1.react
的生命周期
- 生命周期图
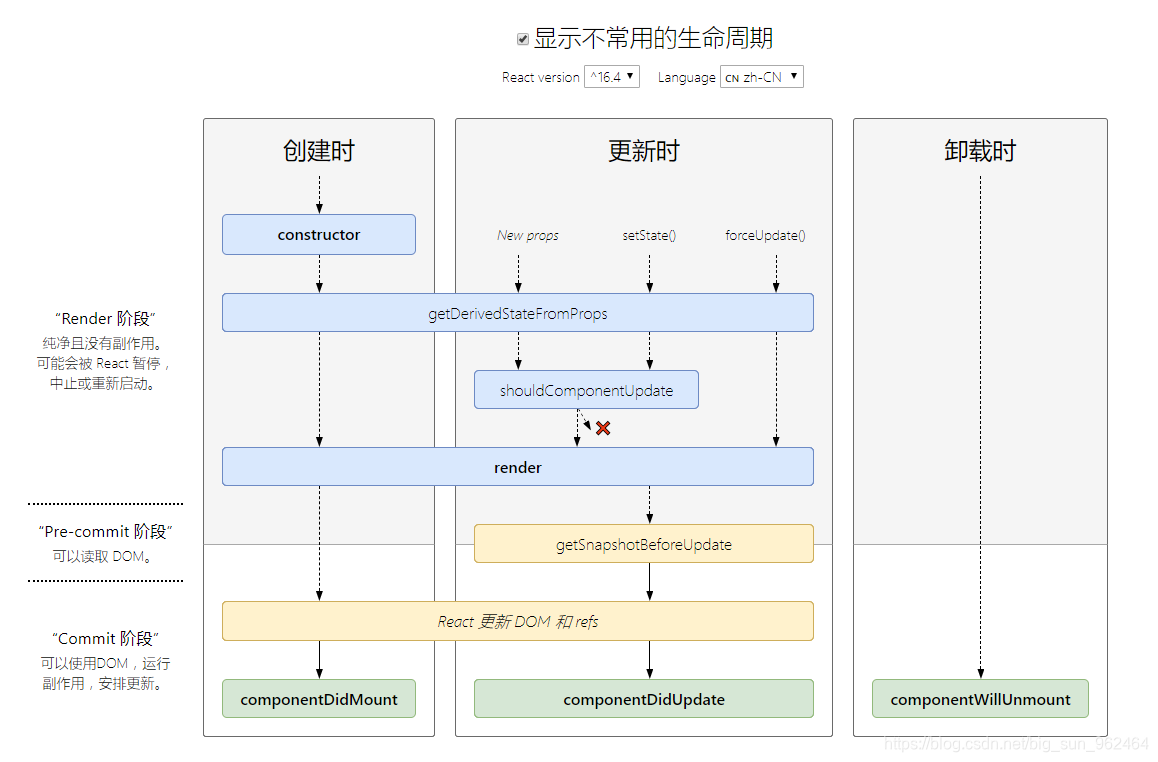
1.1react
的生命周期-类式组件
1.1.1生命周期基本使用
import React, {
Component } from 'react';
import ReactDOM from 'react-dom';
export default class Optimize extends Component {
constructor(props) {
super(props);
console.log('constructor');
this.state = {
msg: '这里是生命周期组件'
}
}
changeMsg = () => {
this.setState({
msg: '修改msg的数据'
})
}
strongUpdate = () => {
this.forceUpdate()
}
unmountCom = () => {
ReactDOM.unmountComponentAtNode(document.querySelector('#root'))
}
static getDerivedStateFromProps(props, state) {
console.log('getDerivedStateFromProps-获取派生状态从props中', props, state);
return null;
}
shouldComponentUpdate(props, state) {
console.log('shouldComponentUpdate-是否应该更新组件', props, state);
return true;
}
render() {
console.log('组件初始化/更新完毕', 'render');
return (
<div>
<h2>组件生命周期</h2>
<p>{
this.state.msg}</p>
<button onClick={
this.changeMsg}>更新msg</button>
<button onClick={
this.strongUpdate}>强制更新</button>
<button onClick={
this.unmountCom}>卸载组件</button>
</div>
)
}
getSnapshotBeforeUpdate(prevProps, prevState) {
console.log('getSnapshotBeforeUpdate-获取快照在更新之前', prevProps, prevState);
return {
name: '李四',
age: 16
}
}
componentDidMount() {
console.log('组件挂载完毕', 'componentDidMount');
}
componentDidUpdate(prevProps, prevState, snapshotValue) {
console.log('componentDidUpdate-组件更新完毕', prevProps, prevState, snapshotValue);
}
componentWillUnmount() {
console.log('componentWillUnmount------组件将要被卸载');
}
}
1.1.2生命周期-初始化
import React, {
Component } from 'react';
export default class Optimize extends Component {
constructor(props) {
super(props);
console.log('constructor');
this.state = {
msg: '这里是生命周期组件'
}
}
static getDerivedStateFromProps(props, state) {
console.log('getDerivedStateFromProps-获取派生状态从props中', props, state);
return null;
}
render() {
console.log('组件初始化/更新完毕', 'render');
return (
<div>
<h2>组件生命周期</h2>
<p>{
this.state.msg}</p>
</div>
)
}
componentDidMount() {
console.log('组件挂载完毕', 'componentDidMount');
}
}
1.1.3生命周期-更新
1.1.3.1生命周期-更新-正常更新
import React, {
Component } from 'react';
export default class Optimize extends Component {
state={
msg: '这里是生命周期组件'
}
changeMsg = () => {
this.setState({
msg: '修改msg的数据'
})
}
static getDerivedStateFromProps(props, state) {
console.log('getDerivedStateFromProps-获取派生状态从props中', props, state);
return null;
}
shouldComponentUpdate(props, state) {
console.log('shouldComponentUpdate-是否应该更新组件', props, state);
return true;
}
render() {
console.log('组件初始化/更新完毕', 'render');
return (
<div>
<h2>组件生命周期</h2>
<p>{
this.state.msg}</p>
<button onClick={
this.changeMsg}>更新msg</button>
</div>
)
}
getSnapshotBeforeUpdate(prevProps, prevState) {
console.log('getSnapshotBeforeUpdate-获取快照在更新之前', prevProps, prevState);
return {
name: '李四',
age: 16
}
}
componentDidUpdate(prevProps, prevState, snapshotValue) {
