这个需求之前做过几遍了,但是因为都没有记录下来,导致再一次用到的时候又苦逼的去找了,特此记录下来,加深印象的同时当过了很久很久之后再需要用的的时候能够快速的实现.
申明一下,fastdfs是部署华为云的centos7的docker上.
1.部署坏境(坏境部署好的跳过第一步)
1.1 docker
yum -y install gcc
yum -y install gcc-c++
yum install -y yum-utils device-mapper-persistent-data lvm2
yum-config-manager --add-repo http://mirrors.aliyun.com/docker-ce/linux/centos/docker-ce.repo
yum makecache fast
yum -y install docker-ce
systemctl start docker
上面全部复制,一起粘贴即可
1.2 在docker上部署mysql
docker run -di --name=mysql -p 3306:3306 -e MYSQL_ROOT_PASSWORD=liuzian centos/mysql-57-centos7
1.3 FastDFS搭建
docker run -d --restart=always --privileged=true --net=host --name=fastdfs -e IP=139.9.xxx.xxx -e WEB_PORT=80 -v /usr/local/fastdfs:/var/local/fdfs registry.cn-beijing.aliyuncs.com/tianzuo/fastdfs
IP 后面是自己的服务器公网ip或者虚拟机ip,-e WEB_PORT=80 指定nginx端口
1.4 部署好测试一下
测试上传
//进入容器
docker exec -it fastdfs /bin/bash
//创建文件
echo "Hello 11111FastDFS!">index1.html
//测试文件上传
fdfs_test /etc/fdfs/client.conf upload index1.html
如果不能正常访问到的话,查看一下是否是端口没开放(占用)或者是防火墙没关.
2.在pom文件中导入相关的依赖
扫描二维码关注公众号,回复:
10747850 查看本文章
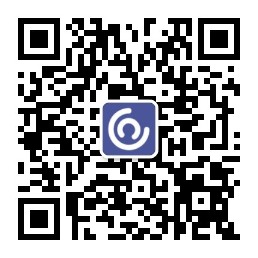
<dependency>
<groupId>com.github.tobato</groupId>
<artifactId>fastdfs-client</artifactId>
<version>1.26.7</version>
</dependency>
3.在application.yml中配置相关配置
3.1 fastdfs相关配置
fdfs:
so-timeout: 2500
connect-timeout: 600
thumb-image:
width: 100
height: 100
tracker-list:
- 139.9.130.249:22122
upload:
base-url: http://139.9.130.249/
allow-types:
- image/jpeg
- image/png
- image/bmp
- image/gif
- audio/mpeg
3.2 springmvc相关文件上床配置
spring:
servlet:
multipart:
max-file-size: 50MB
max-request-size: 100MB
4.配置工具类
4.1 UploadConfig
@Component
@ConfigurationProperties(prefix = "upload")
@Data
public class UploadConfig {
private String baseUrl;
private List<String> allowTypes;
}
4.2 UploadService
package com.lza.blog.utils;
import com.github.tobato.fastdfs.domain.fdfs.StorePath;
import com.github.tobato.fastdfs.service.FastFileStorageClient;
import com.lza.blog.config.UploadConfig;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
/**
* @program: fastdfs-demo
* @author: liuzian
* @create: 2020-4-7 18:28:04
**/
@Component
@EnableConfigurationProperties(UploadConfig.class)
public class UploadService {
private Log log = LogFactory.getLog(UploadService.class);
@Autowired
private FastFileStorageClient storageClient;
@Autowired
private UploadConfig uploadConfig;
public String uploadImage(MultipartFile file) {
// 1、校验文件类型
String contentType = file.getContentType();
if (!uploadConfig.getAllowTypes().contains(contentType)) {
throw new RuntimeException("文件类型不支持");
}
try {
// 3、上传到FastDFS
// 3.1、获取扩展名
String extension = StringUtils.substringAfterLast(file.getOriginalFilename(), ".");
// 3.2、上传
StorePath storePath = storageClient.uploadFile(file.getInputStream(), file.getSize(), extension, null);
// 返回路径
return uploadConfig.getBaseUrl() + storePath.getFullPath();
} catch (IOException e) {
log.error("【文件上传】上传文件失败!....{}", e);
throw new RuntimeException("【文件上传】上传文件失败!" + e.getMessage());
}
}
}
5.提供Controller返回url
package com.lza.blog.controller;
import com.lza.blog.utils.Result;
import com.lza.blog.utils.UploadService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
/**
* @Description:
* @Author: liuzian
* @Date: Create in 20:52 2020/4/7
*/
@RestController
@RequestMapping("/upload")
public class UploadController {
@Autowired
private UploadService uploadService;
/**
* 上传文件
* @param file
* @return
*/
@RequestMapping("/uploadImage")
public Result<String> uploadImage(MultipartFile file){
String url = uploadService.uploadImage(file);
return new Result<>("上传成功!",url);
}
}
6.页面调用测试
搞定!!!