imgaug
This python lib rary helps you with augmenting images for your machine learning projects.
It converts a set of input images into a new, much larger set of slightly altered images.
1. Load and Augment an Image
import imageio
import imgaug as ia
from imgaug import augmenters as iaa
import numpy as np
%matplotlib inline
1.1 Load and Show an Image
image = imageio.imread("img/cat1.png")
print("Original:")
ia.imshow(image)
Output:
Original:
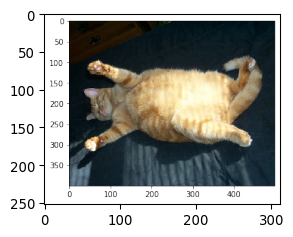
1.2 Augment an Image
ia.seed(4)
rotate = iaa.Affine(rotate=(25,-25))
image_aug = rotate(image=image)
print("Augmented:")
ia.imshow(image_aug)
Output:
Augmented:
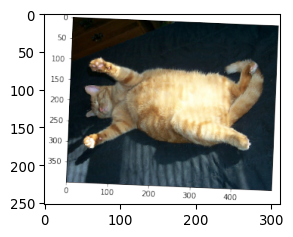
1.3 Augment a Batch of Images
images = [image,image,image,image]
images_aug = rotate(images=images)
print("Augmented batch:")
ia.imshow(np.hstack(images_aug))
Output:
Augmented batch:
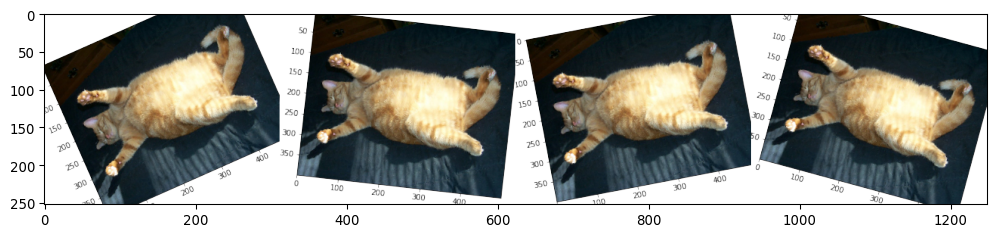
1.4 Use Many Augmentation Techniques Simultaneously
1.4.1 same order
# combine several augmentation techniques on one image
# augment a batch of images
seq = iaa.Sequential([
iaa.Affine(rotate=(-25, 25)),
iaa.AdditiveGaussianNoise(scale=(10, 60)),
"""
Crop by default retains the input image size,
i.e. after removing pixels it resizes the remaining image back to the input size.
If you instead prefer to not resize back to the original image size,
instantiate Crop as Crop(..., keep_size=False).
"""
iaa.Crop(percent=(0, 0.2))
])
images_aug = seq(images=images)
print("Augmented:")
ia.imshow(np.hstack(images_aug))
Output:
Augmented:
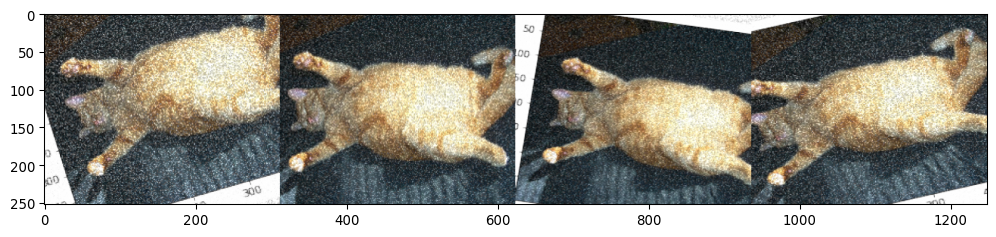
1.4.2 random order
