文章目录
1 控制反转
控制反转(Inversion of Control,缩写为IoC),是[面向对象编程中的一种设计原则,可以用来减低计算机代码之间的耦合度。其中最常见的方式叫做依赖注入(Dependency Injection,简称DI),还有一种方式叫“依赖查找”(Dependency Lookup)。通过控制反转,对象在被创建的时候,由一个调控系统内所有对象的外界实体将其所依赖的对象的引用传递给它。也可以说,依赖被注入到对象中。
在Spring中,使用控制反转是为了降低类与类之间的耦合度。Spring使用IoC容器来对管理相对于的资源,我们将所有Java bean类的控制权交给Spring,由它来实现类的创建。如果我们需要切换不同的实现类,如持久层Dao类或者事务层Service类时,只需要进行少量的配置代码即可,真正做到降低耦合度。
2 常用类
2.1 ApplicationContext和BeanFactory
-
ApplicationContext: 它在构建核心容器时创建对象,采取的策略是采用立即加载的方式。也就是说,只要一读取完配置文件马上就创建配置文件中配置的对象。
-
BeanFactory:它在构建核心容器时采用延迟加载的方式。也就是说,什么时候需要获取对象了,才会真正创建对象。
2.2 ApplicationContext三个实现类
- ClassPathXmlApplicationContext:可以加载类路径下的配置文件,要求配置文件必须在类路径下;
- FileSystemXmlApplicationContext:可以加载磁盘任意路径下的配置文件(必须有访问权限);
- AnnotationConfigApplicationContext:用于读取注解创建容器的
2.3 简单案例
public static void main(String[] args) {
//1.获取配置文件,创建Spring核心容器对象
ApplicationContext ac = new ClassPathXmlApplicationContext("Spring.xml");
//2.根据id获取Bean对象
IAccountService as = (IAccountService)ac.getBean("accountService");
IAccountDao adao = (IAccountDao) ac.getBean("accountDao");
// 3.查看对象是否创建成功
System.out.println(as);
System.out.println(adao);
// 4.查看依赖注入是否成功
as.saveAccount();
}
3 配置bean的方式
在Spring配置文件中配置bean通常有三种方式:使用构造函数、使用工厂的普通方法、使用工厂的静态方法。
第一种方式:使用构造函数创建。在spring的配置文件中使用bean标签,配以id和class属性之后,且没有其他属性和标签时。采用的就是默认构造函数创建bean对象,此时如果类中没有默认构造函数,则对象无法创建。或者可以使用依赖注入的方式,使用有参构造函数对bean进行创建。
<bean id="accountService" class="service.impl.AccountServiceImpl">
<!--此时默认使用AccountServiceImpl的无参构造函数进行对象的创建 -->
</bean>
第二种方式: 使用工厂中的普通方法创建对象(即使用某个类中的方法创建对象,并存入spring容器)
<bean id="instanceFactory" class="factory.InstanceFactory">
<!--配置一个工厂,其中提供一个普通方法getAccountService,该方法返回accountService的实现类 -->
</bean>
<bean id="accountService" factory-bean="instanceFactory" factory-method= "getAccountService">
<!--使用工厂instanceFactory中的方法getAccountService -->
</bean>
第三种方式:使用工厂中的静态方法创建对象(使用某个类中的静态方法创建对象,并存入spring容器)
<bean id="accountService" class="factory.StaticFactory" factory-method="getAccountService">
<!--使用工厂StaticFactory中的静态方法getAccountService -->
</bean>
4 bean的作用范围和生命周期
4.1 作用范围
bean的作用范围包括五种,可以使用bean标签的scope属性进行指定:
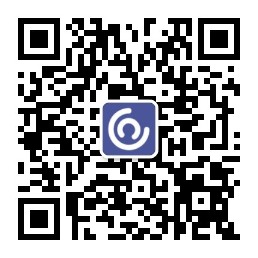
- singleton:单例的(默认值)
- prototype:多例的
- request:作用于web应用的请求范围
- session:作用于web应用的会话范围
- global-session:作用于集群环境的会话范围(全局会话范围),当不是集群环境时,它就是session
<bean id="accountService" class="service.impl.AccountServiceImpl" scope="prototype">
</bean>
4.2 生命周期
