AES ECB加密实现(java/php/python)
这里做个一个加密实现的记录,方便以后查找
AES加密 ECB模式 PKCS5填充 128位密码/密码块
ECB模式是将明文按照固定大小的块进行加密的,块大小不足则进行填充。ECB模式没有用到向量。
python 实现
# -*- coding=utf-8-*-
from Crypto.Cipher import AES
import os
from Crypto import Random
import base64
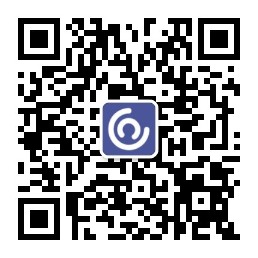
"""
aes加密算法
padding : PKCS5
"""
classAESUtil:
__BLOCK_SIZE_16 = BLOCK_SIZE_16 =AES.block_size
@staticmethod
defencryt(str, key):
cipher = AES.new(key, AES.MODE_ECB)
x = AESUtil.__BLOCK_SIZE_16 - (len(str)% AESUtil.__BLOCK_SIZE_16)
if x != 0:
str = str + chr(x)*x
msg = cipher.encrypt(str)
msg =base64.urlsafe_b64encode(msg).replace('=', '')
return msg
@staticmethod
defdecrypt(enStr, key):
cipher = AES.new(key, AES.MODE_ECB)
enStr += (len(enStr) % 4)*"="
decryptByts =base64.urlsafe_b64decode(enStr)
msg = cipher.decrypt(decryptByts)
paddingLen = ord(msg[len(msg)-1])
return msg[0:-paddingLen]
if __name__ == "__main__":
print AESUtil.encryt("512345", "1234567812345678")
print AESUtil.decrypt("1MbqzdK0IzP8vchDgRlzvw", "1234567812345678")
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
PHP实现
classAES {
var$key = "1234567812345678";
publicfunction__set($key, $value){
$this->$key = $value;
}
publicfunction__get($key) {
return$this->$key;
}
publicfunctionencrypt($input) {
$size = mcrypt_get_block_size(MCRYPT_RIJNDAEL_128,MCRYPT_MODE_ECB);
$input = $this->pkcs5_pad($input, $size);
$td =mcrypt_module_open(MCRYPT_RIJNDAEL_128, '', MCRYPT_MODE_ECB, '');
$iv = mcrypt_create_iv(mcrypt_enc_get_iv_size($td), MCRYPT_RAND);
mcrypt_generic_init($td, $this->key, $iv);
$data = mcrypt_generic($td, $input);
mcrypt_generic_deinit($td);
mcrypt_module_close($td);
$data = $this->base64url_encode($data);
return$data;
}
privatefunctionpkcs5_pad($text, $blocksize) {
$pad = $blocksize - (strlen($text) % $blocksize);
return$text . str_repeat(chr($pad), $pad);
}
functionstrToHex($string)
{
$hex="";
for ($i=0;$i<strlen($string);$i++)
$hex.=dechex(ord($string[$i]));
$hex=strtoupper($hex);
return $hex;
}
functionhexToStr($hex)
{
$string="";
for ($i=0;$i<strlen($hex)-1;$i+=2)
$string.=chr(hexdec($hex[$i].$hex[$i+1]));
return $string;
}
publicfunctiondecrypt($sStr) {
$decrypted= mcrypt_decrypt(
MCRYPT_RIJNDAEL_128,
//$sKey,
$this->key,
//base64_decode($sStr),
$this->base64url_decode($sStr),
//$sStr,
MCRYPT_MODE_ECB
);
$dec_s = strlen($decrypted);
$padding = ord($decrypted[$dec_s-1]);
$decrypted = substr($decrypted, 0, -$padding);
return$decrypted;
}
/**
*url 安全的base64编码 sunlonglong
*/
functionbase64url_encode($data) {
return rtrim(strtr(base64_encode($data), '+/', '-_'), '=');
}
/**
*url 安全的base64解码 sunlonglong
*/
functionbase64url_decode($data) {
return base64_decode(str_pad(strtr($data, '-_', '+/'), strlen($data) % 4, '=', STR_PAD_RIGHT));
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
使用示例
$aes = new AES();
//设置密钥
$aes->__set("key", "1234567812345678");
echo$aes->encrypt("123");
echo"\r\n";
echo$aes->decrypt("q7eZEivlY5ra7BUPzoF9vg");
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
java实现
publicclassSecureUtils {
publicstatic String decryptByAes(String sSrc, String reqKey) {
try {
byte[] raw;
if (reqKey != null) {
raw = reqKey[0].getBytes("ASCII");
} else {
raw = sKey.getBytes("ASCII");
}
SecretKeySpec skeySpec = new SecretKeySpec(raw, "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, skeySpec);
byte[] encrypted1 = decryptUrlSafe(sSrc);
try {
byte[] original =cipher.doFinal(encrypted1);
String originalString = new String(original);
return originalString;
} catch (Exception e) {
e.printStackTrace();
returnnull;
}
} catch (Exception ex) {
ex.printStackTrace();
returnnull;
}
}
publicstatic String encryptByAes(String sSrc, String...reqKey) throws Exception {
byte[] raw;
if (reqKey != null) {
raw = reqKey[0].getBytes("ASCII");
} else {
raw = sKey.getBytes("ASCII");
}
SecretKeySpec skeySpec = new SecretKeySpec(raw, "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE,skeySpec);
byte[] encrypted =cipher.doFinal(sSrc.getBytes());
return encryptUrlSalf(encrypted);
}
publicstaticbyte[] decryptUrlSafe(String key) throws Exception {
String decodeStr = key.replaceAll("-", "+").replaceAll("_", "/");
String qualsStr = "";
for (int i = 0; i < key.length() % 4; i++) {
qualsStr += "=";
}
return Base64.decode(decodeStr + qualsStr);
}
publicstatic String encryptUrlSalf(byte[] key) {
String str = Base64.encode(key);
str = str.replaceAll("\\+", "-").replaceAll("/", "_").replaceAll("=+$", "");
return str;
}
}