1. 给定一个二叉树的结构
public class BinaryTree {
private BinaryTreeNode root;
public BinaryTree(BinaryTreeNode root) {
this.root = root;
}
public BinaryTree() {
}
}
public class BinaryTreeNode {
private int val;
private BinaryTreeNode left;
private BinaryTreeNode right;
public BinaryTreeNode() {
}
public BinaryTreeNode(int val) {
this.val = val;
}
public BinaryTreeNode(int val, BinaryTreeNode left, BinaryTreeNode right) {
this.val = val;
this.left = left;
this.right = right;
}
}
2. 通过数组构建二叉树
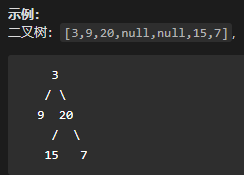
public class BinaryTreeGenerator {
public BinaryTree generate(Integer[] arr) {
if (arr == null || arr.length == 0 || arr[0] == null) {
return new BinaryTree();
}
BinaryTreeNode root = new BinaryTreeNode(arr[0]);
int index = 0;
Queue<BinaryTreeNode> queue = new LinkedList<>();
queue.offer(root);
while (!queue.isEmpty()) {
BinaryTreeNode parent = queue.poll();
index++;
if (index >= arr.length) {
break;
}
if (arr[index] != null) {
BinaryTreeNode left = new BinaryTreeNode(arr[index]);
queue.offer(left);
parent.setLeft(left);
}
index++;
if (index >= arr.length) {
break;
}
if (arr[index] != null) {
BinaryTreeNode right = new BinaryTreeNode(arr[index]);
queue.offer(right);
parent.setRight(right);
}
}
return new BinaryTree(root);
}
public static void main(String[] args) {
Integer[] arr = new Integer[]{
3, 9, 20, null, null, 15, 7};
BinaryTree binaryTree = new BinaryTreeGenerator().generate(arr);
}
}
3. 二叉树的遍历汇总
3.1 层序遍历
LeetCode题目:102. 二叉树的层序遍历
public class BinaryTree {
private BinaryTreeNode root;
public BinaryTree(BinaryTreeNode root) {
this.root = root;
}
public BinaryTree() {
}
public List<List<Integer>> levelOrder() {
List<List<Integer>> result = new ArrayList<>();
Queue<BinaryTreeNode> queue = new LinkedList<>();
queue.offer(root);
while (!queue.isEmpty()) {
int n = queue.size();
List<Integer> list = new ArrayList<>(n);
for (int i = 0; i < n; i++) {
BinaryTreeNode node = queue.poll();
list.add(node.getVal());
if (node.getLeft() != null) {
queue.offer(node.getLeft());
}
if (node.getRight() != null) {
queue.offer(node.getRight());
}
}
result.add(list);
}
return result;
}
}
3.2 锯齿型层序遍历
LeetCode题目:103. 二叉树的锯齿形层序遍历
public List<List<Integer>> zigzagLevelOrder() {
List<List<Integer>> result = new ArrayList<>();
Deque<BinaryTreeNode> deque = new LinkedList<>();
boolean direction = true;
deque.add(root);
while (!deque.isEmpty()) {
int n = deque.size();
List<Integer> list = new ArrayList<>();
if (direction) {
for (int i = 0; i < n; i++) {
BinaryTreeNode node = deque.poll();
list.add(node.getVal());
if (node.getLeft() != null) {
deque.offer(node.getLeft());
}
if (node.getRight() != null) {
deque.offer(node.getRight());
}
}
direction = false;
} else {
for (int i = 0; i < n; i++) {
BinaryTreeNode node = deque.pollLast();
list.add(node.getVal());
if (node.getRight() != null) {
deque.offerFirst(node.getRight());
}
if (node.getLeft() != null) {
deque.offerFirst(node.getLeft());
}
}
direction = true;
}
result.add(list);
}
return result;
}
3.3 前序遍历、中序遍历、后序遍历
public List<Integer> traversal() {
List<Integer> result = new ArrayList<>();
preOrderTraversal(root, result);
return result;
}
private void traversal(BinaryTreeNode node, List<Integer> result) {
if (node == null) {
return;
}
result.add(node.getVal());
preOrderTraversal(node.getLeft(), result);
preOrderTraversal(node.getRight(), result);
}