关于Android加载图片的问题
目前安卓加载图片三大框架:
1) Picasso
2)
Glide
3)
Fresco
简单谈一下使用Glide加载图片,Glide解决了快速加载图片的问题,但还有一个问题悬而未决:就是关于圆形图片和圆角图片问题,关于使用Glide动态加载圆角图片的问题,大家首先找到的很多blog如下所示:
compile 'com.github.bumptech.glide:glide:3.6.1'
GlideRoundTransform类
public class GlideRoundTransform extends BitmapTransformation {
private static float radius = 0f; public GlideRoundTransform(Context context) { this(context, 4); } public GlideRoundTransform(Context context, int dp) { super(context); this.radius = Resources.getSystem().getDisplayMetrics().density * dp; } @Override protected Bitmap transform(BitmapPool pool, Bitmap toTransform, int outWidth, int outHeight) { return roundCrop(pool, toTransform); } private static Bitmap roundCrop(BitmapPool pool, Bitmap source) { if (source == null) return null; Bitmap result = pool.get(source.getWidth(), source.getHeight(), Bitmap.Config.ARGB_8888); if (result == null) { result = Bitmap.createBitmap(source.getWidth(), source.getHeight(), Bitmap.Config.ARGB_8888); } Canvas canvas = new Canvas(result); Paint paint = new Paint(); paint.setShader(new BitmapShader(source, BitmapShader.TileMode.CLAMP, BitmapShader.TileMode.CLAMP)); paint.setAntiAlias(true); RectF rectF = new RectF(0f, 0f, source.getWidth(), source.getHeight()); canvas.drawRoundRect(rectF, radius, radius, paint); return result; } @Override public String getId() { return getClass().getName() + Math.round(radius); } }
然后动态加载:
glideRequest.load("https://www.baidu.com/img/bdlogo.png").transform(new GlideRoundTransform(context)).into(imageView);
当时一想的太简单,无非设置centerCrop的属性,继承BitmapTransformation重画。但是事实并不是这样的,在glide4.0上面 centerCrop和圆角图片有冲突只能显示一个,大部分都是上边的代码代码,发现这个在glide4.0上面直接报错无法识别。原因如下
查看new Centercrop()源码:
- /**
- * Scale the image so that either the width of the image matches the given width and the height of
- * the image is greater than the given height or vice versa, and then crop the larger dimension to
- * match the given dimension.
- *
- * Does not maintain the image's aspect ratio
- */
- public class CenterCrop extends BitmapTransformation {
- private static final String ID = "com.bumptech.glide.load.resource.bitmap.CenterCrop";
- private static final byte[] ID_BYTES = ID.getBytes(CHARSET);
- public CenterCrop() {
- // Intentionally empty.
- }
- @Deprecated
- public CenterCrop(@SuppressWarnings("unused") Context context) {
- this();
- }
- @Deprecated
- public CenterCrop(@SuppressWarnings("unused") BitmapPool bitmapPool) {
- this();
- }
- // Bitmap doesn't implement equals, so == and .equals are equivalent here.
- @SuppressWarnings("PMD.CompareObjectsWithEquals")
- @Override
- protected Bitmap transform(
- @NonNull BitmapPool pool, @NonNull Bitmap toTransform, int outWidth, int outHeight) {
- return TransformationUtils.centerCrop(pool, toTransform, outWidth, outHeight);
- }
- @Override
- public boolean equals(Object o) {
- return o instanceof CenterCrop;
- }
- @Override
- public int hashCode() {
- return ID.hashCode();
- }
- @Override
- public void updateDiskCacheKey(MessageDigest messageDigest) {
- messageDigest.update(ID_BYTES);
- }
- }
里面也是继承了BitmapTransformation这个类然后重画,我们调用transform()这个方法等于把系统的Centercrop这个方法给覆盖了,这下只能在自己自定义的BitmapTransformation将两个效果一起画出来了。
SO,要这样写
扫描二维码关注公众号,回复:
11070002 查看本文章
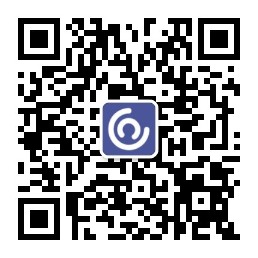
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_main);
- RequestOptions myOptions = new RequestOptions().centerCrop();
- Glide.with(this).load(R.drawable.picture1).apply(myOptions).into(imageView);
- }
RequestOptions myOptions = new RequestOptions().centerCrop().transform(new GlideRoundTransform(this,30));
Glide.with(this).load(R.drawable.item1).apply(myOptions).into(icon1);
重构GlideRoundTransform 类:
public class GlideRoundTransform extends BitmapTransformation {
private static float radius = 0f;
public GlideRoundTransform(Context context) {
this(context, 4);
}
public GlideRoundTransform(Context context, int dp) {
super(context);
this.radius = Resources.getSystem().getDisplayMetrics().density * dp;
}
@Override
protected Bitmap transform(BitmapPool pool, Bitmap toTransform, int outWidth, int outHeight) {
Bitmap bitmap = TransformationUtils.centerCrop(pool, toTransform, outWidth, outHeight);
return roundCrop(pool, bitmap);
}
private static Bitmap roundCrop(BitmapPool pool, Bitmap source) {
if (source == null) return null;
Bitmap result = pool.get(source.getWidth(), source.getHeight(), Bitmap.Config.ARGB_8888);
if (result == null) {
result = Bitmap.createBitmap(source.getWidth(), source.getHeight(), Bitmap.Config.ARGB_8888);
}
Canvas canvas = new Canvas(result);
Paint paint = new Paint();
paint.setShader(new BitmapShader(source, BitmapShader.TileMode.CLAMP, BitmapShader.TileMode.CLAMP));
paint.setAntiAlias(true);
RectF rectF = new RectF(0f, 0f, source.getWidth(), source.getHeight());
canvas.drawRoundRect(rectF, radius, radius, paint);
return result;
}
public String getId() {
return getClass().getName() + Math.round(radius);
}
@Override
public void updateDiskCacheKey(MessageDigest messageDigest) {
}
}
实现效果如下:
最后附上两个关于Glide资源(下载链接):
Glide4.0以下和以上,欢迎下载配套资源: