异常这个概念不用说,我们都知道是个什么意思,在程序运行时,许多情况都会导致异常事件的发生,比如说:
(1)想打开的文件不存在
(2)网络连接中断
(3)操作数超出预定范围
(4)访问的数据打不开
(5)正在装载的类文件丢失等等
简单的说,Java代码运行时期发生的问题就是异常。在Java中,有非常完备的机制来处理异常,它把异常信息封装成了一个类,当出现了问题时,就会创建异常类对象并在控制台输出异常的相关信息。
例如:
程序的下标越界时,会输出相关的异常信息。
package demo1;
public class demo {
public static void main(String[] args) {
int[] arr = {
1,2,3};
System.out.println(arr[4]);
}
}
1.抛出异常(throw)与方法声明异常(throws)
java中,提供了一个throw关键字,用来抛出一个指定的异常对象。
步骤:
(1).创建一个异常对象。(封装一些提示信息。注:提示信息可以自己写)
(2).通过throw关键字将异常对象告知给使用者。
使用格式:
//throw用在方法内,抛出一个异常对象,将这个异常对象传递到调用者处,并结束当前方法的执行。
//格式:throw new 异常类名(参数);
例如:throw new NullPointerExction("要访问的arr数组不存在!");
例如下面的代码,假如不做任何处理,便会出现空指针的异常。
package demo1;
public class demo {
public static void main(String[] args) {
int[] arr = null;
int i = getarray(arr);
System.out.println(i);
}
public static int getarray(int[] arr) {
int i = arr[arr.length-1];
return i*2;
}
}
```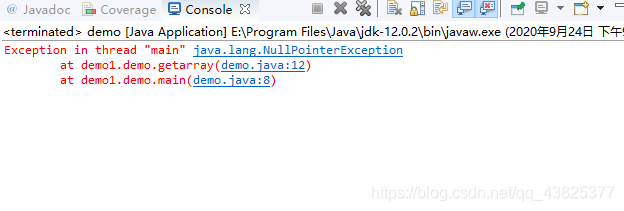
此时如果我们给程序加上throw语句,程序便会报错,不能运行
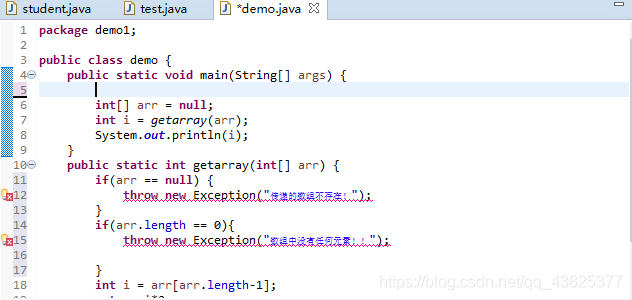
这是因为没有在方法中声明异常,就相当于一个人生病了,但是他没有表现出来,也告诉别人,所以没人知道他生病了一样。此时我们就需要用到另一个关键字throws,它的作用是用在方法声明上,标明此方法可能出现异常,请调用者处理。声明异常后,程序便可以正常运行了,需要注意的是,当在方法后使用throws关键字后,在main函数后面也要使用throws关键字进行异常声明,不然程序会报错。
```java
package demo1;
public class demo {
public static void main(String[] args) throws Exception{
int[] arr = null;
int i = getarray(arr);
System.out.println(i);
}
public static int getarray(int[] arr) throws Exception{
if(arr == null) {
throw new Exception("传递的数组不存在!");
}
if(arr.length == 0){
throw new Exception("数组中没有任何元素!!");
}
int i = arr[arr.length-1];
return i*2;
}
}
现在程序打印出来的异常信息就是我们在throw new Exeption();中写的异常信息。
2.try…cath…finally
上面只是异常处理的一种方法,Java中还可以使用try…cath…finally来进行异常处理。
格式:
try{
被检测的代码
可能出现异常的代码
}catch(异常类的名称 变量){
异常处理方式
循环,判断,调用方法,变量
}finally{
必须要执行的代码
}
例子:
package demo1;
public class demo {
public static void main(String[] args){
int[] arr = null;
try {
int i = getarray(arr);
System.out.println(i);
}catch(NullPointerException ex) {
System.out.println(ex);
}
}
public static int getarray(int[] arr) throws NullPointerException{
if(arr == null) {
throw new NullPointerException("传递的数组不存在!");
}
if(arr.length == 0){
throw new NullPointerException("数组中没有任何元素!!");
}
int i = arr[arr.length-1];
return i*2;
}
}
可以看到,使用这种方法我们不需要在方法的后面使用throws关键字,上面的例子中我们并没有是使用finally代码块的内容。finally在try…cath…finally结构中是可选择的,在这里面的语句无论try语句块中是否存在异常,finally语句中块都会被执行,因此finally语句块最大的作用就是用于资源的释放,防止资源的泄露。
注:finally语句块中的语句只在一种情况下不会执行(在捕获到异常后在catch语句块中以Sytem.exit(0)语句结束虚拟机)