原创转载请注明出处:https://www.cnblogs.com/agilestyle/p/13199558.html
前置条件
Project Directory
Maven Dependency
... <!--redis--> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency> ...
application.properties
... spring.redis.host=localhost spring.redis.port=6379 spring.redis.database=0 spring.redis.password= spring.redis.timeout=2000 spring.redis.pool.max-active=10 spring.redis.pool.max-wait=1000 spring.redis.pool.max-idle=10 spring.redis.pool.min-idle=5 spring.redis.pool.num-tests-per-eviction-run=1024 spring.redis.pool.time-between-eviction-runs-millis=30000 spring.redis.pool.min-evictable-idle-time-millis=60000 spring.redis.pool.soft-min-evictable-idle-time-millis=10000 spring.redis.pool.test-on-borrow=true spring.redis.pool.test-while-idle=true spring.redis.pool.block-when-exhausted=false ...
RedisTemplate CRUD
RedisConfiguration.java
1 package org.fool.redis.config; 2 3 import org.springframework.context.annotation.Bean; 4 import org.springframework.context.annotation.Configuration; 5 import org.springframework.data.redis.connection.RedisConnectionFactory; 6 import org.springframework.data.redis.core.RedisTemplate; 7 import org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer; 8 import org.springframework.data.redis.serializer.StringRedisSerializer; 9 10 @Configuration 11 public class RedisConfiguration { 12 @Bean 13 public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) { 14 RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>(); 15 redisTemplate.setConnectionFactory(redisConnectionFactory); 16 GenericJackson2JsonRedisSerializer jackson2JsonRedisSerializer = new GenericJackson2JsonRedisSerializer(); 17 redisTemplate.setValueSerializer(jackson2JsonRedisSerializer); 18 redisTemplate.setKeySerializer(new StringRedisSerializer()); 19 redisTemplate.setHashKeySerializer(new StringRedisSerializer()); 20 redisTemplate.setHashValueSerializer(jackson2JsonRedisSerializer); 21 redisTemplate.afterPropertiesSet(); 22 return redisTemplate; 23 } 24 }
UserService.java
扫描二维码关注公众号,回复:
11379181 查看本文章
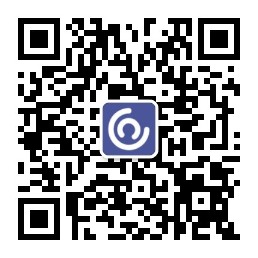
1 package org.fool.redis.service; 2 3 import com.github.pagehelper.PageHelper; 4 import com.github.pagehelper.PageInfo; 5 import lombok.extern.slf4j.Slf4j; 6 import org.fool.redis.mapper.UserMapper; 7 import org.fool.redis.model.User; 8 import org.springframework.beans.factory.annotation.Autowired; 9 import org.springframework.data.redis.core.RedisTemplate; 10 import org.springframework.data.redis.core.ValueOperations; 11 import org.springframework.stereotype.Service; 12 import tk.mybatis.mapper.entity.Example; 13 14 import java.util.Arrays; 15 import java.util.List; 16 17 @Service 18 @Slf4j 19 public class UserService { 20 public static final String CACHE_KEY_USER = "user:"; 21 22 @Autowired 23 private UserMapper userMapper; 24 25 @Autowired 26 private RedisTemplate redisTemplate; 27 28 public void createUser(User user) { 29 userMapper.insertSelective(user); 30 31 String key = CACHE_KEY_USER + user.getId(); 32 user = this.userMapper.selectByPrimaryKey(user.getId()); 33 redisTemplate.opsForValue().set(key, user); 34 } 35 36 public void updateUser(User user) { 37 userMapper.updateByPrimaryKeySelective(user); 38 39 String key = CACHE_KEY_USER + user.getId(); 40 user = this.userMapper.selectByPrimaryKey(user.getId()); 41 redisTemplate.opsForValue().set(key, user); 42 } 43 44 public User findUserById(Integer id) { 45 ValueOperations<String, User> operations = redisTemplate.opsForValue(); 46 String key = CACHE_KEY_USER + id; 47 User user = operations.get(key); 48 49 if (user == null) { 50 user = userMapper.selectByPrimaryKey(id); 51 operations.set(key, user); 52 } 53 54 return user; 55 } 56 57 public void deleteUser(int id) { 58 userMapper.deleteByPrimaryKey(id); 59 String key = CACHE_KEY_USER + id; 60 redisTemplate.delete(key); 61 } 62 63 public