两栏布局
先写一下样式
<div class="box">
<div class="left">左</div>
<div class="right">右</div>
</div>
使用float + margin方式实现
<style>
div {
height: 200px;
color: red;
}
.left {
float: left;
width: 300px;
background-color: green;
}
.right {
width: 100%;
margin-left: 300px;
background-color: black;
}
</style>
还可以使用position实现
div {
height: 200px;
color:red;
}
.left {
position: absolute;
left: 0;
width: 300px;
background-color: green;
}
.right {
width: 100%;
margin-left: 300px;
background-color: black;
}
也可以使用flex实现
div {
height: 200px;
color: red;
}
.box {
display: flex;
}
.left {
flex: 0 0 300px;
background-color: green;
}
.right {
flex: 1 1;
background-color: black;
}
三栏布局
先写样式
<div class="box">
<div class="left">左</div>
<div class="right">右</div>
<div class="center">中</div>
</div>
使用float + margin方式实现
div {
height: 200px;
color: red;
}
.center {
width: 100%;
margin-left: 300px;
margin-right: 100px;
background-color: green;
}
.left {
float: left;
width: 300px;
background-color: black;
}
.right {
float: right;
width: 100px;
background-color: blue;
}
使用position实现
div {
height: 200px;
color: red;
}
.center {
width: 100%;
margin-left: 300px;
margin-right: 100px;
background-color: black;
}
.left {
position: absolute;
left: 0px;
width: 300px;
background-color: green;
}
.right {
position: absolute;
right: 0px;
width: 100px;
background-color: blue;
}
使用flex布局
.box{
display:flex;
}
.left{
background-color: red;
width: 100px;
height: 100px;
flex:0 1 auto;
order:-1;
}
.right{
background-color: blue;
width: 100px;
height: 100px;
flex:0 1 auto;
}
.center{
background-color:yellow;
flex:1 0 auto;
height: 100px;
}
圣杯布局
三列布局,左列和右列都是固定宽度,中间自适应
<html>
2 <head>
3 <meta charset="utf-8">
4 <style type="text/css">
5 .main{
6 width: 80%;
7 height: 300px;
8 }
9 .left,.right,.middle{
10 float: left;
11 height: 100%;
12 }
13 .middle{
14 width: 100%;
15 background-color: gold;
16 }
17 .left{
18 width: 150px;
19 margin-left: -100%;
20 background-color: yellow;
21 }
22 .right{
23 width: 200px;
24 margin-left: -200px;
25 background-color: #ee4311;
26 }
27 .content{
28 margin:0 200px 0 150px;
29 }
30 </style>
31 </head>
32 <body>
33 <div class="main">
34
35 <div class="middle">
36 <div class="content">
37 middle-content
38 </div>
39 </div>
40 <div class="left">left</div>
41
42 <div class="right">right</div>
43 </div>
44 </body>
45 </html>
双飞翼布局
三栏布局,两边的盒子宽度固定,中间盒子自适应
<style>
body {
min-width: 550px;
font-weight: bold;
font-size: 20px;
}
#header,
#footer {
background: rgba(29, 27, 27, 0.726);
text-align: center;
height: 60px;
line-height: 60px;
}
#container {
overflow: hidden;
}
.column {
text-align: center;
height: 300px;
line-height: 300px;
}
#left, #right, #center {
float: left;
}
#center {
width: 100%;
background: rgb(206, 201, 201);
}
#left {
width: 200px;
margin-left: -100%;
background: rgba(95, 179, 235, 0.972);
}
#right {
width: 150px;
margin-left: -150px;
background: rgb(231, 105, 2);
}
.content {
margin: 0 150px 0 200px;
}
</style>
<body>
<div id="header">#header</div>
<div id="container">
<div id="center" class="column">
<div class="content">#center</div>
</div>
<div id="left" class="column">#left</div>
<div id="right" class="column">#right</div>
</div>
<div id="footer">#footer</div>
</body>
双飞翼布局和圣杯布局的区别:
圣杯布局和双飞翼布局解决问题的方案在前一半是相同的,也就是三栏全部float浮动,但左右两栏加上负margin让其跟中间栏div并排,以形成三栏布局。不同的地方在于解决中间div内容不被遮挡的思路上面:
扫描二维码关注公众号,回复:
12498967 查看本文章
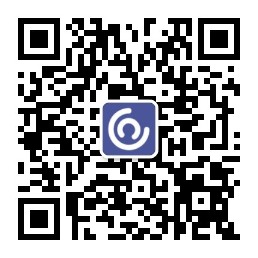
1.圣杯布局的为了中间内容不被修改,是通过包裹元素的padding-left和padding-right来使得内容div置于中间,然后再通过相对定位position:relative,配合right或left属性让左右两栏不遮挡中间内容。
2.双飞翼布局的解决方案是:通过在中间元素的内部新增一个div用于放置内容,然后通过左右外边距margin-left和margin-right为左右两栏留出位置。
垂直居中
position + margin实现
<body>
<div class="box">
<div class="box1"></div>
</div>
</body>
<style>
.box {
position: relative;
width: 500px;
height: 500px;
background-color: red;
}
.box1 {
position: absolute;
left: 50%;
top: 50%;
width: 200px;
height: 200px;
margin-left: -100px;
margin-top: -100px;
background-color: black;
}
</style>
flex实现
<body>
<div class="box">
<div class="box1"></div>
</div>
</body>
<style>
.box {
display: flex;
align-items: center;
justify-content: center;
width: 500px;
height: 500px;
background-color: blue;
}
.box1 {
width: 200px;
height: 200px;
background-color: black;
}
</style>
水平居中
通过text-align: center 实现;
前提是看它的父元素是不是块级元素,如果是,则直接给父元素设置 text-align: center;
<body>
<div class="box">
<span class="box1">1111</span>
</div>
</body>
<style>
.box {
width: 500px;
height: 300px;
background-color: skyblue;
text-align: center;
}
.box1{
width: 100px;
height: 100px;
}
</style>
如果不是,则先将其父元素设置为块级元素,再给父元素设置 text-align: center;
.box {
display: block;
width: 500px;
height: 300px;
background-color: skyblue;
text-align: center;
}
.box1{
width: 100px;
height: 100px;
}
可以使用margin: 0 auto实现
#father {
width: 500px;
height: 300px;
background-color: skyblue;
}
#son {
width: 100px;
height: 100px;
background-color: green;
margin: 0 auto;
还可以使用定位实现
.box {
width: 500px;
height: 300px;
background-color: skyblue;
position: relative;
}
.box1 {
width: 100px;
height: 100px;
background-color: green;
position: absolute;
left: 50%;
margin-left: -50px;
}
使用flexbox布局
.box {
width: 500px;
height: 300px;
background-color: skyblue;
display: flex;
justify-content: center;
}
.box1 {
width: 100px;
height: 100px;
background-color: green;
}