# combine several augmentation techniques on one image
# random order is sampled once per batch
# augment an image eight times via a loop
seq = iaa.Sequential([
iaa.Affine(rotate=(-25, 25)),
iaa.AdditiveGaussianNoise(scale=(30, 90)),
iaa.Crop(percent=(0, 0.4))
], random_order=True)
# augment one image as one batch
images_aug = [seq(image=image) for _ in range(8)]
print("Augmented:")
ia.imshow(ia.draw_grid(images_aug, rows=2, cols=4))
Output:
Augmented:
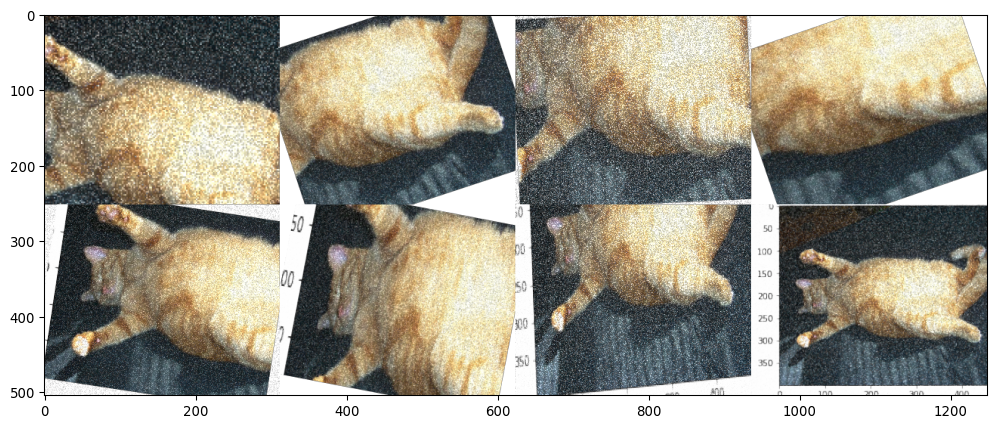
1.5 Augment Images of Different Sizes
seq = iaa.Sequential([
iaa.CropAndPad(percent=(-0.2, 0.2), pad_mode="edge"), # crop and pad
iaa.AddToHueAndSaturation((-60, 60)), # change color
iaa.ElasticTransformation(alpha=90, sigma=9), # water-like effect
iaa.Cutout() # replace one squared area within the image by a constant intensity value
], random_order=True)
# load images with different sizes
images_different_sizes = [
imageio.imread("img/cat2.jpg"),
imageio.imread("img/cat3.jpeg")
]
# augment them as one batch
images_aug = seq(images=images_different_sizes)
# visualize the results
print("Image 0 (input shape: %s, output shape: %s)" % (images_different_sizes[0].shape, images_aug[0].shape))
ia.imshow(np.hstack([images_different_sizes[0], images_aug[0]]))
print("Image 1 (input shape: %s, output shape: %s)" % (images_different_sizes[1].shape, images_aug[1].shape))
ia.imshow(np.hstack([images_different_sizes[1], images_aug[1]]))
Output:
Image 0 (input shape: (561, 999, 3), output shape: (561, 999, 3))
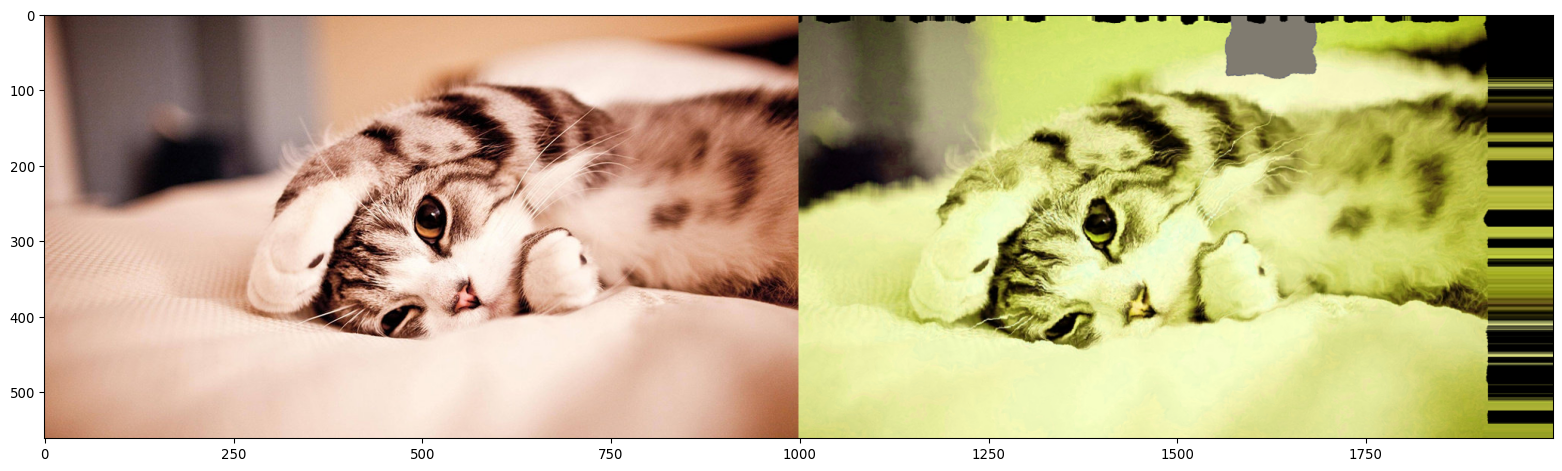
Image 1 (input shape: (225, 225, 3), output shape: (225, 225, 3))
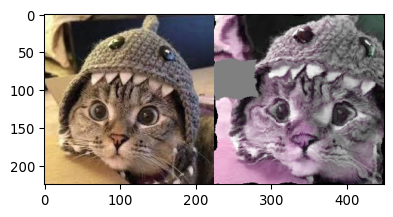