SMT32时钟系统常用寄存器:
Clock control register (RCC_CR)
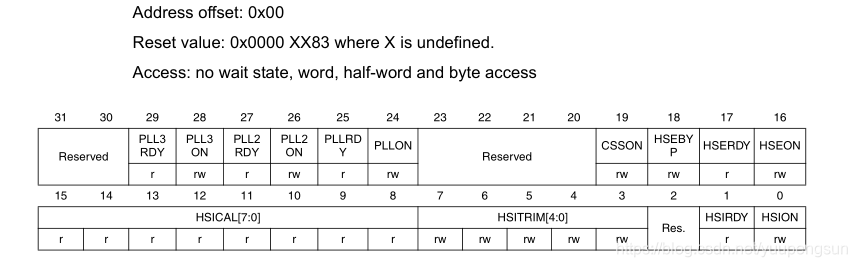
Clock configuration register (RCC_CFGR)
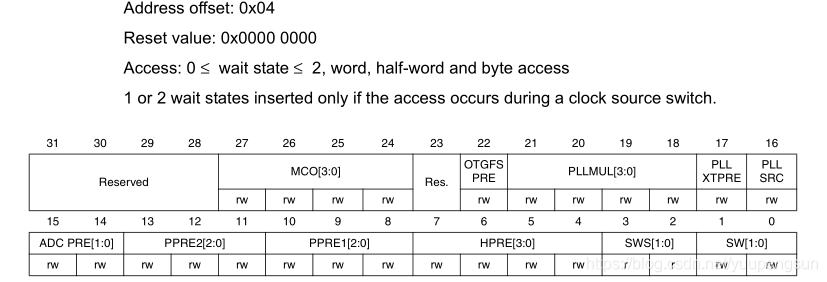
Clock interrupt register (RCC_CIR)
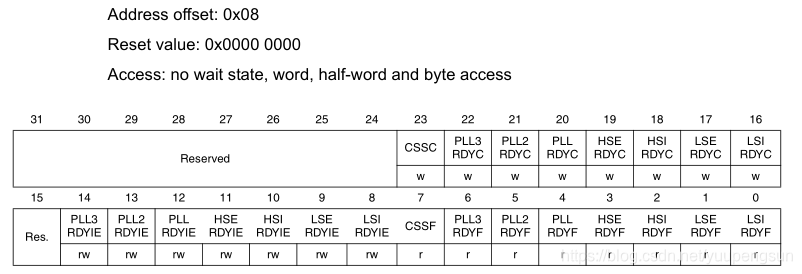
APB2 peripheral reset register (RCC_APB2RSTR)
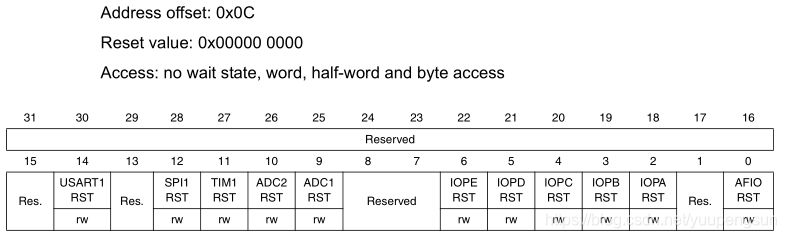
APB1 peripheral reset register (RCC_APB1RSTR)
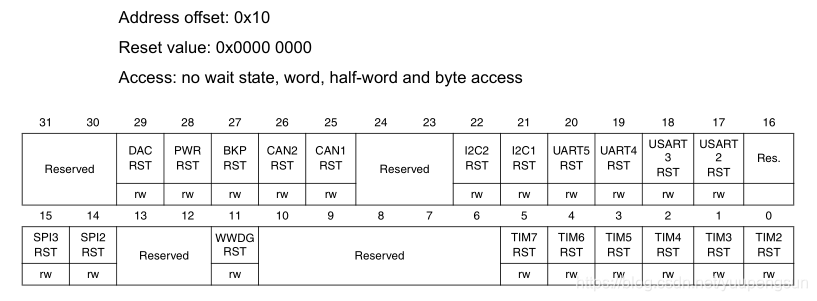
AHB Peripheral Clock enable register (RCC_AHBENR)
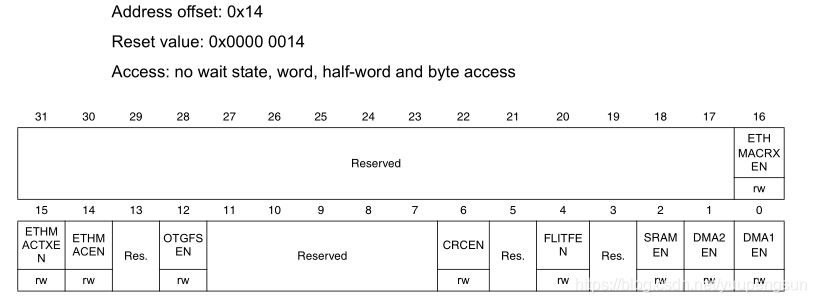
APB2 peripheral clock enable register (RCC_APB2ENR)
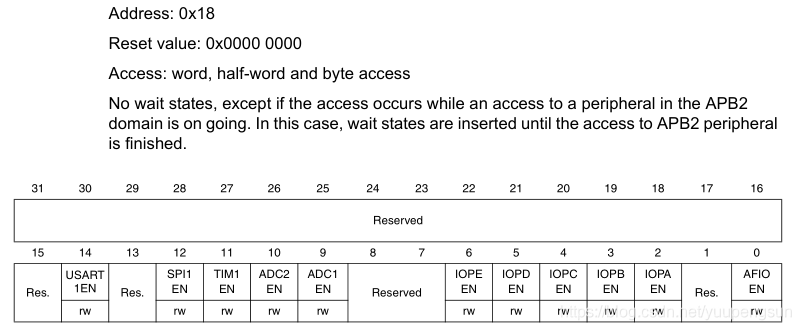
APB1 peripheral clock enable register (RCC_APB1ENR)
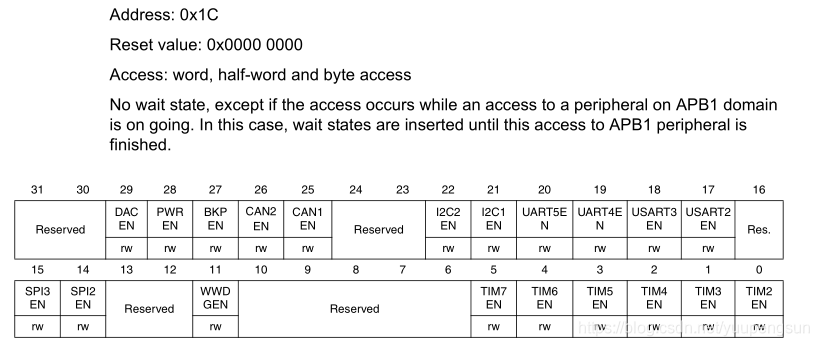
Control/status register (RCC_CSR)
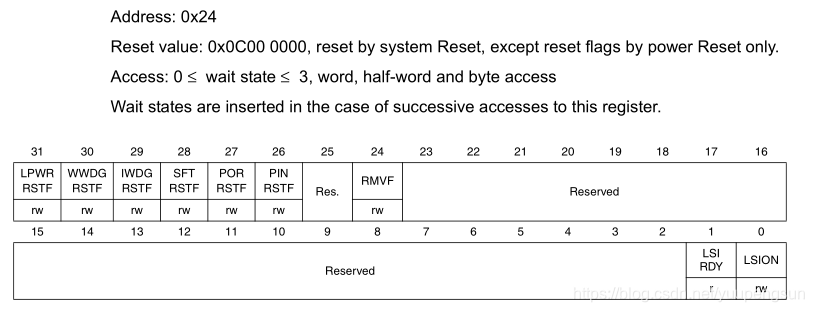
相关官方库文件
寄存器结构体定义:file name: stm32f10x.h
/*file name: stm32f10x.h*/
/*
* @brief Reset and Clock Control
*/
typedef struct
{
/*重要:Clock control register HSI、HSE、CSS、PLL等使能和就绪*/
__IO uint32_t CR;
/*重要:Clock configuration register PLL等时钟源的选择,和分频或者倍频系数设定*/
__IO uint32_t CFGR;
/*Clock interrupt register 清除/使能 时钟就绪中断*/
