统计输入字符串含有几个汉字,只只需求出字符串中小于 0 的字符有几个,将它除以 2 就是汉字的个数。*/ #include<iostream> #include<cstdio> #include<cstring> using namespace std; int main() { string words; int n; cin>>n; getchar(); while(n--) { getline(cin,words); long long sum=0; for(int i=0;i<words.length();i++) if((int)words[i]<0) sum++; printf("%d\n",sum/2); } return 0; }
其他答案
http://www.cnblogs.com/Lvsi/archive/2011/01/30/1947893.html
http://acm.hdu.edu.cn/showproblem.php?pid=2031
#include<iostream> #include<cstdio> #include<cstdlib> #include<cstring> using namespace std; char number[10000]; void conversion(long long N,int R) { int num=abs(N); int flag=0; while(num) { int x=num%R; if(x<=9) number[flag++]=x+'0'; else { if(x==10) number[flag++]='A'; else if(x==11) number[flag++]='B'; else if(x==12) number[flag++]='C'; else if(x==13) number[flag++]='D'; else if(x==14) number[flag++]='E'; else if(x==15) number[flag++]='F'; } num/=R; } number[flag]='\0'; } int main() { long long N; int R; while(~scanf("%lld%d",&N,&R)) { long long consequence; conversion(N,R); if(N<0) { printf("-"); for(int i=strlen(number)-1;i>=0;i--) printf("%c",number[i]); } else { for(int i=strlen(number)-1;i>=0;i--) printf("%c",number[i]); } printf("\n"); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2034
#include<iostream> #include<cstdio> #include<algorithm> using namespace std; int main() { int n,m; while(~scanf("%d%d",&n,&m)) { if(n==0&&m==0) break; int A[110],B[110]; for(int i=1;i<=n;i++) cin>>A[i]; A[n+1]='\0'; for(int i=1;i<=m;i++) cin>>B[i]; B[m+1]='\0'; sort(A+1,A+n+1);//排序很重要,A,B数组都按从小到大的顺序排列; sort(B+1,B+m+1); int *p_A=&A[1],*p_B=&B[1]; bool flag=1; while(*p_A!='\0')//时间复杂度为O(n+m) { if(*p_B=='\0') { flag=0; printf("%d ",*p_A); p_A++; continue; } if(*p_A==*p_B)//当p_A指针与p_B指针所指向的数值相等时,不打印*p_A,p_A与p_B都往下一个地址移; { p_A++; p_B++; continue; } if(*p_A<*p_B) { flag=0; printf("%d ",*p_A);//当p_A指针指向的数值大于p_B指针指向的数值时,打印*p_A,并且p_A往下一个地址移; p_A++; } else p_B++; } if(flag) printf("NULL"); printf("\n"); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2035
#include<iostream> #include<cstdio> using namespace std; long long quick_power(long long x,long long n,long long mod) { long long ans=1; while(n>0) { if(n&1) ans=(ans*x)%mod; x=(x*x)%mod; n/=2; } return ans; } int main() { long long x,n; while(~scanf("%lld%lld",&x,&n)) { if(x==0&&n==0) break; int mod=1000; long long ans=quick_power(x,n,mod); printf("%lld\n",ans); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2036
n边形可以拆分成(n-2)个三角形;假设三角形的三个顶点为A,B,C,(设(向量)AB=(x1,y1),(向量)AC=(x2,y2));
根据向量叉乘公式S=(向量)AB*(向量)AC,即S=(x1*y2-x2*y1);
把n边形拆分成(n-1)个三角形后用向量积求出面积即可;
#include<iostream> #include<cstdio> #include<cmath> using namespace std; struct { long long x,y; }angle[110]; int main() { int n; while(cin>>n) { if(n==0) break; for(int i=1;i<=n;i++) scanf("%lld%lld",&angle[i].x,&angle[i].y); int k=2; double ans=0; while(k!=n) { double x1=angle[k].x-angle[1].x; double y1=angle[k].y-angle[1].y; double x2=angle[k+1].x-angle[1].x; double y2=angle[k+1].y-angle[1].y; ans+=(x1*y2-x2*y1);//错误提交:ans+=fabs((x1*y2-x2*y1)); k++; } printf("%.1f\n",ans/2); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2047
一、题目分析
这是一道递推的题目。分析一下:
由于长度是n的字符串只有’E’、’O’、’F’组成,并且不能有两个’O’相连,所以我们假设一共有M(n)种排列的可能。而这M(n)中可能又可以分为一下两种:
1)最后一个字符是‘O’:
对于这种情况,很显然第n-1个位置不能是’O’,否则会出现两个’O’相邻的情况,所以此时前面n-1个位置有2*M(n-2)种可能。而第n个位置此时是’O’,所以此时共有2* M(n-2)种可能;
2)最后一个字符不是 ‘O’:
对于这种情况,那么对前面n-1个位置没有特殊的要求,所以对于前面n-1个位置有M(n-1)种可能,而第n个位置有两种可能,所以此时共有2*M(n-1)种可能
综上可知,本题的递推关系为:M(n)=2*[M(n-1)+M(n-2)]
#include<iostream> #include<cstdio> using namespace std; long long a[41]; int main() { int n; a[1]=3; a[2]=8; for(int i=3;i<=40;i++) a[i]=2*(a[i-1]+a[i-2]); while(cin>>n) printf("%lld\n",a[n]); return 0; }
参照大牛博客
http://acm.hdu.edu.cn/showproblem.php?pid=2096
#include<iostream>
#include<cstdio>
using namespace std;
int main()
{
int A,B;
int T;
cin>>T;
while(T--)
{
cin>>A>>B;
cout<<(A%100+B%100)%100<<endl;//注意int型,a不超过int型所表示的最大整数,b也不超过,但是a+b可能超过!
}
return 0;
}
http://acm.hdu.edu.cn/showproblem.php?pid=2014
#include<iostream> #include<cstdio> #include<algorithm> using namespace std; int main() { int n; while(cin>>n) { double average=0; long long record[110];//评委打分可能会超过int的数据范围; for(int i=1;i<=n;i++) scanf("%lld",&record[i]); sort(record+1,record+n+1); for(int i=2;i<n;i++) average+=record[i]; printf("%.2f\n",average/(n-2)); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2097
#include<iostream> #include<cstdio> using namespace std; void trans_16(int number,char *p) { int flag=0; while(number) { int x=number%16; if(x<10) p[flag++]=x+'0'; else { if(x==10) p[flag++]='A'; if(x==11) p[flag++]='B'; if(x==12) p[flag++]='C'; if(x==13) p[flag++]='D'; if(x==14) p[flag++]='E'; if(x==15) p[flag++]='F'; } number/=16; } p[flag]='\0'; } void trans_12(int number,char *p) { int flag=0; while(number) { int x=number%12; if(x<10) p[flag++]=x+'0'; else { if(x==10) p[flag++]='A'; if(x==11) p[flag++]='B'; } number/=12; } p[flag]='\0'; } int main() { int number; char p_12[5],p_16[5]; while(cin>>number&&number!=0) { trans_16(number,p_16); trans_12(number,p_12); int sum=0; int num=number; while(num) { sum+=num%10; num/=10; } int sum_16=0,sum_12=0; for(int i=0;p_16[i]!='\0';i++) { if(isalpha(p_16[i])) sum_16+=(p_16[i]-'0'-7); else sum_16+=(p_16[i]-'0'); } for(int i=0;p_12[i]!='\0';i++) { if(isalpha(p_12[i])) sum_12+=(p_12[i]-'0'-7); else sum_12+=(p_12[i]-'0'); } if(sum==sum_16&&sum_16==sum_12) printf("%d is a Sky Number.\n",number); else printf("%d is not a Sky Number.\n",number); } return 0; }
http://acm.hdu.edu.cn/showproblem.php?pid=2098
#include<iostream> #include<cmath> using namespace std; bool is_prime(int x) { if(x<=1) return false; for(int i=2;i<=sqrt(x);i++) if(x%i==0) return false; return true; } int main() { int even_num; while(cin>>even_num) { int sum=0; for(int i=2;i<=even_num/2;i++) { int x=i,y=even_num-i; if(is_prime(x)&&is_prime(y)&&x!=y) sum++; } cout<<sum<<endl; } return 0; }