调用接口见:
https://blog.csdn.net/FRESHET/article/details/100705065
后面又在评论补上了M5,M6,M7三个方法
package com.example.demo.controller;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.example.demo.pojo.Student;
@RestController
public class InterfaceController {
//普通接口访问并获取返回值
@RequestMapping("/i1")
public String i1() {
CloseableHttpClient client = null;
client = HttpClients.createDefault();
//http(s)://server_ip_address(:port)/xxx
//server_ip_address可以是localhost
//server_ip_address可以是127.0.0.1
//server_ip_address可以是内网IP
//server_ip_address可以是外网IP
HttpPost post = new HttpPost("http://10.98.106.217:8080/j3");
// post.setHeader("Content-Type","application/json;charset=UTF-8");
try {
HttpResponse response=client.execute(post);
HttpEntity entity = response.getEntity();
String entityJson=EntityUtils.toString(entity);
System.out.println(entityJson);
} catch (ClientProtocolException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "i1";
}
//向接口传递参数并获取返回值
@RequestMapping("/i2")
public String i2() {
CloseableHttpClient client = null;
client = HttpClients.createDefault();
HttpPost post = new HttpPost("http://10.98.106.217:8080/m2?username=aa&password=bb");
try {
HttpResponse response=client.execute(post);
HttpEntity entity = response.getEntity();
String entityJson=EntityUtils.toString(entity);
System.out.println(entityJson);
} catch (ClientProtocolException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "i2";
}
//向接口传递JSON并获取返回值
@RequestMapping("/i3")
public String i3() {
CloseableHttpClient client = null;
client = HttpClients.createDefault();
HttpPost post = new HttpPost("http://10.98.106.217:8080/m7");
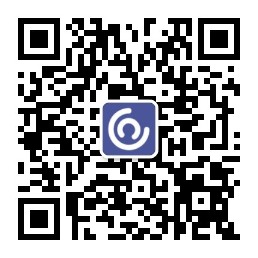
//1.字符串形式
String json="{\"bar\":\"ok\",\"world\":1.5,\"foo\":true,\"hello\":1}";
//2.JSON转换Map形式
Map<String,Object> map=new HashMap<String,Object>();
map.put("foo", 1.5f);
map.put("bar", "hello");
String json2=JSON.toJSONString(map);
//3.JSON转换对象形式
Student student = new Student();
student.setId(1);
student.setName("smith");
student.setWeight(185.5f);
student.setMale(true);
student.setLikes(Arrays.asList("football", "basketball", "volleyball"));
String json3=JSON.toJSONString(student);
//服务器接收
//{"name":"smith","weight":185.5,"id":1,"male":true,"likes":["football","basketball","volleyball"]}
try {
// StringEntity s = new StringEntity(json);
// StringEntity s = new StringEntity(json2);
StringEntity s = new StringEntity(json3);
post.setHeader("Content-Type","application/json;charset=UTF-8");
s.setContentEncoding("UTF-8");
s.setContentType("application/json");
post.setEntity(s);
HttpResponse response=client.execute(post);
HttpEntity entity = response.getEntity();
String entityJson=EntityUtils.toString(entity);
System.out.println(entityJson);
} catch (ClientProtocolException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "i3";
}
}