__IO uint32_t CIR;
/*APB2 peripheral reset register APB2线上外设复位寄存器*/
__IO uint32_t APB2RSTR;
/*APB1 peripheral reset register APB1线上外设复位寄存器*/
__IO uint32_t APB1RSTR;
/*重要:AHB Peripheral Clock enable register DMA,SDIO等时钟使能*/
__IO uint32_t AHBENR;
/*重要:APB2 peripheral clock enable register APB2线上外设I/O时钟使能*/
__IO uint32_t APB2ENR;
/*重要:APB1 peripheral clock enable register APB1线上外设TIM3EN/CAN/I2C/UART/SPI时钟使能*/
__IO uint32_t APB1ENR;
/*Backup domain control register 备份域控制寄存器*/
__IO uint32_t BDCR;
/*Control/status register 控制状态寄存器*/
__IO uint32_t CSR;
#ifdef STM32F10X_CL
/*AHB peripheral clock reset register (RCC_AHBRSTR)AHB外设时钟复位寄存器(RCC_AHBRSTR)*/
__IO uint32_t AHBRSTR;
/*Clock configuration register2 */
__IO uint32_t CFGR2;
#endif /* STM32F10X_CL */
#if defined (STM32F10X_LD_VL) || defined (STM32F10X_MD_VL) || defined (STM32F10X_HD_VL)
uint32_t RESERVED0;
/*Clock configuration register2 */
__IO uint32_t CFGR2;
#endif /* STM32F10X_LD_VL || STM32F10X_MD_VL || STM32F10X_HD_VL */
} RCC_TypeDef;
寄存器常用操作:file name: stm32f10x_rcc.c
重要:外设时钟使能
/**
* @brief Enables or disables the AHB peripheral clock.
