基本介绍
- 适配器模式(Adapter Pattern)将某个类的接口转换成客户端期望的另一个接口表示,主的目的是兼容性,让原本 因接口不匹配不能一起工作的两个类可以协同工作。其别名为包装器(Wrapper)
- 适配器模式属于结构型模式
- 主要分为三类:类适配器模式、对象适配器模式、接口适配器模式
工作原理
- 适配器模式:将一个类的接口转换成另一种接口.让原本接口不兼容的类可以兼容
- 从用户的角度看不到被适配者,是解耦的
- 用户调用适配器转化出来的目标接口方法,适配器再调用被适配者的相关接口方法
类适配器
例题:
众所周知我们生活常用电压是220V但是我们手机的规格电压是5V,所以我们就需要适配器去控制电压到5V,根据此过程编写程序:
UML类图:
电源类:Fu220
public class Fu220 {
public int outpu220V() {
int src = 220;
System.out.println("输入电压" + src + "V");
return src;
}
}
输出电源规则:Fu5接口
public interface Fu5 {
public int out5();
}
适配器:Adapter
public class Adapter implements Fu5 {
Fu220 f = null;
public Adapter(Fu220 f) {
super();
this.f = f;
}
@Override
public int out5() {
int src = f.outpu220V();
int d = src / 44;
System.out.println("适配器开始工作,转换后电压" + d + "V");
return d;
}
}
充电设备(Accpet类)
public class Accpet {
public void Charging(Adapter src) {
if (src.out5() == 5) {
System.out.println("满足要求,可以开始充电");
} else {
System.out.println("不能充电");
}
}
}
客户调用测试
public class Client {
public static void main(String[] args) {
System.out.println("===对象适配器模式===");
Accpet p = new Accpet();
p.Charging(new Adapter(new Fu220()));
}
}
注意事项和细节
- Java 是单继承机制,所以类适配器需要继承 src 类这一点算是一个缺点, 因为这要求 dst 必须是接口,有一定局 限性;
- src 类的方法在 Adapter 中都会暴露出来,也增加了使用的成本。
- 由于其继承了 src 类,所以它可以根据需求重写 src 类的方法,使得 Adapter 的灵活性增强了。
对象适配器模式
- 思路:将 Adapter 类作修改,不是继承A 类,而是持有A 类的实例,以解决 兼容性的问题
- 根据“合成复用原则”,在系统中尽量使用关联关系(聚合)来替代继承关系。
- UML类图:
Adapter类
public class Adapter implements Fu5 {
private Fu220 fu220; // 关联关系-聚合
// 通过构造器,传入一个 Fu220 实例
public Adapter(Fu220 fu220) {
this.fu220 = fu220;
}
@Override
public int output5V() {
int res = 0;
if (null != fu220) {
int src = fu220.output220V();
res = src / 44;
System.out.println("输出的电压为=" + res);
}
return res;
}
}
Accpet类
public class Accept {
//充电
public void charging(Fu5 fu) {
if(fu.output5V() == 5) {
System.out.println("电压为5V, 可以充电~~");
} else if (fu.output5V() > 5) {
System.out.println("电压大于5V, 不能充电~~");
}
}
}
Client类
public class Client {
public static void main(String[] args) {
// TODO Auto-generated method stub
System.out.println(" === 对象适配器模式 ====");
Accept phone = new Accept();
phone.charging(new Adapter(new Fu220()));
}
}
注意事项和细节
-
对象适配器和类适配器其实算是同一种思想,只不过实现方式不同。 根据合成复用原则,使用组合替代继承, 所以它解决了类适配器必须继承 src 的局限性问题。
-
使用成本更低,更灵活。
接口适配器模式
- 又叫缺省适配器模式。
- 核心思路:当不需要全部实现接口提供的方法时,可先设计一个抽象类实现接口,并为该接口中每个方法提供 一个默认实现(空方法),那么该抽象类的子类可有选择地覆盖父类的某些方法来实现需求
- 适用于一个接口不想使用其所有的方法的情况。
接口类
public interface Interface4 {
public void T1();
public void T2();
public void T3();
}
抽象方法默认实现类
扫描二维码关注公众号,回复:
11869177 查看本文章
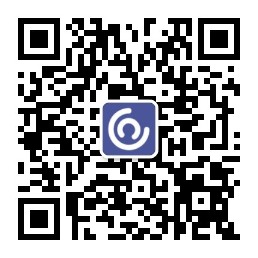
public abstract class AbsAdapter implements Interface4 {
//默认实现
public void T1() {
}
public void T2() {
}
public void T3() {
}
}
客户端调用
public class Client {
public static void main(String[] args) {
AbsAdapter absAdapter = new AbsAdapter() {
//只需要去实现覆盖我们 需要使用 接口方法
@Override
public void T1() {
// TODO Auto-generated method stub
System.out.println("使用了T1的方法");
}
};
absAdapter.T1();
}
}
应用场景(在 SpringMVC 框架应用)