void findUser() { 64 log.info("select by primary key: where id = 100"); 65 User result = userMapper.selectByPrimaryKey(100); 66 log.info(result.toString()); 67 68 log.info("select by: where sex = 1"); 69 User user1 = new User(); 70 user1.setSex((byte) 1); 71 List<User> resultList = userMapper.select(user1); 72 log.info("sex=1 nums: {}", resultList.size()); 73 74 log.info("select by: where username = ? and password = ?"); 75 User user2 = new User(); 76 user2.setUsername("update100"); 77 user2.setPassword("update100"); 78 User user = userMapper.selectOne(user2); 79 log.info("user: {}", user); 80 } 81 82 public void selectByExample() { 83 log.info("select by example: where username = ? and password = ?"); 84 Example example = new Example(User.class); 85 Example.Criteria criteria = example.createCriteria(); 86 criteria.andEqualTo("username", "update100"); 87 criteria.andEqualTo("password", "update100"); 88 List<User> resultList = userMapper.selectByExample(example); 89 log.info("result list: {}", resultList); 90 } 91 92 public void selectByExampleAndLike() { 93 log.info("select by example with andLike: where username like = ?"); 94 Example example = new Example(User.class); 95 Example.Criteria criteria = example.createCriteria(); 96 criteria.andLike("username", "%update%"); 97 List<User> resultList = userMapper.selectByExample(example); 98 log.info("result list: {}", resultList); 99 } 100 101 public void selectByExampleOrderBy() { 102 log.info("select by example with order by: where username like = ? order by id desc"); 103 Example example = new Example(User.class); 104 example.setOrderByClause("username desc"); 105 Example.Criteria criteria = example.createCriteria(); 106 criteria.andLike("username", "%user%"); 107 List<User> resultList = userMapper.selectByExample(example); 108 log.info("result list: {}", resultList); 109 } 110 111 public void selectByExampleIn() { 112 log.info("select by example with in: where id in (100, 101)"); 113 Example example = new Example(User.class); 114 Example.Criteria criteria = example.createCriteria(); 115 criteria.andIn("id", Arrays.asList(100, 101)); 116 List<User> resultList = userMapper.selectByExample(example); 117 log.info("result list: {}", resultList); 118 } 119 120 public void selectByPageInfo(int pageNo, int pageSize) { 121 log.info("select by PageInfo: limit ?, ?"); 122 PageHelper.startPage(pageNo, pageSize); 123 List<User> userList = userMapper.selectAll(); 124 PageInfo<User> pageInfo = new PageInfo<>(userList); 125 long totalPage = pageInfo.getPages(); 126 long totalSize = pageInfo.getTotal(); 127 log.info("total page: {}, total size: {}, result list: {}", totalPage, totalSize, userList); 128 } 129 }
Test
1 package org.fool.redis.test; 2 3 import org.fool.redis.Application; 4 import org.fool.redis.model.User; 5 import org.fool.redis.service.UserService; 6 import org.junit.Assert; 7 import org.junit.jupiter.api.Test; 8 import org.springframework.beans.factory.annotation.Autowired; 9 import org.springframework.boot.test.context.SpringBootTest; 10 11 import java.util.Random; 12 13 @SpringBootTest(classes = Application.class) 14 public class RedisTemplateTest { 15 @Autowired 16 private UserService userService; 17 18 @Test 19 public void testCreate() { 20 User user = new User(); 21 user.setId(1001); 22 user.setUsername("hello"); 23 user.setPassword("world"); 24 user.setSex((byte) new Random().nextInt(2)); 25 userService.createUser(user); 26 } 27 28 @Test 29 public void testUpdate() { 30 User user = new User(); 31 user.setId(1001); 32 user.setPassword("helloworld"); 33 userService.updateUser(user); 34 } 35 36 @Test 37 public void testSelect() { 38 User result = userService.findUserById(1001); 39 Assert.assertEquals("hello", result.getUsername()); 40 } 41 42 @Test 43 public void testDelete() { 44 userService.deleteUser(1001); 45 } 46 }