* @param RCC_AHBPeriph: specifies the AHB peripheral to gates its clock.
*
* For @b STM32_Connectivity_line_devices, this parameter can be any combination
* of the following values:
* @arg RCC_AHBPeriph_DMA1
* @arg RCC_AHBPeriph_DMA2
* @arg RCC_AHBPeriph_SRAM
* @arg RCC_AHBPeriph_FLITF
* @arg RCC_AHBPeriph_CRC
* @arg RCC_AHBPeriph_OTG_FS
* @arg RCC_AHBPeriph_ETH_MAC
* @arg RCC_AHBPeriph_ETH_MAC_Tx
* @arg RCC_AHBPeriph_ETH_MAC_Rx
*
* For @b other_STM32_devices, this parameter can be any combination of the
* following values:
* @arg RCC_AHBPeriph_DMA1
* @arg RCC_AHBPeriph_DMA2
* @arg RCC_AHBPeriph_SRAM
* @arg RCC_AHBPeriph_FLITF
* @arg RCC_AHBPeriph_CRC
* @arg RCC_AHBPeriph_FSMC
* @arg RCC_AHBPeriph_SDIO
*
* @note SRAM and FLITF clock can be disabled only during sleep mode.
* @param NewState: new state of the specified peripheral clock.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_AHBPeriphClockCmd(uint32_t RCC_AHBPeriph, FunctionalState NewState)
/**
* @brief Enables or disables the High Speed APB (APB2) peripheral clock.
* @param RCC_APB2Periph: specifies the APB2 peripheral to gates its clock.
