使用python做一个IRC在线下载器
1.开发流程
2.软件流程
3.开始
3.0 准备工作
新建.py文件,安装json,requests,tkinter,os
库
规范mp3文件名'歌名-歌手.mp3'
3.1寻找API接口
笔者在这里用了http://doc.gecimi.com/en/latest/ 这里的API歌词接口,从该开发文档来看,返回值为Json
例如"http://gecimi.com/api/lyric/海阔天空/Beyond"
的返回值为:
{"code":0,"count":15,"result":[{"aid":1563419,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/166/16685/1668536.lrc","sid":1668536,"song":"海阔天空"},
{"aid":1567586,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/167/16739/1673997.lrc","sid":1673997,"song":"海阔天空"},
{"aid":1571906,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/167/16796/1679605.lrc","sid":1679605,"song":"海阔天空"},
{"aid":1573814,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/168/16819/1681961.lrc","sid":1681961,"song":"海阔天空"},
{"aid":1656038,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/179/17907/1790768.lrc","sid":1790768,"song":"海阔天空"},
{"aid":1718741,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/187/18757/1875769.lrc","sid":1875769,"song":"海阔天空"},
{"aid":2003267,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/226/22642/2264296.lrc","sid":2264296,"song":"海阔天空"},
{"aid":2020610,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/228/22889/2288967.lrc","sid":2288967,"song":"海阔天空"},{"aid":2051678,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/233/23323/2332322.lrc","sid":2332322,"song":"海阔天空"},
{"aid":2412704,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/283/28376/2837689.lrc","sid":2837689,"song":"海阔天空"},
{"aid":2607041,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/311/31116/3111659.lrc","sid":3111659,"song":"海阔天空"},
{"aid":2647055,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/316/31663/3166350.lrc","sid":3166350,"song":"海阔天空"},
{"aid":2657468,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/318/31803/3180339.lrc","sid":3180339,"song":"海阔天空"},
{"aid":3093833,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/377/37740/3774083.lrc","sid":3774083,"song":"海阔天空"},
{"aid":3161846,"artist_id":9208,"lrc":"http://s.gecimi.com/lrc/386/38612/3861244.lrc","sid":3861244,"song":"海阔天空"}]}
然后,我们用这段代码就可以把歌词解析出来
# coding: utf-8
import json
import requests
def down(song, singer):
a = requests.get(
r"http://gecimi.com/api/lyric/"+song+"/"+singer)
a.raise_for_status()
a = a.text
res = json.loads(a)
if(res["count"] == 0):
return "null"
return res["result"][0]["lrc"]
print(down("海阔天空", "beyond"))
其中,requests库的运用请参考我以前的blog传送门
json库的运用参考这里
至此,我们的抓取歌词代码就解决了
3.2 文件模块
3.2.1 选择文件弹窗
import tkinter.filedialog
def choice():
filename = tkinter.filedialog.askopenfilenames(
title=u'选择MP3文件', filetypes=[(u'MP3文件', '*.mp3')])
if filename != '':
return filename
else:
return "null"
root = tkinter.Tk() # 创建一个Tkinter.Tk()实例
root.withdraw() # 将Tkinter.Tk()实例隐藏
c = choice()
for a in range(len(c)):
print(c[a])
3.2.2 提取文件名
通过这段代码可以提取
print(c[a][len(os.path.dirname(c[a]))+1:])
参考这里
3.2.2.1 提取歌名和歌手
info = name.split(".")[0].split("-")
这段可以实现
3.2.3 下载
参考这里
代码:
扫描二维码关注公众号,回复:
11145888 查看本文章
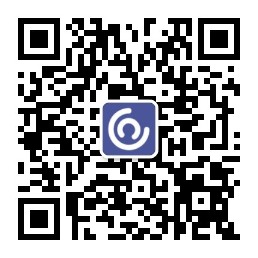
r = requests.get(down(info[0], info[1]))
with open(os.path.dirname(name)+"\\"+name.split(".")[0]+".lrc", "wb") as code:
code.write(r.content)
4.完成
全部代码:
# coding: utf-8
import os
import tkinter.filedialog
import json
import requests
def down(song, singer): # 歌词API
a = requests.get(
r"http://gecimi.com/api/lyric/"+song+"/"+singer)
a.raise_for_status()
a = a.text
res = json.loads(a)
if(res["count"] == 0):
return "null"
return res["result"][0]["lrc"]
def choice(): # 选择文件
filename = tkinter.filedialog.askopenfilenames(
title=u'选择MP3文件', filetypes=[(u'MP3文件', '*.mp3')])
if filename != '':
return filename
else:
return "null"
# 开始
root = tkinter.Tk() # 创建一个Tkinter.Tk()实例
root.withdraw() # 将Tkinter.Tk()实例隐藏
c = choice()
if(c != "null"):
for a in range(len(c)):
name = c[a][len(os.path.dirname(c[a]))+1:]
info = name.split(".")[0].split(" - ")
res = down(info[0], info[1])
if(res == "null"):#没有信息
continue
r = requests.get(res)
with open(os.path.dirname(name)+"\\"+name.split(".")[0]+".lrc", "wb") as code:
code.write(r.content)
print("成功")