链式编程思想
一般的程序
#include <iostream>
#include <string>
using namespace std;
class Person
{
private:
string name;
int age;
public:
void ShowInf()
{
cout << this->name << "的年龄为" << this->age << endl;
}
void Add10() // 每调用一次age加10
{
this->age += 10;
}
Person(string name, int age)
{
this->name = name;
this->age = age;
}
};
int main()
{
Person PersonObject("张三", 10);
PersonObject.Add10(); // +10
PersonObject.Add10(); // +10
PersonObject.ShowInf();
}
我们这样重复的调用Add10()函数来多次改变Person对象中age成员变量的值,有点太麻烦。
链式编程代码
其实就是使得函数返回值为*this,即对象本身,这样我们可以不断地对对象进行相同的操作,类似于”cout<<var<<var<<……<<endl;”的用法。
#include <iostream>
#include <string>
using namespace std;
class Person
{
private:
string name;
int age;
public:
void ShowInf()
{
cout << this->name << "的年龄为" << this->age << endl;
}
Person& Add10() // 每调用一次age加10
{
this->age += 10;
return *this;
}
Person(string name, int age)
{
this->name = name;
this->age = age;
}
};
int main()
{
Person PersonObject("张三", 10);
PersonObject.Add10().Add10(); // +10+10
PersonObject.ShowInf();
}
注意:我们这里一定要用”Person& Add10()”,函数返回值一定是类的引用,如果没有对象的引用会发生以下结果:
#include <iostream>
#include <string>
using namespace std;
class Person
{
private:
string name;
int age;
public:
void ShowInf()
{
cout << this->name << "的年龄为" << this->age << endl;
}
Person Add10() // 每调用一次age加10
{
this->age += 10;
return *this;
}
Person(string name, int age)
{
this->name = name;
this->age = age;
}
};
int main()
{
Person PersonObject("张三", 10);
PersonObject.Add10().Add10(); // 输出结果为20,并不是我们预期的30
PersonObject.ShowInf();
}
为什么呢?
因为链式编程思维,要求我们不断在原来的已经修改的基础上再进行修改,但是我们函数的返回值是Person类对象的值拷贝,因此当你再次用PersonObject.Add10()去调用Add10()成员函数时(PersonObject.Add10().Add10()),其实是“PersonObject.Add10()返回的新的临时变量,再在这个新的临时变量的基础上再调用Add10()函数”,最终我们的PersonObject对象仅仅调用了一次Add10()成员函数。
要想不改变上述函数的结构,依然让函数返回Person类对象而非引用,我们要对程序做一下修改:
#include <iostream>
#include <string>
using namespace std;
class Person
{
private:
string name;
int age;
public:
void ShowInf()
{
cout << this->name << "的年龄为" << this->age << endl;
}
Person Add10() // 每调用一次age加10
{
this->age += 10;
return *this;
}
Person(string name, int age)
{
this->name = name;
this->age = age;
}
};
int main()
{
Person PersonObject("张三", 10);
PersonObject = PersonObject.Add10();
PersonObject.Add10();
PersonObject.ShowInf();
}
我们再PersonObject.Add10()返回值的基础上再次调用Add10()函数才可以达到我们链式编程的结果。
扫描二维码关注公众号,回复:
12282276 查看本文章
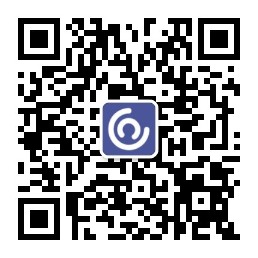