互联网持久层框架Mybatis系列学习笔记
认识Mybatis的核心组件
1.1持久层的概念及Mybatis特点
持久层可以将业务数据转存到磁盘,在重启系统或者系统断电后,这些数据依然能被再次读取。一般执行持久层任务的都是数据库管理系统,持久层可以使用巨大的磁盘空间,缺点是,相对于内存数据,磁盘数据读写较慢。经常在网上见到的各种商家秒杀,又是怎么实现的呢?秒杀对数据读写要求高,所以出现了一种东西,名字叫Redis,它的存储介质是内存,可以高速读写。后面会有专题博文来分析Redis技术,这里说说Mybatis的三个优点:1. 不屏蔽原生SQL语句。优化SQL能显著提高数据的读写效率,对于复杂的业务,可以使用原生SQL语句,也可以调用编写好的存储过程。这一点,Hibernate是不支持原生SQL的。
2. Mybatis提供强大灵活的映射机制,方便Java开发者使用。这其实在说动态SQL功能,允许开发人员根据条件组装SQL。有了它,再也不怕产品经理经常改需求了。
3. Mybatis提供了基于Mapper的接口编程。一个接口对应一个XML配置,便能创建一个映射器。基于接口编程,对接Spring的IOC也会方便很多。
1.2准备Mybatis环境
笔者建议是下载Mybatis.jar包后,最好将源代码也拿到,现在附上一个官方Mybatis使用教程的PDF文档, [欢迎大家点击下载](https://download.csdn.net/download/qq_37040173/10757111)。对于刚入门的开发者来说,阅读源代码这事儿,先放一放吧,等能够熟练使用这个框架了,或许时机更合适。对于配置环境这件事儿,网上教程很多,DRY原则,呵呵。
1.3Mybatis核心组件介绍
Mybatis核心组件有四个:SqlSessionFactoryBuilder(构造器)/SqlSessionFactory(工厂接口)/SqlSession(会话)/SQL Mapper(映射器).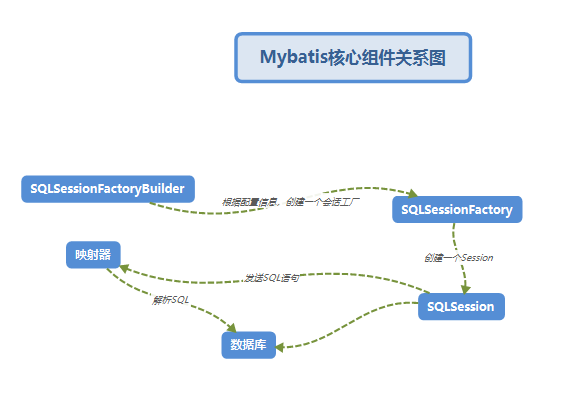
1.4SqlSessionFactory
使用Mybatis的第一步是创建一个SqlSessionFactory.首先是使用org.ibatis.session.Configuration类读取配置信息,然后使用“建造者模式”,由接口SqlSessionFactoryBuilder的build()方法创建一个SqlSessionFactory。需要声明的是,SqlSessionFactory是一个接口,不是类。它的默认实现类时DefaultSqlSessionFactory,这个是应用在单线程场景;SqlSessionManager是应用在多线程场景的
一般来说,可以读取XML配置文件,然后进行创建;也可以通过java代码,推荐使用XML方式。
14.1使用XML方式创建接口SqlSessionFactory
1.首先是基础配置文件,mybatis-config.xml,具体的每一个节点含义,请自行查阅。如下:<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<typeAliases>
<!-- AliasName -->
<typeAlias type="com.bert.chapter3.pojo.Role" alias="role"/>
</typeAliases>
<!-- Database Environment Configuration -->
<environments default="development">
<environment id="development">
<transactionManager type="JDBC" />
<dataSource type="POOLED">
<property name="driver" value="com.mysql.cj.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/mybatis?characterEncoding=utf8&serverTimezone=UTC" />
<property name="username" value="YourName" />
<property name="password" value="YourPassword" />
</dataSource>
</environment>
</environments>
<!-- MapperConfigs -->
<mappers>
<mapper resource="com/bert/chapter3/mapper/RoleMapper.xml"/>
</mappers>
</configuration>
2.POJO类,Role
public class Role {
private Long id;
private String roleName;
private String note;
/** getter and setter **/
public long getId() {
return this.id;
}
public void setId(long id) {
this.id = id;
}
public String getRoleName() {
return this.roleName;
}
public void setRoleName(String name) {
this.roleName = name;
}
public String getNote() {
return this.note;
}
}
3.RoleMapper接口定义
public interface RoleMapper {
public Role getRole(long id);
public int insertRole(Role role);
public int deleteRole(long id);
public int updateRole(long id);
public List<Role> findRoles(String roleName);
}
4.RoleMapper.xml配置文件
<?xml version="1.0" encoding="utf-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.bert.chapter3.mapper.RoleMapper">
