首先单例模式是希望只产生一个对象,主要用做工具类,所以使用私有化构造方法。
原理:用private关键字声明的构造器,其访问权限是private,于是它只能被包含它的类自身所访问,而无法在类的外部调用,故而可以阻止对象的生成。所以,如果一个类只有一个私有构造器,而没有任何公有构造器,是无法生成任何对象的。
单例模式五种写法:
(1)饿汉式:
package com.androidtv.pos.single;
/**
* @author wuqiqi
* date on 2020/8/7
* describe 饿汉式
*/
public class HSingleton {
private HSingleton(){}
private static HSingleton instance = new HSingleton();
public static HSingleton getInstance(){
return instance;
}
}
顾名思义,就是提前生成,好处是没有线程安全的问题,坏处是提前加载会影响性能,有可能不使用会浪费内存空间。
(2)懒汉式:
线程不安全:
package com.androidtv.pos.single;
/**
* @author wuqiqi
* date on 2020/8/7
* describe 懒汉式(线程不安全)
*/
public class LSingleton {
private LSingleton(){}
private static LSingleton instance;
public static LSingleton getInstance(){
if(instance == null){
instance = new LSingleton();
}
return instance;
}
}
顾名思义,就是延迟生成,在调用的时候生成,好处是调用时候生成,不会浪费内存空间,性能高,坏处有线程安全问题。
线程安全:
就是加了 synchronized 修饰符
package com.androidtv.pos.single;
/**
* @author wuqiqi
* date on 2020/8/7
* describe 懒汉式(线程安全)
*/
public class LSSingleton {
private LSSingleton(){};
private static LSSingleton instance;
public static synchronized LSSingleton getInstance(){
if(instance == null){
instance = new LSSingleton();
}
return instance;
}
}
好处是调用时候生成,不会浪费内存空间,线程安全,坏处是性能低,每次调用都要进行synchronized判断。
扫描二维码关注公众号,回复:
11541321 查看本文章
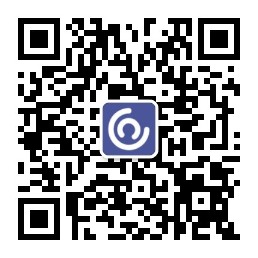
由这个改善为 双重检测方式:
(3)双重检测:
package com.androidtv.pos.single;
/**
* @author wuqiqi
* date on 2020/8/7
* describe 双重检测
*/
public class DoubleSingleton {
private DoubleSingleton(){}
private volatile static DoubleSingleton doubleSingleton;
public static DoubleSingleton getInstance(){
if(doubleSingleton == null){
synchronized (DoubleSingleton.class){
if(doubleSingleton == null){
doubleSingleton = new DoubleSingleton();
}
}
}
return doubleSingleton;
}
}
这样既保证了线程安全,又比直接上锁提高了执行效率,还节省了内存空间,但这不是完全线程安全。(推荐使用)
(4)静态内部类:
package com.androidtv.pos.single;
/**
* @author wuqiqi
* date on 2020/8/7
* describe 静态内部类
*/
public class StaticSingleton {
private StaticSingleton(){}
private static class StaticSingletonHolder{
private static final StaticSingleton instance = new StaticSingleton();
}
public static final StaticSingleton getInstance(){
return StaticSingletonHolder.instance;
}
}
也有饿汉式线程安全优点,又因为内部静态类初始化是在外部类静态方法调用的时候才初始化,所以初始化时机也是使用的时候,也节省了内存空间,避免不必要浪费。(推荐使用)
(5)枚举形式:
package com.androidtv.pos.single;
/**
* @author wuqiqi
* date on 2020/8/7
* describe 枚举类型
*/
public enum ESingleton {
INSTANCE;
}
线程安全,但耗费资源多,java是不推荐使用枚举