set/multiset的查找
set.find(elem); //查找elem元素,返回指向elem元素的迭代器。 |
|
set.count(elem); //返回容器中值为elem的元素个数。对set来说,要么是0,要么是1。对multiset来说,值可能大于1。 |
|
set.lower_bound(elem); //返回第一个>=elem元素的迭代器。 |
|
set.upper_bound(elem); // 返回第一个>elem元素的迭代器。 |
|
set.equal_range(elem); //返回容器中与elem相等的上下限的两个迭代器。上限是闭区间,下限是开区间,如 [beg,end) 。以上函数返回两个迭代器,而这两个迭代器被封装在pair中。 |
|
例子:
#include <functional>
#include <algorithm>
#include <iostream>
#include <set>
using namespace std;
int main()
{
set<int> setInt;
setInt.insert(1);
setInt.insert(2);
setInt.insert(3);
setInt.insert(4);
setInt.insert(5);
set<int>::iterator it1 = setInt.find(4);
int elem1 = *it1;
cout<<"elem1: "<<elem1<<endl;
it1 = setInt.find(8);
if(it1 != setInt.end())
{
int elem2 = *it1;
cout<<"elem2: "<<elem2<<endl;
}
else
{
cout<<"8 不存在. "<<endl;
}
int iCout = setInt.count(3);
cout<<"cout(3) = "<<iCout<<endl;
set<int>::iterator it2 = setInt.lower_bound(3);
set<int>::iterator it3 = setInt.upper_bound(3);
int elem2 = *it2;
int elem3 = *it3;
cout<<"elem2: "<<elem2<<endl;
cout<<"elem3: "<<elem3<<endl;
pair<set<int>::iterator, set<int>::iterator> ii = setInt.equal_range(3);
cout<<"equal_range(3) 返回的第一个迭代器的值: "<<*(ii.first)<<endl;
cout<<"equal_range(3) 返回的第二个迭代器的值: "<<*(ii.second)<<endl;
system("pause");
return 0;
}
编译环境:
vc++ 2010学习版
运行结果:
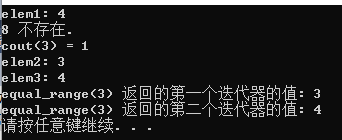