一、注意事项
C语言中使用字符串时,使用#include <string.h>
C++中使用#include <string>
二、字符串的操作
1.字符串拷贝全部: char* strcpy(char* dest,const char* src);
把src所指向的字符串复制到dest所指向的空间中,'\0'也会拷贝。
#include <string.h>
char dest_str[100] = { 0 };
const char* str = "hello\0 world";
strcpy(dest_str, str);
printf("%s\n", dest_str);//hello
2.字符串拷贝n个:char* strncpy(char* dest,const char* src,size_t n);
把src所指向的字符串的前n个字符复制到dest所指向的空间中,如果包含'\0',也会拷贝。
#include <stdio.h>
char str[] = "10,hello" ;
int a=0;
char new_str[100] = {0};
sscanf(str, "%d,%s", &a, new_str);
printf("%d\n", a);//10
printf("%s\n", new_str);//hello
3.字符串连接:char* strcat(char* dest,const char* src);
把src所指向的字符串连接到到dest所指向的空间的尾部,'\0'也会追加过去,返回dest字符串的首地址。
#include <string.h>
char dest_str[100] = "My First Prigram:";
char* str = "hello\0 world";
strcat(dest_str, str);
printf("%s\n", dest_str);//My First Prigram:hello
4.字符串比较:int strcmp(const char* s1,const char* s2);
比较s1与s2的字符的ASCII大小,相等返回0。
#include <string.h>
char dest_str[100] = "hello";
char* str = "hello\0 world";
int ret = strcmp(dest_str, str);
printf("%d\n", ret);//0,相同
5.字符串比较:int strncmp(const char* s1,const char* s2,size_t n);
比较s1与s2前n个字符的ASCII大小,相等返回0。
#include <string.h>
char dest_str[100] = "hello world" ;
char* str = "hello WORLD";
int ret = strncmp(dest_str, str,6);
printf("%d\n", ret);//0,相同
6.字符串格式化:int sprintf(char* str,const char* format,...);
根据参数format字符串来转换并格式化数据,将结果输出到str空间中,直到出现字符串结束符'\0'为止。
#include <stdio.h>
char str[] = "hello" ;
int a = 10;
char new_str[100];
sprintf(new_str, "%d %s",a,str);
printf("%s\n", new_str);//10 hello
7.字符串格式化:int sscanf(char* str,const char* format,...);
从指定的str中读取数据,根据参数format字符串来转换并格式化数据。
#include <string.h>
char dest_str[100] = "hello";
char* str = "hello\0 world";
int ret = strcmp(dest_str, str);
printf("%d\n", ret);//0,相同
8.查找字符出现的位置:char* strchr(const char *s,int c);
在字符串s中查找字符c出现的位置。
#include <string.h>
char str[] = "hello world";
char* new_str = strchr(str, 'l');
printf("%s\n", new_str);//hello
9.查找字符串出现的位置:char* strstr(const char *str,const char *s);
在字符串str中查找字符串s出现的位置。
扫描二维码关注公众号,回复:
11842865 查看本文章
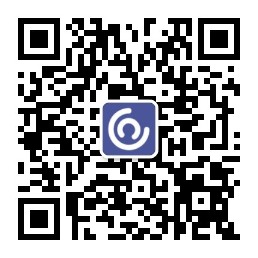
#include <string.h>
char str[] = { "hello world" };
char* new_str = strstr(str,"o");
printf("%s\n", new_str);//hello
10.切割字符串:char* strtok(char *str,const char *s);
在字符串str中发现字符串s出现时,将s改为\0字符。在第一次调用时,str参数必须赋值;往后调用则将str设置成NULL。strtok()会破坏源字符串。
#include <string.h>
char str[] = "hello,world,ok,fine";
char* new_str = strtok(str, ",");
while (new_str)
{
printf("%s\n", new_str);
new_str=strtok(NULL,",");
}
11.字符串转数字:int atoi(const char *s);
将字符串s转成整型。atoi扫描s时,会跳过前面的空字符串,直到遇到数字或正负号才开始转换,遇到非数字或\0结束转换。
#include <stdlib.h>
char str[] = " 123.5ab" ;
int num = atoi(str);
printf("%d\n", num);
double d = atof(str);
printf("%f\n", d);