JS轮播图自动播放和点击联动功能!
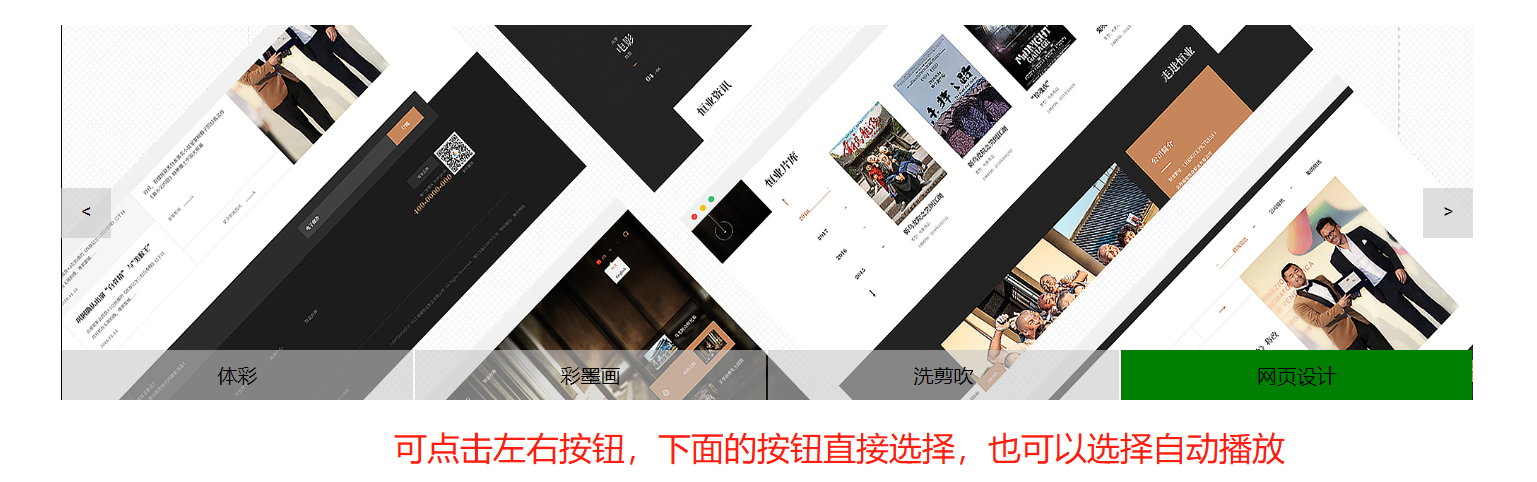
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
.box{width: 1130px;height: 300px;margin: 20px auto;position: relative;
overflow: hidden;
}
.imgbox{height: 300px;position: absolute;left:0;}
.imgbox a{float: left;}
.imgbox img{width: 1130px;height: 300px;}
</style>
</head>
<body>
<div class="box">
<div class="imgbox">
<a><img src="https://img.zcool.cn/community/013c185ec5e1a0a801214d72ae5567.jpg"></a>
<a><img src="https://img.zcool.cn/community/019b5a5ec5ea6ba801209b864afbad.png" alt=""></a>
<a><img src="https://img.zcool.cn/community/0155475ec5ea82a801209b86526dc0.png" alt=""></a>
<a><img src="https://img.zcool.cn/community/0133395ec5ea9fa801209b86533992.png" alt=""></a>
<a><img src="https://img.zcool.cn/community/013c185ec5e1a0a801214d72ae5567.jpg"></a>
</div>
</div>
</body>
<script src="../day24/move.js"></script>
<script>
// 可自定义的轮播图
class Banner{
constructor(ops){
// 处理参数
this.imgbox = ops.imgbox;
this.list = ops.list!==false ? true : false;
this.btn = ops.btn!==false ? true : false;
this.autoPlay = ops.autoPlay!==false ? true : false;
// 默认索引
this.index = 0;
// 获取所有图片
this.items = this.imgbox.children;
// 功能1. 初始化布局:完善imgbox的宽度
this.init();
// 功能B. 根据参数决定是否创建按键
this.isBtn();
// 功能L. 根据参数决定是否创建list
this.isList();
// 功能A. 根据参数决定是否需要自动播放
this.isAutoPlay();
}
init(){
this.imgbox.style.width = this.items.length * this.items[0].offsetWidth + "px";
}
isAutoPlay(){
// A1.如果是false,终止当前函数,不执行后面代码,不做这个功能
if(!this.autoPlay) return;
// 如果执行到此处,说明上面过来的是true,没有执行return
// A2.立即开启计时器,执行点击右按钮要做的事情
this.t = setInterval(() => {
// 右按钮被点击时要执行的函数
this.changeIndex(-1);
}, 2000);
var that = this;
// A3.鼠标进入停止
this.imgbox.parentNode.onmouseover = function(){
clearInterval(that.t);
}
// A4.鼠标离开继续
this.imgbox.parentNode.onmouseout = function(){
that.t = setInterval(() => {
that.changeIndex(-1);
}, 2000);
}
}
isList(){
// L1.如果是false,终止当前函数,不执行后面代码,不做这个功能
if(!this.list) return;
// 如果执行到此处,说明上面过来的是true,没有执行return
// L2.为了实现无缝轮播,将第一张图复制了一遍多了一张,记得去掉
var num = this.items.length-1;
// 假设的图片的名字,将来必然是数据
var arr = ["体彩","彩墨画","洗剪吹","网页设计"];
// L3.创建list的容器
this.list = document.createElement("div");
this.list.style.cssText = `position: absolute;width: 100%;height: 40px;line-height: 40px;display: flex;bottom: 0;left:0;text-align: center;`;
// L4.根据图片的数量,创建对应个span
var str = "";
for(var i=0;i<num;i++){
str += `<span key=${i} style="flex: 1;margin: 0 1px;background: rgba(200,200,200,0.6)">${arr[i]}</span>`;
}
// L5.将span放在list容器中
this.list.innerHTML = str;
// 设置索引对应span的当前项
this.list.children[this.index].style.background = "green";
// L6.将list容器插入页面
this.imgbox.parentNode.appendChild(this.list);
// L7.绑定list中span的事件
this.listAddEvent();
}
listAddEvent(){
var that = this;
var aspan = this.list.children;