* This parameter can be any combination of the following values:
* @arg RCC_APB2Periph_AFIO, RCC_APB2Periph_GPIOA, RCC_APB2Periph_GPIOB,
* RCC_APB2Periph_GPIOC, RCC_APB2Periph_GPIOD, RCC_APB2Periph_GPIOE,
* RCC_APB2Periph_GPIOF, RCC_APB2Periph_GPIOG, RCC_APB2Periph_ADC1,
* RCC_APB2Periph_ADC2, RCC_APB2Periph_TIM1, RCC_APB2Periph_SPI1,
* RCC_APB2Periph_TIM8, RCC_APB2Periph_USART1, RCC_APB2Periph_ADC3,
* RCC_APB2Periph_TIM15, RCC_APB2Periph_TIM16, RCC_APB2Periph_TIM17,
* RCC_APB2Periph_TIM9, RCC_APB2Periph_TIM10, RCC_APB2Periph_TIM11
* @param NewState: new state of the specified peripheral clock.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_APB2PeriphClockCmd(uint32_t RCC_APB2Periph, FunctionalState NewState)
/**
* @brief Enables or disables the Low Speed APB (APB1) peripheral clock.
* @param RCC_APB1Periph: specifies the APB1 peripheral to gates its clock.
* This parameter can be any combination of the following values:
* @arg RCC_APB1Periph_TIM2, RCC_APB1Periph_TIM3, RCC_APB1Periph_TIM4,
* RCC_APB1Periph_TIM5, RCC_APB1Periph_TIM6, RCC_APB1Periph_TIM7,
* RCC_APB1Periph_WWDG, RCC_APB1Periph_SPI2, RCC_APB1Periph_SPI3,
* RCC_APB1Periph_USART2, RCC_APB1Periph_USART3, RCC_APB1Periph_USART4,
* RCC_APB1Periph_USART5, RCC_APB1Periph_I2C1, RCC_APB1Periph_I2C2,
* RCC_APB1Periph_USB, RCC_APB1Periph_CAN1, RCC_APB1Periph_BKP,
* RCC_APB1Periph_PWR, RCC_APB1Periph_DAC, RCC_APB1Periph_CEC,
* RCC_APB1Periph_TIM12, RCC_APB1Periph_TIM13, RCC_APB1Periph_TIM14
* @param NewState: new state of the specified peripheral clock.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_APB1PeriphClockCmd(uint32_t RCC_APB1Periph, FunctionalState NewState)
时钟使能:
/**
* @brief Configures the External High Speed oscillator (HSE).
* @note HSE can not be stopped if it is used directly or through the PLL as system clock.
* @param RCC_HSE: specifies the new state of the HSE.
* This parameter can be one of the following values:
* @arg RCC_HSE_OFF: HSE oscillator OFF
* @arg RCC_HSE_ON: HSE oscillator ON
* @arg RCC_HSE_Bypass: HSE oscillator bypassed with external clock
* @retval None
*/
void RCC_HSEConfig(uint32_t RCC_HSE)
/**
* @brief Enables or disables the Internal High Speed oscillator (HSI).
* @note HSI can not be stopped if it is used directly or through the PLL as system clock.
* @param NewState: new state of the HSI. This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_HSICmd(FunctionalState NewState)
/**
* @brief Enables or disables the PLL.
* @note The PLL can not be disabled if it is used as system clock.
* @param NewState: new state of the PLL. This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_PLLCmd(FunctionalState NewState)
/**
* @brief Enables or disables the PLL2.
* @note
* - The PLL2 can not be disabled if it is used indirectly as system clock
* (i.e. it is used as PLL clock entry that is used as System clock).
* - This function applies only to STM32 Connectivity line devices.
* @param NewState: new state of the PLL2. This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_PLL2Cmd(FunctionalState NewState)
/**
* @brief Enables or disables the PLL3.
* @note This function applies only to STM32 Connectivity line devices.
* @param NewState: new state of the PLL3. This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_PLL3Cmd(FunctionalState NewState)
/**
* @brief Configures the External Low Speed oscillator (LSE).
* @param RCC_LSE: specifies the new state of the LSE.
* This parameter can be one of the following values:
* @arg RCC_LSE_OFF: LSE oscillator OFF
* @arg RCC_LSE_ON: LSE oscillator ON
* @arg RCC_LSE_Bypass: LSE oscillator bypassed with external clock
* @retval None
*/
void RCC_LSEConfig(uint8_t RCC_LSE)
/**
* @brief Enables or disables the Internal Low Speed oscillator (LSI).
* @note LSI can not be disabled if the IWDG is running.
