springboot整合Dubbo和Zookeeper
1、Zookeeper的安装
1. Zookeeper概述
ZooKeeper 是一种分布式协调服务,用于管理大型主机。在分布式环境中协调和管理服务是一个复杂的过程。ZooKeeper 通过其简单的架构和 API 解决了这个问题。ZooKeeper 允许开发人员专注于核心应用程序逻辑,而不必担心应用程序的分布式特性。
ZooKeeper 框架最初是在“Yahoo!"上构建的,用于以简单而稳健的方式访问他们的应用程序。 后来,Apache ZooKeeper 成为 Hadoop,HBase 和其他分布式框架使用的有组织服务的标准。 例如,Apache HBase 使用 ZooKeeper 跟踪分布式数据的状态。
(更多信息:https://www.w3cschool.cn/zookeeper/zookeeper_overview.html)
2. Zookeeper的安装与启动
去官网:https://archive.apache.org/dist/zookeeper/
下载对应的安装包,我选择zookeeper-3.3.6版本。其他高版本,如zookeeper-3.5.8、zookeeper-3.6.2在Windows 10可能无法启动(亲测),不服可以试试。原因我没有去探究。
下载完成后,在zookeeper-3.3.6\conf\目录下 把 zoo_sample.cfg 改为 zoo.cfg
然后在zookeeper-3.3.6\bin 目录下 双击zkServer.cmd文件启动zookeeper(Windows)
如图表示启动成功,它会一直停在那个cmd窗口(表示服务正在运行),直到你关闭命令窗口(服务停止)
2、springboot整合Dubbo和Zookeeper实现分布式开发
Dubbo是阿里巴巴公司开源的一个高性能优秀的服务框架,使得应用可通过高性能的 RPC 实现服务的输出和输入功能,可以和Spring框架无缝集成。
Dubbo是一款高性能、轻量级的开源Java RPC框架,它提供了三大核心能力:面向接口的远程方法调用,智能容错和负载均衡,以及服务自动注册和发现。
1. 创建服务接口模块dubbotest-api
总体上项目是这样的
dubbotest-api项目是一个maven项目,主要是定义接口与实体类,用于提供接口,类似于数据库的dao层。
主要代码如下:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>dubbotest-api</artifactId>
<!-- 打包成正式版,这样生产者与消费者就可以引用 -->
<version>1.0.0</version>
</project>
UserService.java
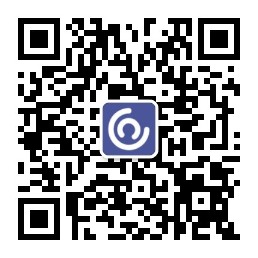
package com.example.duubo.service;
/*
* springboot接口
* ye
* 2020.12.04
* */
public interface UserService {
public String hello(String name);
}
2. 开发dubbotest-provider生产者模块
dubbotest-provider 是一个生产者通过实现dubbotest-api的接口可以提供服务,也可以自己创建自己的服务,在这一点类似于springboot 的service层
主要代码如下:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.4.0</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>dubbotest-provider</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>dubbotest-provider</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<!--dubbo -->
<dependency>
<groupId>com.alibaba.spring.boot</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>2.0.0</version>
</dependency>
<!-- zookeeper -->
<dependency>
<groupId>com.101tec</groupId>
<artifactId>zkclient</artifactId>
<version>0.10</version>
</dependency>
<!-- 接口的jar包 -->
<dependency>
<groupId>org.example</groupId>
<artifactId>dubbotest-api</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.properties
# 端口
server.port = 8081
# Dubbo配置,不能少,工程的名字
spring.application.name=dubbotest-provider
# 表示提供者,可以省略
spring.dubbo.server=true
# 注册中心地址
spring.dubbo.registry=zookeeper://localhost:2181
入口类
package com.example.dubbotestprovider;
import com.alibaba.dubbo.spring.boot.annotation.EnableDubboConfiguration;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableDubboConfiguration //开启自动配置支持
public class DubbotestProviderApplication {
public static void main(String[] args) {
SpringApplication.run(DubbotestProviderApplication.class, args);
}
}
UserServiceImpl.java
package com.example.dubbotestprovider.serviceimpl;
import com.alibaba.dubbo.config.annotation.Service;
import com.example.duubo.service.UserService;
import org.springframework.stereotype.Component;
/*
* 服务提供者
* ye
* 2020.12.04
* */
@Component
// 相当于 <dubbo:service interface="" ref="">
@Service(interfaceClass = UserService.class,timeout = 10000)
public class UserServiceImpl implements UserService {
@Override
public String hello(String name) {
return "你好呀~"+name;
}
}
3. 开发dubbotest-consumer消费者模块
消费者是使用生产者的服务的一种角色,类似于springboot的controller层
具体代码如下:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.4.0</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>dubbotest-consumer</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>dubbotest-consumer</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!--dubbo -->
<dependency>
<groupId>com.alibaba.spring.boot</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>2.0.0</version>
</dependency>
<!-- zookeeper -->
<dependency>
<groupId>com.101tec</groupId>
<artifactId>zkclient</artifactId>
<version>0.10</version>
</dependency>
<!-- 接口的jar包 -->
<dependency>
<groupId>org.example</groupId>
<artifactId>dubbotest-api</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.properties
# 端口
server.port = 8082
# Dubbo配置,不能少
spring.application.name=dubbotest-consumer
# 表示提供者,可以省略
spring.dubbo.appname=springboot-dubbo-consumer
# 注册中心地址
spring.dubbo.registry=zookeeper://localhost:2181
入口类
package com.example.dubbotestconsumer;
import com.alibaba.dubbo.spring.boot.annotation.EnableDubboConfiguration;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableDubboConfiguration //开启自动配置支持
public class DubbotestConsumerApplication {
public static void main(String[] args) {
SpringApplication.run(DubbotestConsumerApplication.class, args);
}
}
UserController.java
package com.example.dubbotestconsumer.controller;
import com.alibaba.dubbo.config.annotation.Reference;
import com.example.duubo.service.UserService;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
/*
* 消费者
* ye
* 2020.12.04
*
* */
@RestController
public class UserController {
//引用远程的dubbo服务 <dubbo:refrence id="" interface="">
@Reference
private UserService userService;
@GetMapping("/dubbotest")
public String hello(){
return userService.hello("Chasing stars");
}
}
4.测试
打开zookeeper服务,运行生产者,消费者模块,在浏览器输入 http://localhost:8082/dubbotest
出现如下图:
表示测试成功!
参考资料:https://www.w3cschool.cn/zookeeper/zookeeper_overview.html
参考资料:https://baike.baidu.com/item/Dubbo/18907815?fr=aladdin