位示图
/*************************************************************************
> File Name: test2.cpp
> Author: kwh
> Mail:
> Created Time: Fri Jun 12 17:33:55 2020
************************************************************************/
#include <iostream>
#include <cstring>
#include <cstdlib>
#include <cstdio>
using namespace std;
const int MAX = 100;
int map[MAX][MAX];
typedef struct file {
char fileName[30];
int length, start;
} file;
file File[60];
int line, column;
int fileTotalNum = 0;
void mapInit() {
printf("Please enter rows and columns:");
cin >> line >> column;
for (int i = 0; i < line; i++) {
for (int j = 0; j < column; j++) {
map[i][j] = 0;
cout << ' ' << map[i][j] << ' ';
}
cout << '\n';
}
}
void allocation (int fileNum) {
int length = File[fileNum].length;
int b[60];
int x = 0;
for (int i = 0; i < line; i++) {
for (int j = 0; j < column; j++) {
if (map[i][j] == 0) {
b[x] = i * line + j;
x++;
if (x == length) break;
} else x = 0;
}
if (x == length) break;
}
if (length > x) {
cout << "There is not enough space to allocate.\n";
} else {
File[fileNum].start = b[0];
for (int i = 0; i < x; i++) {
int x1 = b[i] / column;
int y1 = b[i] % column;
map[x1][y1] = 1;
}
}
for (int i = 0; i < line; i++) {
for (int j = 0; j < column; j++) {
cout << ' ' << map[i][j] << ' ';
}
cout << '\n';
}
}
void recovery(char s[0]) {
int cnt = -1;
for (int i = 0; i < fileTotalNum; i++) {
if (strcmp(s, File[i].fileName) == 0) {
cnt = i;
break;
}
}
if (cnt == -1) {
cout << "This file is not available, please re-enter!\n";
}
int start = File[cnt].start;
int length = File[cnt].length;
for (int i = start; i < start + length; i++) {
int x1 = i / column;
int y1 = i % column;
map[x1][y1] = 0;
}
for (int i = cnt + 1; i < fileTotalNum; i++) {
strcpy(File[i - 1].fileName, File[i].fileName);
File[i - 1].start = File[i].start;
File[i - 1].length = File[i].length;
}
cout << "Recycling success\n";
for (int i = 0; i < line; i++) {
for (int j = 0; j < column; j++) {
cout << ' ' << map[i][j] << ' ';
}
cout << '\n';
}
}
void allocation() {
cout << "Please enter the file name\n";
cin >> File[fileTotalNum].fileName;
cout << "Please enter the length of the file\n";
cin >> File[fileTotalNum].length;
allocation(fileTotalNum);
fileTotalNum++;
}
void recoveryInit() {
char name[30];
cout << "Please enter the recycle file name\n";
cin >> name;
recovery(name);
fileTotalNum--;
}
void showDir() {
if (fileTotalNum == 0) cout << "Empty file!\n";
else {
for (int i = 0; i < fileTotalNum; i++) {
cout << "fileName: \n" << File[i].fileName;
cout << "The start position: \n" << File[i].start;
cout << "The file length: \n" << File[i].length;
}
}
for (int i = 0; i < line; i++) {
for (int j = 0; j < column; j++) {
cout << ' ' << map[i][j] << ' ';
}
cout << '\n';
}
}
int main() {
int mode;
mapInit();
while (1) {
cout << "1 creates the file; 2 is the recovered file; 3 shows all files\n";
cout << "Please select operation mode\n";
scanf("%d", &mode);
switch (mode) {
case 1: allocation(); break;
case 2: recoveryInit(); break;
case 3: showDir(); break;
default: cout << "Error!\n"; exit(-1);
}
}
return 0;
}
运行结果
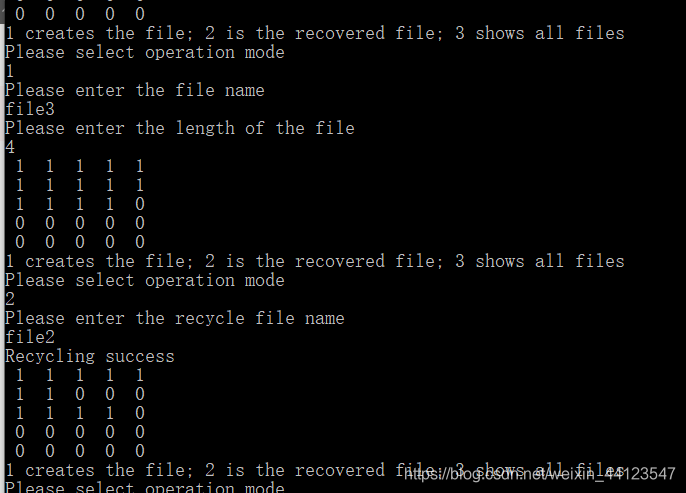