* @param NewState: new state of the LSI. This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_LSICmd(FunctionalState NewState)
/**
* @brief Enables or disables the RTC clock.
* @note This function must be used only after the RTC clock was selected using the RCC_RTCCLKConfig function.
* @param NewState: new state of the RTC clock. This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_RTCCLKCmd(FunctionalState NewState)
时钟源相关
/**
* @brief Configures the system clock (SYSCLK).
* @param RCC_SYSCLKSource: specifies the clock source used as system clock.
* This parameter can be one of the following values:
* @arg RCC_SYSCLKSource_HSI: HSI selected as system clock
* @arg RCC_SYSCLKSource_HSE: HSE selected as system clock
* @arg RCC_SYSCLKSource_PLLCLK: PLL selected as system clock
* @retval None
*/
void RCC_SYSCLKConfig(uint32_t RCC_SYSCLKSource)
/**
* @brief Configures the PLL clock source and multiplication factor.
* @note This function must be used only when the PLL is disabled.
* @param RCC_PLLSource: specifies the PLL entry clock source.
* For @b STM32_Connectivity_line_devices or @b STM32_Value_line_devices,
* this parameter can be one of the following values:
* @arg RCC_PLLSource_HSI_Div2: HSI oscillator clock divided by 2 selected as PLL clock entry
* @arg RCC_PLLSource_PREDIV1: PREDIV1 clock selected as PLL clock entry
* For @b other_STM32_devices, this parameter can be one of the following values:
* @arg RCC_PLLSource_HSI_Div2: HSI oscillator clock divided by 2 selected as PLL clock entry
* @arg RCC_PLLSource_HSE_Div1: HSE oscillator clock selected as PLL clock entry
* @arg RCC_PLLSource_HSE_Div2: HSE oscillator clock divided by 2 selected as PLL clock entry
* @param RCC_PLLMul: specifies the PLL multiplication factor.
* For @b STM32_Connectivity_line_devices, this parameter can be RCC_PLLMul_x where x:{[4,9], 6_5}
* For @b other_STM32_devices, this parameter can be RCC_PLLMul_x where x:[2,16]
* @retval None
*/
void RCC_PLLConfig(uint32_t RCC_PLLSource, uint32_t RCC_PLLMul)
/**
* @brief Configures the PLL2 multiplication factor.
* @note
* - This function must be used only when the PLL2 is disabled.
* - This function applies only to STM32 Connectivity line devices.
* @param RCC_PLL2Mul: specifies the PLL2 multiplication factor.
* This parameter can be RCC_PLL2Mul_x where x:{[8,14], 16, 20}
* @retval None
*/
void RCC_PLL2Config(uint32_t RCC_PLL2Mul)
/**
* @brief Configures the PLL3 multiplication factor.
* @note
* - This function must be used only when the PLL3 is disabled.
* - This function applies only to STM32 Connectivity line devices.
* @param RCC_PLL3Mul: specifies the PLL3 multiplication factor.
* This parameter can be RCC_PLL3Mul_x where x:{[8,14], 16, 20}
* @retval None
*/
void RCC_PLL3Config(uint32_t RCC_PLL3Mul)
状态参数获取
/**
* @brief Returns the clock source used as system clock.
* @param None
* @retval The clock source used as system clock. The returned value can
* be one of the following:
* - 0x00: HSI used as system clock
* - 0x04: HSE used as system clock
* - 0x08: PLL used as system clock
*/
uint8_t RCC_GetSYSCLKSource(void)
/**
* @brief Returns the frequencies of different on chip clocks.
* @param RCC_Clocks: pointer to a RCC_ClocksTypeDef structure which will hold
* the clocks frequencies.
* @note The result of this function could be not correct when using
* fractional value for HSE crystal.
* @retval None
*/
void RCC_GetClocksFreq(RCC_ClocksTypeDef* RCC_Clocks)
/**
* @brief Checks whether the specified RCC flag is set or not.
* @param RCC_FLAG: specifies the flag to check.
