1.1 pagehelper介绍和使用
PageHelper是一款好用的开源免费的Mybatis第三方物理分页插件。
原本以为分页插件,应该是很简单的,然而PageHelper比我想象的要复杂许多,它做的很强大,也很彻底,强大到使用者可能并不需要这么多功能,彻底到一参可以两用。
1.1.1 springboot下使用
- 依赖包导入(这里划重点!!!有坑!!)
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
<version>最新版本</version>
</dependency>
这是从官网上的标准形式,但是注意了。pagehelper与springboot集成的话,不能用这个包,这样会导致分页失效!
正确的是下面这种导入方式:
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.2.3</version>
</dependency>
可以看到跟springboot集成的包大多数后面都是以spring-boot-starter结尾。。。。
刚开始我用的第一种,代码也全都正确,就是没有分页效果。。。这坑!!
- User
public class User {
private Integer id;
@Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", password='" + password + '\'' +
", uid=" + uid +
", admin=" + admin +
", email='" + email + '\'' +
", phonenumber=" + phonenumber +
", classid=" + classid +
'}';
}
private String name;
private String password;
private Integer uid;
private Integer admin;
private String email;
private Integer phonenumber;
private Integer classid;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name == null ? null : name.trim();
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password == null ? null : password.trim();
}
public Integer getUid() {
return uid;
}
public void setUid(Integer uid) {
this.uid = uid;
}
public Integer getAdmin() {
return admin;
}
public void setAdmin(Integer admin) {
this.admin = admin;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email == null ? null : email.trim();
}
public Integer getPhonenumber() {
return phonenumber;
}
public void setPhonenumber(Integer phonenumber) {
this.phonenumber = phonenumber;
}
public Integer getClassid() {
return classid;
}
public void setClassid(Integer classid) {
this.classid = classid;
}
}
- UserMapper
@Repository
public interface UserMapper {
long countByExample(UserExample example);
int deleteByExample(UserExample example);
int insert(User record);
int insertSelective(User record);
List<User> selectAllUser();
User selectByExample(UserExample example);
User selectBynameANDpassword(String name,String password);
int updateByExampleSelective(@Param("record") User record, @Param("example") UserExample example);
int updateByExample(@Param("record") User record, @Param("example") UserExample example);
}
这里只用selectAllUser()这个方法就行了!
- UserMapper.xml
<select id="selectAllUser" resultType="com.hifix.springbootmyfirstproject.pojo.User">
select *from user
</select>
鄙人还是比较喜欢这种xml的方式声明sql,并不是觉得注解的不好,只是觉得这种方式使得代码的整体可读性更好!
- controller
//测试分页信息
@RequestMapping("/UserInfo")
public String presentUser(Model model,
@RequestParam(required = false,defaultValue="1",value="pageNum")Integer pageNum,
@RequestParam(defaultValue="2",value="pageSize")Integer pageSize)throws Exception {
if(pageNum == null){
pageNum = 1; //设置默认当前页
}
if(pageNum <= 0){
pageNum = 1;
}
if(pageSize == null){
pageSize = 2; //设置默认每页显示的数据数
}
System.out.println("当前页是:"+pageNum+"显示条数是:"+pageSize);
//1.引入分页插件,pageNum是第几页,pageSize是每页显示多少条,默认查询总数count
//2.紧跟的查询就是一个分页查询-必须紧跟.后面的其他查询不会被分页,除非再次调用PageHelper.startPage
PageHelper.startPage(pageNum,pageSize);
List<User> userList =pageimpl.getAllUser();
System.out.println("分页数据:"+userList);
//3.使用PageInfo包装查询后的结果,5是连续 显示的条数,结果list类型是Page<E>
PageInfo<User> pageInfo = new PageInfo<User>(userList,pageSize);