<select id="getRole" parameterType="long" resultType="role">
select id,role_name as roleName,note from t_role where id = #{id}
</select>
<select id="findRoles" parameterType="String" resultType="role">
<bind name="pattern" value="'%' + roleName + '%'" />
select id,role_name as roleName,note from t_role
where role_name like #{pattern}
</select>
<insert id="insertRole" parameterType="role" >
insert into t_role(role_name,note) values(#{roleName},#{note})
</insert>
<delete id="deleteRole" parameterType="long" >
delete from t_role where id = #{id}
</delete>
<update id="updateRole" parameterType="long" >
update t_role set role_name=#{roleName},note=#{note} where id=#{id}
</update>
</mapper>
5.单例模式创建一个SqlSessionFactory和SqlSession(多线程)
import java.io.IOException;
import java.io.InputStream;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
public class SqlSessionFactoryUtil {
//1.Ensure the single pattern
private final static Class<SqlSessionFactoryUtil> LOCK = SqlSessionFactoryUtil.class;
//2.Ensure sessionFactory is the only instance of the SqlSessionFactory
private static SqlSessionFactory sessionFactory = null;
//3.the constructor is privatized.
private SqlSessionFactoryUtil() {
;
}
//4.A singleton approach to public disclosure
public static SqlSessionFactory getSqlSessionFactory() {
synchronized (LOCK) {
if(sessionFactory != null) {
return sessionFactory;
}
String resource = "mybatis-config.xml";
InputStream inputStream;
try {
inputStream = Resources.getResourceAsStream(resource);
//The Builder Pattern.
sessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
}catch(IOException e) {
e.printStackTrace();
return null;
}
return sessionFactory;
}
}
public static SqlSession openSqlSession() {
if(sessionFactory == null) {
getSqlSessionFactory();
}
return sessionFactory.openSession();
}
}
6.程序的入口Main类
public class Main {
public static void main(String [] args) {
Logger logger = Logger.getLogger(Main.class);
SqlSession sqlSession =null;
try {
sqlSession = SqlSessionFactoryUtil.openSqlSession();
RoleMapper roleMapper = sqlSession.getMapper(RoleMapper.class);
Role role = roleMapper.getRole(3L);
logger.error(role.getRoleName());
System.out.println("--------------");
System.out.println(role.getId()+"---"+ role.getRoleName()+"-----"+role.getNote());
sqlSession .commit();
}catch(Exception e) {
sqlSession.rollback();
e.printStackTrace();
}
finally {
if(sqlSession != null) {
sqlSession.close();
}
}
System.out.println("I am in love with your body.");
}
}
第一篇介绍就写到这里,可能会有后续篇。
说一下我写博客的感受吧。我觉得,这篇博客中的东西,没什么独特性,也不一定能为读者带来什么帮助。我写完之后,貌似也没有对什么概念理解得更加清楚,反之,我更像是一个搬运工,简单的把知识从书本搬运到了这个博客,关键是花费了我一个上午的时间来整理这个博客。所以,以后再写博客时,就不会像这样写了,,,,我又不是来写教程的,现在是自顾不暇状态,哪有时间和能力来分享我的学习经验啊。
相反的,什么东西是有用的呢?其实,我在写代码中间,会把遇到的错误Bug记录到一个记事本文件中,同样也会在旁边写下当时是怎么解决的。。。。这个记事本的记录,应该对大家和我都有用,而且内容也不多。咱们不能老是模仿着别人在写这些表面博客,做实事儿更重要。下一次再有闲心了,不如贴出来Bug集合。