*
* For @b STM32_Connectivity_line_devices, this parameter can be one of the
* following values:
* @arg RCC_FLAG_HSIRDY: HSI oscillator clock ready
* @arg RCC_FLAG_HSERDY: HSE oscillator clock ready
* @arg RCC_FLAG_PLLRDY: PLL clock ready
* @arg RCC_FLAG_PLL2RDY: PLL2 clock ready
* @arg RCC_FLAG_PLL3RDY: PLL3 clock ready
* @arg RCC_FLAG_LSERDY: LSE oscillator clock ready
* @arg RCC_FLAG_LSIRDY: LSI oscillator clock ready
* @arg RCC_FLAG_PINRST: Pin reset
* @arg RCC_FLAG_PORRST: POR/PDR reset
* @arg RCC_FLAG_SFTRST: Software reset
* @arg RCC_FLAG_IWDGRST: Independent Watchdog reset
* @arg RCC_FLAG_WWDGRST: Window Watchdog reset
* @arg RCC_FLAG_LPWRRST: Low Power reset
*
* For @b other_STM32_devices, this parameter can be one of the following values:
* @arg RCC_FLAG_HSIRDY: HSI oscillator clock ready
* @arg RCC_FLAG_HSERDY: HSE oscillator clock ready
* @arg RCC_FLAG_PLLRDY: PLL clock ready
* @arg RCC_FLAG_LSERDY: LSE oscillator clock ready
* @arg RCC_FLAG_LSIRDY: LSI oscillator clock ready
* @arg RCC_FLAG_PINRST: Pin reset
* @arg RCC_FLAG_PORRST: POR/PDR reset
* @arg RCC_FLAG_SFTRST: Software reset
* @arg RCC_FLAG_IWDGRST: Independent Watchdog reset
* @arg RCC_FLAG_WWDGRST: Window Watchdog reset
* @arg RCC_FLAG_LPWRRST: Low Power reset
*
* @retval The new state of RCC_FLAG (SET or RESET).
*/
FlagStatus RCC_GetFlagStatus(uint8_t RCC_FLAG)
分频系数选择配置
/**
* @brief Configures the AHB clock (HCLK).
* @param RCC_SYSCLK: defines the AHB clock divider. This clock is derived from
* the system clock (SYSCLK).
* This parameter can be one of the following values:
* @arg RCC_SYSCLK_Div1: AHB clock = SYSCLK
* @arg RCC_SYSCLK_Div2: AHB clock = SYSCLK/2
* @arg RCC_SYSCLK_Div4: AHB clock = SYSCLK/4
* @arg RCC_SYSCLK_Div8: AHB clock = SYSCLK/8
* @arg RCC_SYSCLK_Div16: AHB clock = SYSCLK/16
* @arg RCC_SYSCLK_Div64: AHB clock = SYSCLK/64
* @arg RCC_SYSCLK_Div128: AHB clock = SYSCLK/128
* @arg RCC_SYSCLK_Div256: AHB clock = SYSCLK/256
* @arg RCC_SYSCLK_Div512: AHB clock = SYSCLK/512
* @retval None
*/
void RCC_HCLKConfig(uint32_t RCC_SYSCLK)
/**
* @brief Configures the Low Speed APB clock (PCLK1).
* @param RCC_HCLK: defines the APB1 clock divider. This clock is derived from
* the AHB clock (HCLK).
* This parameter can be one of the following values:
* @arg RCC_HCLK_Div1: APB1 clock = HCLK
* @arg RCC_HCLK_Div2: APB1 clock = HCLK/2
* @arg RCC_HCLK_Div4: APB1 clock = HCLK/4
* @arg RCC_HCLK_Div8: APB1 clock = HCLK/8
* @arg RCC_HCLK_Div16: APB1 clock = HCLK/16
* @retval None
*/
void RCC_PCLK1Config(uint32_t RCC_HCLK)
/**
* @brief Configures the High Speed APB clock (PCLK2).
* @param RCC_HCLK: defines the APB2 clock divider. This clock is derived from
* the AHB clock (HCLK).
