1. Morphia depends on the following third party JARs:
1) The MongoDB java driver (see the required version for each Morphia Release )
2) Optional (but needed if you use Maven)
a) CGLib
b) ProxyToys
2. If you use Maven to manage your project, you can reference Morphia as a dependency:
Repo: http://morphia.googlecode.com/svn/mavenrepo/
Project Settings:
<dependency> <groupId>com.google.code.morphia</groupId> <artifactId>morphia</artifactId> <version>###</version> </dependency> <!-Optional Jars (for certain features) but required by maven for bulding. --> <dependency> <groupId>cglib</groupId> <artifactId>cglib-nodep</artifactId> <version>[2.1_3,)</version> <type>jar</type> <optional>true</optional> </dependency> <dependency> <groupId>com.thoughtworks.proxytoys</groupId> <artifactId>proxytoys</artifactId> <version>1.0</version> <type>jar</type> <optional>true</optional> </dependency>
3. If we want to save instances of these objects to MongoDB, you need to add the Morphia annotations @Entity to the class we want to persist. We can annotate the member filed of the class with @Embedded if it doesn’t have a life outside that class. All the basic fields are automatically mapped by Morphia. If you want to exclude a field, just annotate it with @Transient .
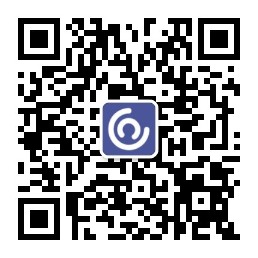
4. The "id " value can be any persist-able type; like an int, uuid, or other object. If you want an auto-generated value just declare it as an ObjectId . If you don't use an ObjectId you must set the value before saving.
5. Mapping the classes at the beginning of your application is a good practice. It allows the system to validate your classes and prepare for storing the data, and retrieving it:
Mongo mongo = ...; Morphia morphia = new Morphia(); morphia.map(Hotel.class).map(Address.class); Datastore ds = morphia.createDatastore(mongo, "my_database");
6. We can use the Datastore instance to save classes with MongoDB:
Hotel hotel = new Hotel(); hotel.setName("My Hotel"); … Address address = new Address(); address.setStreet("123 Some street"); … hotel.setAddress(address); Morphia morphia = ...; Datastore ds = morphia.createDatastore("testDB"); ds.save(hotel);
7. Load a Hotel from Mongo:
String hotelId = ...; // the ID of the hotel we want to load // and then map it to our Hotel object Hotel hotel = ds.get(Hotel.class, hotelId);
8. Load a Hotel using a query:
// it is easy to get four-star hotels. List<Hotel> fourStarHotels = ds.find(Hotel.class, "stars >=", 4).asList(); //or fourStarHotels = ds.find(Hotel.class).field("stars").greaterThenEq(4).asList();
9. In a web application environment, we would probably inject the Mongo, Morphia, and Datastore instances into a DAO/Service, and then inject that into a controller, so the controller would never directly deal with Mongo or Morphia:
public class HotelDAO extends BasicDAO<Hotel, String> { public HotelDAO(Morphia morphia, Mongo mongo ) { super(mongo, morphia, "myDB"); } } HotelDAO hDAO = ... hDAO.save(new Hotel(...));