console.log('componentDidUpdate-组件更新完毕', prevProps, prevState, snapshotValue);
}
}
1.1.3.1生命周期-更新-强制更新
import React, {
Component } from 'react';
export default class Optimize extends Component {
strongUpdate = () => {
this.forceUpdate()
}
static getDerivedStateFromProps(props, state) {
console.log('getDerivedStateFromProps-获取派生状态从props中', props, state);
return null;
}
render() {
console.log('组件初始化/更新完毕', 'render');
return (
<div>
<h2>组件生命周期</h2>
<button onClick={
this.strongUpdate}>强制更新</button>
</div>
)
}
getSnapshotBeforeUpdate(prevProps, prevState) {
console.log('getSnapshotBeforeUpdate-获取快照在更新之前', prevProps, prevState);
return {
name: '李四',
age: 16
}
}
componentDidUpdate(prevProps, prevState, snapshotValue) {
console.log('componentDidUpdate-组件更新完毕', prevProps, prevState, snapshotValue);
}
}
1.1.4生命周期-卸载
import React, {
Component } from 'react';
import ReactDOM from 'react-dom';
export default class Optimize extends Component {
unmountCom = () => {
ReactDOM.unmountComponentAtNode(document.querySelector('#root'))
}
render() {
console.log('组件初始化/更新完毕', 'render');
return (
<div>
<h2>组件生命周期</h2>
<button onClick={
this.unmountCom}>卸载组件</button>
</div>
)
}
componentWillUnmount() {
console.log('componentWillUnmount------组件将要被卸载');
}
}
1.1.4getSnapshotBeforeUpdate/componentDidUpdate
使用实例
import React, {
Component } from 'react';
import ReactDOM from 'react-dom';
export default class Optimize extends Component {
constructor(props) {
super(props);
console.log('constructor');
this.state = {
msg: '这里是生命周期组件',
arr: []
}
}
addArrDate = () => {
let {
arr } = this.state;
arr.push({
id: arr.length + 1,
name: 'name' + (arr.length + 1)
});
this.setState({
arr
})
}
unmountCom = () => {
ReactDOM.unmountComponentAtNode(document.querySelector('#root'))
}
render() {
console.log('组件初始化/更新完毕', 'render');
return (
<div>
<h2>组件生命周期</h2>
<div ref={
(currentNode) => {
this.fatherWrapDOM = currentNode;
}} className="father-wrap">
{
this.state.arr.map(item => {
return (
<div key={
item.id} className="son-inner">
{
item.name}
</div>
)
})
}
</div>
<button onClick={
this.unmountCom}>卸载组件</button>
</div>
)
}
getSnapshotBeforeUpdate(prevProps, prevState) {
console.log('getSnapshotBeforeUpdate-获取快照在更新之前', prevProps, prevState);
let beforeUpdateWrapHeight = this.fatherWrapDOM.scrollHeight;
return {
beforeUpdateWrapHeight: beforeUpdateWrapHeight
}
}
componentDidMount() {
console.log('组件挂载完毕', 'componentDidMount');
this.timer = setInterval(() => {
this.addArrDate()
}, 1000);
}
componentDidUpdate(prevProps, prevState, snapshotValue) {
console.log('componentDidUpdate-组件更新完毕', prevProps, prevState, snapshotValue);
let {
beforeUpdateWrapHeight } = snapshotValue;
let nowWrapHeight = this.fatherWrapDOM.scrollHeight;
this.fatherWrapDOM.scrollTop += nowWrapHeight - beforeUpdateWrapHeight;
