持续更新。。。。
1. 用两个栈实现一个队列;
考察点:队列和栈的特点
public class MyQueue {
Stack<Integer> stack1 = new Stack<Integer>();
Stack<Integer> stack2 = new Stack<Integer>();
public void appendTail(int item) {
stack1.push(item);
}
public int deleteHead() {
while(!stack2.isEmpty()){
return stack2.pop();
}
while(!stack1.isEmpty()){
stack2.push(stack1.pop());
}
return stack2.pop();
}
}
2. 用两个队列实现栈
考察点:同上
import java.util.LinkedList;
public class MyStack {
LinkedList<Integer> queue1=new LinkedList<Integer>();
LinkedList<Integer> queue2=new LinkedList<Integer>();
public void push(int value) {
queue1.addLast(value);
}
public int pop() {
if(size() > 0) {
if (queue2.size() > 0) {
putToAnother();
return queue2.removeFirst();
} else {
putToAnother();
return queue2.removeFirst();
}
} else {
return -1;
}
}
public void putToAnother() {
if (!queue1.isEmpty()) {
while(queue1.size() > 1) {
queue2.addLast(queue1.removeFirst());
}
} else if (!queue2.isEmpty()) {
while(queue2.size() > 1) {
queue1.addLast(queue2.removeFirst());
}
}
}
public int size() {
return queue1.size() + queue2.size();
}
}
3. 判断一棵二叉树是否是平衡二叉树
满足以下两点的就是平衡二叉树:
1.左右子树的高度差不能超过1
2.左右子树也是平衡二叉树
最直接的做法,就是遍历每个结点,借助一个获取树深度的递归函数,根据该结点的左右子树高度差判断是否平衡,然后递归地对左右子树进行判断。
public boolean isBalance(Node root) {
if(root==null) return true;
int left = depth(root.left);
int right = depth(root.right);
if(Math.abs(left-right)>1){
return false;
}
return true;
}
public int depth(Node root) {
if(root==null){
return 0;
}
int left = depth(root.left);
int right = depth(root.right);
return left > right ? left : right;
}
public boolean isBalanceTree (BinaryTree tree) {
if (tree.root == null) {
return true;
}
boolean isBalance = true;
Queue<Node> nodes = new LinkedList<Node>();
nodes.add(tree.root);
while (!nodes.isEmpty()) {
Node node = nodes.remove();
if (node.left != null) {
nodes.add(node.left);
}
if (node.right != null) {
nodes.add(node.right);
}
if (!isBalance(node)) isBalance = false;
break;
}
return isBalance;
}
扫描二维码关注公众号,回复:
11108471 查看本文章
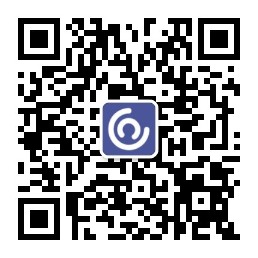
4. 单链表反转
// 递归
static Element reverseLinkedList(Element node) {
if (node ==null || node.next == null) {
return node;
} else {
Element headNode = reverseLinkedList(node.next);
node.next.next = node;
node.next = null;
return headNode;
}
}
// 循环
static Element reverseLinkedList1(Element node) {
Element previousNode = null;
Element currentNode = node;
Element headNode = null;
while (currentNode != null) {
Element nextNode = currentNode.next;
if (nextNode == null) {
headNode = currentNode;
}
currentNode.next = previousNode;
previousNode = currentNode;
currentNode = nextNode;
}
return headNode;
}
LinkedList的反转:
static LinkedList reverseLinkedList(LinkedList linkedList) {
LinkedList<Object> newLinkedList = new LinkedList<>();
for (Object object : linkedList) {
newLinkedList.add(0, object);
}
return newLinkedList;
}