导包:
import re
re模块:
compile、match、search、findall、sub、split
compile:
re.compile(pattern[, flags])
用于编译正则表达式,生成一个正则表达式( Pattern )对象,供 match() 和 search() 这两个函数使用
pattern=re.compile('www')
result=pattern.match('wwww.baidu.com')
match
re.match(pattern, string, flags=0)
成功返回一个匹配的对象,否则返回None(从起始位置开始)
print(re.match('www','www.baidu.com'))#(0,3)
print(re.match('com','www.baidu.com'))#(None)
search
re.search(pattern, string,flags=0)
成功返回一个匹配的对象,否则返回None。(扫描整个字符串并返回第一个成功的匹配)
print(re.search('www','www.swww.com'))#(0,3)
print(re.search('com','www.swww.com'))#(9,12)
findall
findall(string[, pos[, endpos]]) 成功返回一个列表,没有则None
print(re.findall('www','wwww.swww.com')) #['wwww','www']
match 和 search 是匹配一次 findall 匹配所有
sub
re.sub(pattern, repl, string, count=0, flags=0)用于替换字符串中的匹配项。
repl : 替换的字符串,也可为一个函数。
string : 要被查找替换的原始字符串。
count : 模式匹配后替换的最大次数,默认 0 表示替换所有的匹配
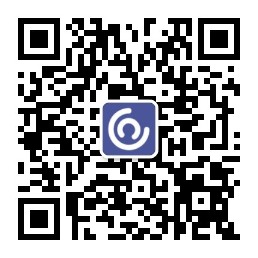
s='a a1 a2 a3'
pattern=r'\d'
repl1='99'
m=re.sub(pattern,repl1,s)
print(m)
def func(pattern):
num=pattern.group()
sum=int(num)+1
return str(sum)
m=re.sub(pattern,func,s)
print(m)
#a a99 a99 a99
#a a2 a3 a4
split
re.split(pattern, string[, maxsplit=0, flags=0])按照能够匹配的子串将字符串分割后返回列表
maxsplit 分隔次数,maxsplit=1 分隔一次,默认为 0,不限制次数。
m=re.split(r',','a,a1,a2,a3')
print(m)
#['a', 'a1', 'a2', 'a3']
. 任意字符除\n
^ 开头
$ 结尾
[] 范围 [0-9][a-z]
预定义
\s 空白 (空格)
\b 边界
\d 数字
\w 字母[0-9a-zA-Z_]
大写反面 \D 非数字
量词
* >=0
+ >=1
? 0,1
{m} 固定m位
{m,} >=m
{m,n} >=m <=n
text='a1anb22bgir33333f'
pattern=re.compile('[a-z][0-9]+[a-z]')
print(pattern.findall(text))
贪婪与非贪婪
量词默认是贪婪,通过?变成非贪婪
m=re.match(r'a(\d+)','a123a') #a123
m=re.match(r'a(\d+?)','a123a') #a1
分组
(w|w|w)与[123]
re.match(r'\w@(163|qq|126)\.(com|cn)$',email)
phone='010-12345678'
m=re.match(r'(\d{3}|\d{4})-(\d{8})$',phone)print(m.group()) #分组获取匹配的内容print(m.group(1))print(m.group(2))
不引用分组的内容
msg='<h1>11111</h1>'
m=re.match(r'<[a-zA-Z0-9]+>(.+)</[a-zA-Z0-9]+>$',msg)
print(m.group(1))#11111
引用分组匹配内容:
1.number \number
msg='<html><h1>11111</h1></html>'
m=re.match(r'<([a-zA-Z0-9]+)><([a-zA-Z0-9]+)>(.+)</\2></\1>$',msg)
print(m.group(1))#html
print(m.group(2))#h1
print(m.group(3))#1111
2.?P<名字> ?P=名字
msg='<html><h1>11111</h1></html>'
m=re.match(r'<(?P<name1>[a-zA-Z0-9]+)><(?P<name2>[a-zA-Z0-9]+)>(.+)</(?P=name2)></(?P=name1)>$',msg)
print(m.group(1))#html
print(m.group(2))#h1
print(m.group(3))#1111