}
componentWillUnmount() {
console.log('componentWillUnmount------组件将要被卸载');
clearInterval(this.timer)
}
}
1.2react
的生命周期-函数式组件
1.2.1React.useEffect(()=>{})
- 如果不传React.useEffect的第二个参数,就代表着要监听所有的React.useState的状态,也就相当于类式组件中的
componentDidUpdate
- 注意点:这样写是十分危险的,如果在回调函数中不断更新数据,数据一更新,就要重新调用这个回到函数和更新界面,就会导致界面卡死的情况
import React, {
Component } from 'react';
export default function Optimize() {
const [count, changeCount] = React.useState(1)
function handleAddCount() {
changeCount((count) => {
return count + 1
})
}
React.useEffect(() => {
console.log('React.useReducer调用了');
setInterval(() => {
handleAddCount();
}, 1000);
})
return (
<div>
<h2>函数式组件生命周期</h2>
<p>{
count}</p>
</div>
)
}
1.2.2React.useEffect(()=>{},[])
- 空对象就是什么
React.useState
的状态都不监听,这个也就相当于类式组件中的componentDidMount
import React, {
Component } from 'react';
export default function Optimize() {
const [count, changeCount] = React.useState(1)
function handleAddCount() {
changeCount((count) => {
return count + 1
})
}
React.useEffect(() => {
console.log('React.useReducer调用了');
setInterval(() => {
handleAddCount();
}, 1000);
}, [])
return (
<div>
<h2>函数式组件生命周期</h2>
<p>{
count}</p>
</div>
)
}
1.2.3React.useEffect(()=>{},['React.useState()的值','React.useState()的值',...])
- 只会监听数组中的
React.useState()
的值,这个也就相当于类式组件中的componentDidUpdate
import React, {
Component } from 'react';
export default function Optimize() {
const [msg, changeMsg] = React.useState('初始msg')
const [count, changeCount] = React.useState(1)
function handleChangeMsg() {
changeMsg('修改msg')
}
function handleAddCount() {
changeCount((count) => {
return count + 1
})
}
React.useEffect(() => {
console.log('React.useReducer调用了');
setInterval(() => {
handleAddCount();
}, 1000);
}, [msg])
return (
<div>
<h2>函数式组件生命周期</h2>
<p>{
msg}</p>
<p>{
count}</p>
<button onClick={
handleChangeMsg}>改变msg</button>
</div>
)
}
1.2.4React.useEffect(()=>{return ()=>{}})
- 如果在
React.useEffect
的回调函数中返回了一个回调函数,那么这个回调函数中的内容就会在组件将要卸载的时候触发也就相当于类式组件中的componentWillUnmount
import React, {
Component } from 'react';
import ReactDOM from 'react-dom';
export default function Optimize() {
const [msg, changeMsg] = React.useState('初始msg')
const [count, changeCount] = React.useState(1)
function handleChangeMsg() {
changeMsg('修改msg')
}
function handleAddCount() {
changeCount((count) => {
return count + 1
})
}
function unmount() {
ReactDOM.unmountComponentAtNode(document.querySelector('#root'));
}
React.useEffect(() => {
console.log('React.useReducer调用了');
let timer = setInterval(() => {
handleAddCount();
}, 1000);
return ()=>{
console.log('组件将要卸载');
clearInterval(timer);
}
}, [msg])
return (
<div>
<h2>函数式组件生命周期</h2>
<p>{
msg}</p>
<p>{
count}</p>
<button onClick={
handleChangeMsg}>改变msg</button>
<button onClick={
unmount}>卸载组件</button>
</div>
)
}