String是javascript中的一个重要的复杂数据类型。我们在学习的过程中会经常用到,这里我总结了学习过程中常用的一些方法和属性。
1.字符串的常用属性:
// constructor:展示字符串的构造函数。
var str = "hello,world";
console.log(str.constructor); // f String() { native code }
// length: 字符串的长度。
var str = "hello,world";
console.log(str.length); // 11
// prototype:添加字符串对象的属性。
var str = "hello,world";
String.prototype.a = 1;
console.log(str.a); // 1
2.字符串的常用方法:
1.位置查看方法:
// indexOf: 查看指定字符在字符串中第一个的下标位置
var str = "hello,world";
let i = str.indexOf('l');
console.log(i); // 2
// 返回值为下标位置,找不到返回-1
// lastIndexOf: 查看指定字符在字符串中最后一个的下标位置
var str = "hello,world";
let i = str.indexOf('l');
console.log(i); // 3
// 返回值为下标位置,找不到返回-1
2.字符串截取:
// slice:截取需要的字符串
var str = "hello,world";
let newStr = str.slice(1,8);
console.log(newStr); // ello,wo
// 返回值为:字符串,找不到返回空字符串
// slice()可以给一个参数,表示从什么地方开始截取
// 也可以给两个参数,表示从开始位置,截取到结束位置,结束位置不包括在内
3.字符串去空格:
// trim:去除字符串前后无意义的空格
var str = " hel lo,wor ld ";
let newStr = str.trim();
console.log(newStr); // hel lo,wor ld
// 返回值为新字符串,不会改变原字符串
4.字符串分割:
// split:按指定的字符对字符串进行分割
var str = "hel lo,wor ld";
let newStr = str.split(',');
console.log(newStr); // ['hel lo','wor ld']
// 返回值为数组,找不到返回['hel lo,wor ld']
5.字符串替换:
// replace:对满足条件的第一个字符串进行替换
var str = "hel lo,wor ld";
let newStr = str.replace('l', 'aa');
console.log(newStr); // heaa lo,wor ld
// 返回值为新的字符串,找不到返回原字符串
6.匹配正则表达式:
// match:可以匹配正则表达式,和字符串
var str = "hello,world";
let newStr = str.match(/l/g);
console.log(newStr); // ["l", "l", "l"]
// 返回值为数组,找不到返回null
var str = "hello,world";
let newStr = str.match('l');
console.log(newStr); // ["l", index: 2, input: "hello,world", groups: undefined]
// 返回值为数组,找不到返回null
7.获取字符对应的ASCII码:
// charCodeAt:获取一个字符的ASCII码
var str = "h";
let newStr = str.charCodeAt();
console.log(newStr); // 104
// 返回值为一个整型,若是个字符串,只会返回第一个字符的ASCII码
// 空字符串返回NaN
8.字符串指定位置的值:
// charAt():字符串指定位置的字符
var str = "hello,world";
let newStr = str.charAt(2);
console.log(newStr); // l
// 返回值为字符,找不到返回空字符
9.字符串大小写转换:
// toLocaleLowerCase:按本地方式将字符串转换成小写
var str = "Hello,World";
let newStr = str.toLocaleLowerCase(22);
console.log(newStr); // hello,world
// 返回值为字符串
// toLocaleUpperCase:按本地方式将字符串转换成大写
var str = "Hello,World";
let newStr = str.toLocaleLowerCase(22);
console.log(newStr); // HELLO,WORLD
// 返回值为字符串
// toLowerCase:把字符串转换成小写
var str = "Hello,World";
let newStr = str.toLocaleLowerCase(22);
console.log(newStr); // hello,world
// 返回值为字符串
// toUpperCase:把字符串转换成大写
var str = "Hello,World";
let newStr = str.toLocaleLowerCase(22);
console.log(newStr); // HELLO,WORLD
// 返回值为字符串
10.一些数据类型共有的方法:
扫描二维码关注公众号,回复:
12699872 查看本文章
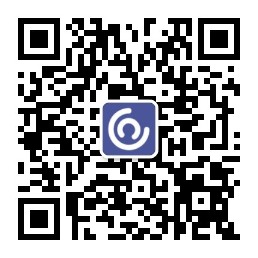
// valueOf:返回某个字符串对象的原始值
// toString:将对象转换成字符串
var str = "Hello,World";
let str1 = str.valueOf();
let str2 = str.toString();
console.log(str1); // Hello,World
console.log(str2); // Hello,World
以上代码就是在js中我们经常使用的字符串的方法和属性,当然还有很多字符串的方法和属性没有提及,等到要用到时,会及时学习更新。