for(var i=0;i<aspan.length;i++){
aspan[i].onclick = function(){
// L8.获取span身上的索引:保证索引的数据是数值,防止将来出现问题
that.index = parseInt(this.getAttribute("key"));
// L9.执行move,显示对应图片
that.move();
// L11.修改当前背景色
that.setActive()
}
}
}
setActive(){
// 取消所有
for(var i=0;i<this.list.children.length;i++){
this.list.children[i].style.background = "rgba(200,200,200,0.6)";
}
// 为了处理最后一张图(其实就是复制的第一张图)的索引,单独判断,保存变量
// 计算索引
var k = this.index===this.items.length-1 ? 0 : this.index;
// 设置当前索引对应的span的背景色
this.list.children[k].style.background = "green";
}
isBtn(){
// B1.如果是false,终止当前函数,不执行后面代码,不做这个功能
if(!this.btn) return;
// 如果执行到此处,说明上面过来的是true,没有执行return
// B2.创建并插入左按钮
this.left = document.createElement("input");
this.left.style.cssText = `width: 40px;height: 40px;background: rgba(200,200,200,0.6);border:none;position: absolute;top:130px;left:0`;
this.left.value = "<"
this.imgbox.parentNode.appendChild(this.left);
// 创建并插入右按钮
this.right = document.createElement("input");
this.right.style.cssText = `width: 40px;height: 40px;background: rgba(200,200,200,0.6);border:none;position: absolute;top:130px;right:0`;
this.right.value = ">";
this.imgbox.parentNode.appendChild(this.right);
this.left.type = this.right.type = "button";
// B3.绑定左右按钮的点击事件
this.btnAddEvent();
}
btnAddEvent(){
var that = this;
this.left.onclick = function(){
// B4-1.改变索引
that.changeIndex(1)
}
this.right.onclick = function(){
// B4-2.改变索引
that.changeIndex(-1);
}
}
changeIndex(d){
if(d===1){
if(this.index===0){
this.index = this.items.length-2;
this.imgbox.style.left = -(this.items.length-1) * this.items[0].offsetWidth + "px";
}else{
this.index--;
}
}else{
if(this.index===this.items.length-1){
this.index = 1;
this.imgbox.style.left = 0;
}else{
this.index++;
}
}
// B5.移动大框,切换图片
this.move();
// L12.点击按钮时,设置索引对应的list的span的背景色
// 设置之前先判断,list出否存在,不存在,不设置样式
this.list && this.setActive();
}
move(){
// B6.显示图片
// L10.显示图片
// 神器:console.log
// console.log(this.index);
move(this.imgbox, {left: -this.index*this.items[0].offsetWidth});
}
}
// 准备执行方式
new Banner({
imgbox:document.querySelector(".box .imgbox"), // 必传
list:true, // 可选,默认为true
btn:true, // 可选,默认为true
autoPlay:true // 可选,默认为true
})
</script>
</html>
move.js
function move(ele, data, cb){
clearInterval(ele.t);
ele.t = setInterval(() => {
var onoff = true;
for(var i in data){
var iNow = parseInt(getStyle(ele,i));
var speed = (data[i] - iNow)/8;
speed = speed>0 ? Math.ceil(speed) : Math.floor(speed);
ele.style[i] = iNow + speed + "px";
if(iNow != data[i]){
onoff = false;
}
}
if(onoff){
clearInterval(ele.t);
// 结束的位置要做什么,交给使用者决定
// 传个功能进来,传函数
cb && cb();
}
}, 30);
}
// 动画结束后,再执行其他效果
// 获取样式的兼容处理
function getStyle(ele,attr){
if(ele.currentStyle){
return ele.currentStyle[attr];
}else{
return getComputedStyle(ele, false)[attr];
}
}