* This parameter can be one of the following values:
* @arg RCC_HCLK_Div1: APB2 clock = HCLK
* @arg RCC_HCLK_Div2: APB2 clock = HCLK/2
* @arg RCC_HCLK_Div4: APB2 clock = HCLK/4
* @arg RCC_HCLK_Div8: APB2 clock = HCLK/8
* @arg RCC_HCLK_Div16: APB2 clock = HCLK/16
* @retval None
*/
void RCC_PCLK2Config(uint32_t RCC_HCLK)
RCC的中断相关
/**
* @brief Enables or disables the specified RCC interrupts.
* @param RCC_IT: specifies the RCC interrupt sources to be enabled or disabled.
*
* For @b STM32_Connectivity_line_devices, this parameter can be any combination
* of the following values
* @arg RCC_IT_LSIRDY: LSI ready interrupt
* @arg RCC_IT_LSERDY: LSE ready interrupt
* @arg RCC_IT_HSIRDY: HSI ready interrupt
* @arg RCC_IT_HSERDY: HSE ready interrupt
* @arg RCC_IT_PLLRDY: PLL ready interrupt
* @arg RCC_IT_PLL2RDY: PLL2 ready interrupt
* @arg RCC_IT_PLL3RDY: PLL3 ready interrupt
*
* For @b other_STM32_devices, this parameter can be any combination of the
* following values
* @arg RCC_IT_LSIRDY: LSI ready interrupt
* @arg RCC_IT_LSERDY: LSE ready interrupt
* @arg RCC_IT_HSIRDY: HSI ready interrupt
* @arg RCC_IT_HSERDY: HSE ready interrupt
* @arg RCC_IT_PLLRDY: PLL ready interrupt
*
* @param NewState: new state of the specified RCC interrupts.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void RCC_ITConfig(uint8_t RCC_IT, FunctionalState NewState)
外设时钟相关
/**
* @brief Configures the USB clock (USBCLK).
* @param RCC_USBCLKSource: specifies the USB clock source. This clock is
* derived from the PLL output.
* This parameter can be one of the following values:
* @arg RCC_USBCLKSource_PLLCLK_1Div5: PLL clock divided by 1,5 selected as USB
* clock source
* @arg RCC_USBCLKSource_PLLCLK_Div1: PLL clock selected as USB clock source
* @retval None
*/
void RCC_USBCLKConfig(uint32_t RCC_USBCLKSource)
/**
* @brief Configures the ADC clock (ADCCLK).
* @param RCC_PCLK2: defines the ADC clock divider. This clock is derived from
* the APB2 clock (PCLK2).
* This parameter can be one of the following values:
* @arg RCC_PCLK2_Div2: ADC clock = PCLK2/2
* @arg RCC_PCLK2_Div4: ADC clock = PCLK2/4
* @arg RCC_PCLK2_Div6: ADC clock = PCLK2/6
* @arg RCC_PCLK2_Div8: ADC clock = PCLK2/8
* @retval None
*/
void RCC_ADCCLKConfig(uint32_t RCC_PCLK2)
/**
* @brief Configures the I2S2 clock source(I2S2CLK).
* @note
* - This function must be called before enabling I2S2 APB clock.
* - This function applies only to STM32 Connectivity line devices.
* @param RCC_I2S2CLKSource: specifies the I2S2 clock source.
* This parameter can be one of the following values:
* @arg RCC_I2S2CLKSource_SYSCLK: system clock selected as I2S2 clock entry
* @arg RCC_I2S2CLKSource_PLL3_VCO: PLL3 VCO clock selected as I2S2 clock entry
* @retval None
*/
void RCC_I2S2CLKConfig(uint32_t RCC_I2S2CLKSource)
/**
* @brief Configures the RTC clock (RTCCLK).
* @note Once the RTC clock is selected it can't be changed unless the Backup domain is reset.
* @param RCC_RTCCLKSource: specifies the RTC clock source.
* This parameter can be one of the following values:
* @arg RCC_RTCCLKSource_LSE: LSE selected as RTC clock
* @arg RCC_RTCCLKSource_LSI: LSI selected as RTC clock
* @arg RCC_RTCCLKSource_HSE_Div128: HSE clock divided by 128 selected as RTC clock
* @retval None
*/
void RCC_RTCCLKConfig(uint32_t RCC_RTCCLKSource)