Math类是数学工具,它是一个final类 ,不能被继承,也不能被创建对象。它提供的所有属性和方法都是static修饰 ,直接使用类名.方法名/属性来调用。接下来就介绍常用的Math类的方法~
一. 取整函数
-
round():四舍五入
-
abs():取绝对值
-
取整方法:a. floor():将变量向下取整,也就是往数值小的方向取整
b. ceil():将变量向上取整,也就是往数值大的方向取整代码块如下:
......
double decimalPositive = 9.9;
// 注意下面的9.4999999999999999,取整之后会变成10。
// 这是因为9.4999999999999999超出了双精度数的精度范围,Java会先将其转为近似9.5的小数,然后再进行取整操作
// double decimalPositive = 9.4999999999999999;
long roundPositive = Math.round(decimalPositive); // 四舍五入
System.out.println("roundPositive=" + roundPositive);
double floorPositive = Math.floor(decimalPositive); // 往下取整,也就是往数值小的方向取整
System.out.println("floorPositive=" + floorPositive);
double ceilPositive = Math.ceil(decimalPositive); // 往上取整,也就是往数值大的方向取整
System.out.println("ceilPositive=" + ceilPositive);
double decimalNegative = -9.9;
long roundNegative = Math.round(decimalNegative); // 四舍五入
System.out.println("roundNegative=" + roundNegative);
double floorNegative = Math.floor(decimalNegative); // 往下取整,也就是往数值小的方向取整
System.out.println("floorNegative=" + floorNegative);
double ceilNegative = Math.ceil(decimalNegative); // 往上取整,也就是往数值大的方向取整
System.out.println("ceilNegative=" + ceilNegative);
double absoluteValue = Math.abs(decimalNegative); // 取绝对值
System.out.println("absoluteValue=" + absoluteValue);
运行结果:
- 计划任务
- 完成任务
二. 取随机数
- random方法只能生成小于1的随机小数(包括0和正小数),并不能生成随机整数。
- Random随机数工具包,可以解决random方法的问题:
import java.util.Random;
该工具实例化(new)后,nextInt 方法生成int类型的随机整数、nextLong 方法生成long类型的随机长整数、nextfloat 方法生成float类型的随机浮点小数、nextdouble 方法生成double类型的随机双精度小数。
(注:nextInt 和 nextLong 方法得到的随机整数可能是负数,而其他两个只能是正小数)
代码块如下:
......
double decimal = Math.random(); // 生成小于1的随机小数(包括0和正小数)
System.out.println("decimal=" + decimal);
int integer = new Random().nextInt(); // 生成随机整数(包括负数)
System.out.println("integer=" + integer);
long long_integer = new Random().nextLong(); // 生成随机长整数(包括负数)
System.out.println("long_integer=" + long_integer);
float float_decimal = new Random().nextFloat(); // 生成随机的浮点小数(不包括负数)
System.out.println("float_decimal=" + float_decimal);
double double_decimal = new Random().nextDouble(); // 生成随机的双精度小数(不包括负数)
System.out.println("double_decimal=" + double_decimal);
int hundred = new Random().nextInt(100); // 生成一百以内的随机整数(0≤随机整数<100,即包括0但不包括100)
System.out.println("hundred=" + hundred);
运行结果:
三、科学计算函数
- sqrt():开平方运算
- pow():求某数的n次方
- exp():求自然常数e的n次方
- log():求自然对数运算
- log10():求底数为10的对数
代码块如下:
扫描二维码关注公众号,回复:
12266983 查看本文章
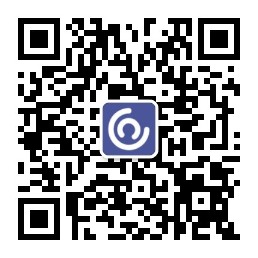
......
double nine = 9;
double sqrt = Math.sqrt(nine); // 开平方。对应数学符号√ ̄
System.out.println("sqrt=" + sqrt);
// 求n次方。pow的**第一个参数为幂运算的底数**,**第二个参数为幂运算的指数**
double pow = Math.pow(nine, 2);
System.out.println("pow=" + pow);
double five = 5;
double exp = Math.exp(five); // 求自然常数e的n次方
System.out.println("exp=" + exp);
double log = Math.log(exp); // 求自然对数,为exp方法的逆运算。对应数学函数lnN
System.out.println("log=" + log);
double log10 = Math.log10(100); // 求底数为10的对数。对应数学函数logN
System.out.println("log10=" + log10);
运行结果:
四、三角函数
Math库中三角函数方法是用 弧度的数值 (即:弧度 = 弧长/半径 =(角度/360)X 2πr/r = 角度 X π/180)。
代码块如下:
......
double angle = 60; // 三角函数的角度
// 弧度=该角度对应的弧长/半径。数学函数库Math专门提供了常量PI表示圆周率π的粗略值
double radian = angle * Math.PI / 180;
double sin = Math.sin(radian); // 求某弧度的正弦。求反正弦要调用asin方法
System.out.println("sin=" + sin);
double cos = Math.cos(radian); // 求某弧度的余弦。求反余弦要调用acos方法
System.out.println("cos=" + cos);
double tan = Math.tan(radian); // 求某弧度的正切。求反正切要调用atan方法
System.out.println("tan=" + tan);
// 求某弧度的余切。Math库未提供求余切值的方法,其实余切值就是正切值的倒数
double ctan = 1.0 / tan;
System.out.println("ctan=" + ctan);
运行结果: