10.1 项目目录
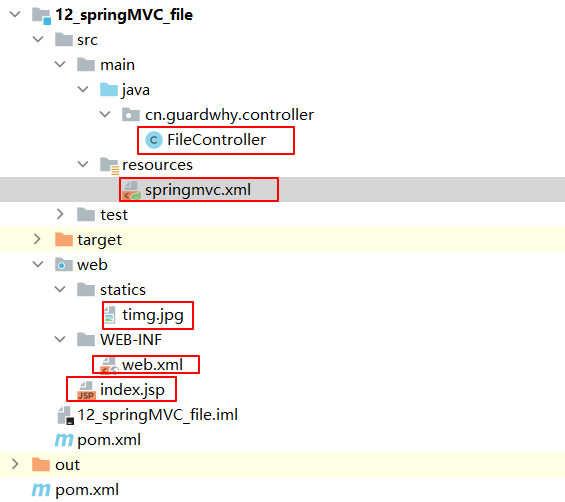
10.2 导入相关依赖
<!--导入文件上传-->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.3</version>
</dependency>
<!--servlet-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
</dependency>
10.3 配置springmvc.xml
<!--文件上传配置-->
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<!-- 请求的编码格式,必须和jSP的pageEncoding属性一致,以便正确读取表单的内容,默认为ISO-8859-1 -->
<property name="defaultEncoding" value="utf-8"/>
<!-- 上传文件大小上限,单位为字节(10485760=10M) -->
<property name="maxUploadSize" value="10485760"/>
<property name="maxInMemorySize" value="40960"/>
</bean>
CommonsMultipartFile 的 常用方法:
String getOriginalFilename():获取上传文件的原名
InputStream getInputStream():获取文件流
void transferTo(File dest):将上传文件保存到一个目录文件中
10.4 前端页面
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>下载与上传文件</title>
</head>
<body>
<%--上传--%>
<form action="${pageContext.request.contextPath}/upload2" enctype="multipart/form-data" method="post">
<input type="file" name="file"/>
<input type="submit" value="upload">
</form>
<%--下载图片--%>
<a href="${pageContext.request.contextPath}/statics/timg.jpg">下载图片</a>
</body>
</html>
10.5控制器(Controller)
package cn.guardwhy.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.commons.CommonsMultipartFile;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.URLEncoder;
@RestController
public class FileController {
/***
* 上传文件(方式1)
* @param file
* @param request
* @return
* @throws IOException
*/
@RequestMapping("/upload")
public String fileUpload(@RequestParam("file") CommonsMultipartFile file,
HttpServletRequest request) throws IOException {
//1.获取文件名 : file.getOriginalFilename();
String uploadFileName = file.getOriginalFilename();
//2.如果文件名为空,直接回到首页
if ("".equals(uploadFileName)) {
return "redirect:/index.jsp";
}
System.out.println("上传文件名 : " + uploadFileName);
//3. 上传路径保存设置
String path = request.getServletContext().getRealPath("/upload");
//4. 如果路径不存在,创建一个
File realPath = new File(path);
if (!realPath.exists()) {
realPath.mkdir();
}
System.out.println("上传文件保存地址:" + realPath);
//5. 文件输入流
InputStream is = file.getInputStream();
//6. 文件输出流
OutputStream os = new FileOutputStream(new File(realPath, uploadFileName));
//7. 读取写出
int len = 0;
byte[] buffer = new byte[1024];
while ((len = is.read(buffer)) != -1) {
os.write(buffer, 0, len);
os.flush();
}
// 8.关闭资源
os.close();
is.close();
// 9.重定向到index页面
return "redirect:/index.jsp";
}
/***
* 采用file.Transto 来保存上传的文件
* @param file
* @param request
* @return
* @throws IOException
*/
@RequestMapping("/upload2")
public String fileUpload2(@RequestParam("file") CommonsMultipartFile file,
HttpServletRequest request) throws IOException {
//1.上传路径保存设置
String path = request.getServletContext().getRealPath("/upload");
File realPath = new File(path);
if (!realPath.exists()) {
realPath.mkdir();
}
//2. 上传文件地址
System.out.println("上传文件保存地址:" + realPath);
//3. 通过CommonsMultipartFile的方法直接写文件
file.transferTo(new File(realPath + "/" + file.getOriginalFilename()));
// 4.重定向到index页面
return "redirect:/index.jsp";
}
/***
* 下载资源
* @param response
* @param request
* @return
* @throws Exception
*/
@RequestMapping(value = "/download")
public String downloads(HttpServletResponse response,
HttpServletRequest request) throws Exception {
//1. 要下载的图片地址
String path = request.getServletContext().getRealPath("/upload");
String fileName = "timg.jpg";
//2.设置response 响应头
response.reset();
//3.字符编码
response.setCharacterEncoding("UTF-8");
//4.二进制传输数据
response.setContentType("multipart/form-data");
//5.设置响应头
response.setHeader("Content-Disposition", "attachment;fileName=" + URLEncoder.encode(fileName, "UTF-8"));
File file = new File(path, fileName);
//6.读取文件--输入流
InputStream input = new FileInputStream(file);
//7. 写出文件--输出流
OutputStream out = response.getOutputStream();
byte[] buff = new byte[1024];
int index = 0;
//8. 执行 写出操作
while ((index = input.read(buff)) != -1) {
out.write(buff, 0, index);
out.flush();
}
// 9.关闭资源
out.close();
input.close();
return "ok";
}
}
10.6 执行结果