一.策略模式
在策略模式(Strategy Pattern)中,一个类的行为或其算法可以在运行时更改。这种类型的设计模式属于行为型模式。
在策略模式中,我们创建表示各种策略的对象和一个行为随着策略对象改变而改变的 context 对象。策略对象改变 context 对象的执行算法。
二.一个商场促销例子
1.抽象类
package strategic; /** * 现金收费抽象类 * @author wuwuyong * */ abstract class CashSuper { public double acceptCash(double money) { return money; } }
2.正常收费子类
package strategic; /** * 正常收费子类 * @author wuwuyong * */ public class CashNormal extends CashSuper { public double acceptCash(double money) { return money; } }
3.打折收费子类
package strategic; /** * 打折收费子类 * @author wuwuyong * */ public class CashRebate extends CashSuper { private double moneyRebate = 1; public CashRebate(Double moneyRebate) { this.moneyRebate = moneyRebate; } public double acceptCash(double money) { return money * this.moneyRebate; } }
4.返利收费子类
package strategic; /** * 返利收费子类 * @author wuwuyong * */ public class CashReturn extends CashSuper { private double moneyCondition = 0; private double moneyReturn = 0; public CashReturn(double moneyCondition, double moneyReturn) { this.moneyCondition = moneyCondition; this.moneyReturn = moneyReturn; } public double acceptCash(double money) { double result = money; if (money >= moneyCondition) { result = money - moneyReturn; } return result; } }
5.CashContext
package strategic; public class CashContext { private CashSuper cs; public CashContext(String type) { switch (type) { case "正常收费": cs = new CashNormal(); break; case "满300返100": cs = new CashReturn(300, 100); break; case "打8折": cs = new CashRebate(0.8); break; } } public double getResult(double money) { return cs.acceptCash(money); } }
6.测试
package strategic; public class Test { public static void main(String[] args) { double result = 0; CashContext normal = new CashContext("正常收费"); result = normal.getResult(300); System.out.println("正常收费:"+result); CashContext cashReturn = new CashContext("满300返100"); result = cashReturn.getResult(300); System.out.println("满300返100:"+result); CashContext cashRebate = new CashContext("打8折"); result = cashRebate.getResult(300); System.out.println("打8折:"+result); } }
扫描二维码关注公众号,回复:
11379470 查看本文章
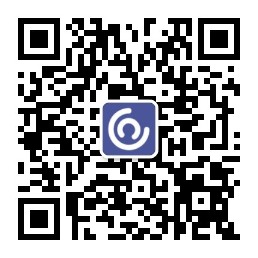