//4.使用model/map/modelandview等带回前端
model.addAttribute("pageInfo",pageInfo);
return "bootstraptable";
}
- 前端
<!doctype html>
<html lang="en" xmlns:th="http://www.thymeleaf.org" xmlns:c="http://www.w3.org/1999/xhtml">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>Hello, Bootstrap Table!</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous">
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.6.3/css/all.css" integrity="sha384-UHRtZLI+pbxtHCWp1t77Bi1L4ZtiqrqD80Kn4Z8NTSRyMA2Fd33n5dQ8lWUE00s/" crossorigin="anonymous">
<link rel="stylesheet" href="https://unpkg.com/[email protected]/dist/bootstrap-table.min.css">
</head>
<body>
<table data-toggle="table">
<thead>
<tr>
<th>name</th>
<th>password</th>
<th>uid</th>
</tr>
</thead>
<tbody >
<tr th:each="c:${pageInfo.list}">
<td>[[${c.name}]]</td>
<td>[[${c.password}]]</td>
<td>[[${c.uid}]]</td>
</tr>
</tbody>
</table>
<br/>
<div class="modal-footer no-margin-top">
<div class="col-md-6">
当前第 [[${pageInfo.pageNum}]]页,共 [[${pageInfo.pages}]] 页.一共 [[${pageInfo.total}]] 条记录
</div>
<ul class="pagination pull-right no-margin">
<li th:if="${pageInfo.hasPreviousPage}">
<a th:href="'/UserInfo?pageNum=1'">首页</a>
</li>
<li class="prev" th:if="${pageInfo.hasPreviousPage}">
<a th:href="'/UserInfo?pageNum='+${pageInfo.prePage}">
<i class="ace-icon fa fa-angle-double-left"></i>
</a>
</li>
<!--遍历条数-->
<li th:each="nav:${pageInfo.navigatepageNums}">
<a th:href="'/UserInfo?pageNum='+${nav}" th:text="${nav}" th:if="${nav != pageInfo.pageNum}"></a>
<span style="font-weight: bold;background: #6faed9;" th:if="${nav == pageInfo.pageNum}" th:text="${nav}" ></span>
</li>
<li class="next" th:if="${pageInfo.hasNextPage}">
<a th:href="'/UserInfo?pageNum='+${pageInfo.nextPage}">
<i class="ace-icon fa fa-angle-double-right"></i>
</a>
</li>
<li>
<a th:href="'/UserInfo?pageNum='+${pageInfo.pages}">尾页</a>
</li>
</ul>
</div>
<div>当前页号:<span th:text="${pageInfo.pageNum}"></span></div>
<div>每页条数:<span th:text="${pageInfo.pageSize}"></span></div>
<div>起始行号:<span th:text="${pageInfo.startRow}"></span></div>
<div>终止行号:<span th:text="${pageInfo.endRow}"></span></div>
<div>总结果数:<span th:text="${pageInfo.total}"></span></div>
<div>总页数:<span th:text="${pageInfo.pages}"></span></div>
<hr />
<div>是否为第一页:<span th:text="${pageInfo.isFirstPage}"></span></div>
<div>是否为最后一页:<span th:text="${pageInfo.isLastPage}"></span></div>
<div>是否有前一页:<span th:text="${pageInfo.hasPreviousPage}"></span></div>
<div>是否有下一页:<span th:text="${pageInfo.hasNextPage}"></span></div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js" integrity="sha512-bLT0Qm9VnAYZDflyKcBaQ2gg0hSYNQrJ8RilYldYQ1FxQYoCLtUjuuRuZo+fjqhx/qtq/1itJ0C2ejDxltZVFg==" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="sha384-UO2eT0CpHqdSJQ6hJty5KVphtPhzWj9WO1clHTMGa3JDZwrnQq4sF86dIHNDz0W1" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" integrity="sha384-JjSmVgyd0p3pXB1rRibZUAYoIIy6OrQ6VrjIEaFf/nJGzIxFDsf4x0xIM+B07jRM" crossorigin="anonymous"></script>
<script src="https://unpkg.com/[email protected]/dist/bootstrap-table.min.js"></script>
</body>
</html>
- 效果
2.1 总结
相比其他分页插件而言,pagehelper强大的很多。个人觉得用起来还是挺香的。
扫描二维码关注公众号,回复:
11474838 查看本文章
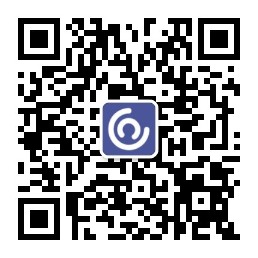