单例对象:
- 出生:当容器创建时对象出生;
- 活着:只要容器还在,对象一直活着;
- 死亡:容器销毁,对象消亡;
- 总结:单例对象的生命周期和容器相同。
多例对象:
- 出生:当我们使用对象时spring框架为我们创建;
- 活着:对象只要是在使用过程中就一直活着;
- 死亡:当对象长时间不用,且没有别的对象引用时,由Java的垃圾回收器回收。
<bean id="accountService" class="service.impl.AccountServiceImpl" scope="prototype" init-method="init" destroy-method="destroy">
<!--可以指定init和destroy方法 -->
</bean>
5 依赖注入
在xml文件中配置依赖注入有两种方式:使用构造函数和set方法
5.1 构造函数注入法
构造函数注入(类必须提供对应的构造方法):
- 标签:constructor-arg
- 标签出现的位置:bean标签的内部
- 标签中的属性(常用的是name + value或者 name + ref)
- type:用于指定要注入的数据的数据类型,该数据类型也是构造函数中某个或某些参数的类型
- index:用于指定要注入的数据给构造函数中指定索引位置的参数赋值。索引的位置是从0开始
- name:用于指定给构造函数中指定名称的参数赋值
- value:用于提供基本类型和String类型的数据
- ref:用于指定其他的bean类型数据,该类型必须在IoC容器配置了
<bean id="person" class="domain.Person">
<constructor-arg name="name" value="Tom"></constructor-arg>
<constructor-arg name="age" value="18"></constructor-arg>
<constructor-arg name="birthday" ref="birth"></constructor-arg>
</bean>
<!-- 配置一个日期对象 -->
<bean id="birth" class="java.util.Date">
<!--配置一个日期对象,可以供给person对象注入 -->
</bean>
5.2 使用P标签注入
P标签注入(类必须提供对应的set方法):
在xml文件上部要添加P标签依赖:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="student" class="domain.Student" p:name="小明" p:age="23">
</bean>
5.3 set方法注入
5.3.1 普通类型
set方法注入(类必须提供对应的set方法):
- 标签:property
- 位置:bean标签的内部
- 属性:
- name:用于指定注入时所调用的set方法名称
- value:用于提供基本类型和String类型的数据
- ref:用于指定其他的bean类型数据,该类型必须在IoC容器配置了
<bean id="person" class="domain.Person">
<property name="name" value="Tom" ></property>
<property name="age" value="21"></property>
<property name="birthday" ref="birth"></property>
</bean>
<!-- 配置一个日期对象 -->
<bean id="birth" class="java.util.Date">
<!--配置一个日期对象,可以供给person对象注入 -->
</bean>
5.3.2 复杂类型
<bean id="complex" class="domain.Complex">
<property name="myStrs">
<!--注入一个数组 -->
<set>
<value>AAA</value>
<value>BBB</value>
<value>CCC</value>
</set>
</property>
<property name="myList">
<!--注入一个list -->
<array>
<value>AAA</value>
<value>BBB</value>
<value>CCC</value>
</array>
</property>
<property name="mySet">
<!--注入一个Set -->
<list>
<value>AAA</value>
<value>BBB</value>
<value>CCC</value>
</list>
</property>
<property name="myMap">
<!--注入一个map -->
<props>
<prop key="testC">ccc</prop>
<prop key="testD">ddd</prop>
</props>
</property>
<property name="myProps">
<!--注入一个属性集properties -->
<map>
<entry key="testA" value="aaa"></entry>
<entry key="testB">
<value>BBB</value>
</entry>
</map>
</property>
</bean>