一、引言
设计此程序(仓库管理系统,以下简称仓管)为了促进掌握 C/C++ 的基础语法知识,提高阅读、编写 C/C++ 程序的能力,掌握用 C 语言解决具体问题的一般方法和步骤,并在调试程序的过程中,提高解决问题的能力,为进一步学习其他语言打好基础。
此程序的需求如下:
建立严格的仓管系统,严格管理物资的出入库。
以名字、类型/品类、唯一识别码(name,type,id)作为每样在架货品的识别方式,并予以输入。
以唯一识别码(id)为凭据完成出库。
输出在架货品列表。
以 名字、类型/品类 (name,type)搜索在架货品,并返回在架数量与在架货品的唯一码列表。
以唯一识别码(id)搜索在架货品,并返回此在架货品的相关信息。
二、系统设计
2.1 系统结构图
2.2 系统流程图
扫描二维码关注公众号,回复:
14232729 查看本文章
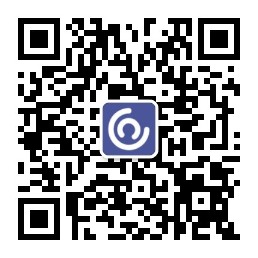
2.3 API(MIB.h)
三、系统实现
MIB.h Damo
- 主界面
- 入库界面,输入货品名字与货品类型与货品 ID,API 可以按需接入外部输入
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-juwzYr9D-1654394574493)(https://www.writebug.com/myres/static/uploads/2022/6/4/65e4c3be3af4c46c579e0ec5c32fa376.writebug)]
- 列表,自动列表
- 查找物品,以货品名与货品类型查找相对 ID
- 查找 ID,以货品 ID 查找相对货品名与货品类型
- 出库
3.1 源码
/*本源码按照GPL开源协议开源,最终著作权属于本人*/
3.1.1 MIB.h
# pragma once
# include <iostream>
# include <vector>
# include <map>
using namespace std;
class goods
{
public:
long G_id;
string G_name;
string G_type;
goods(string name, string type, long id)
{
G_id = id;
G_type = type;
G_name = name;
}
};
class wareHouseMIB
{
private:
map<string, string> Findgoods_type()//搜索货品类型
{
map<string, string> typ;
int a = 0;
for (vector<goods>::iterator i = goods_.begin(); i != goods_.end(); i++)
{
typ[i->G_type] = i->G_type;
a++;
}
return typ;
}
public:
vector<goods> goods_;
int Input(string name, string type, long id)
{
if (Findgoods(id) == -1)
goods_.push_back(goods(name, type, id));
else
cout << "输入失败" << endl << "ID所指向的货物已在架,请检查ID输入" << endl;
return 0;
}
int Findgoods(string name, string type)
{
int count_ = 0;
for (int i = 0; i < goods_.size(); i++)
{
if (goods_[i].G_name == name && goods_[i].G_type == type)
{
cout <<" "<< goods_[i].G_id<<endl;
count_++;
}
}
cout << "所寻货品在架" << count_ << "件" << endl;
return count_;
}
int Findgoods()
{
return goods_.size();
}
int Findgoods(long id)
{
for (int i = 0; i < goods_.size(); i++)
{
if (goods_[i].G_id == id)
return i;
}
return -1;
}
int outWare(long id)
{
int i = Findgoods(id);
if (i >= 0)
{
goods_.erase(goods_.begin() + i);
return 1;
}
return 0;
}
void OutgoodsList()
{
map<string, string> typ = Findgoods_type();
for (map<string, string>::iterator i = typ.begin()++; i != typ.end(); i++)
{
cout << i->first << endl;
for (vector<goods>::iterator j = goods_.begin(); j != goods_.end(); j++)
{
if (i->first == j->G_type)
{
cout << " " << j->G_name << " " << j->G_id << endl;
}
}
}
}
};
3.1.2 Main.cpp
# include <iostream>
# include <vector>
# include <map>
# include "MIB.h"
using namespace std;
int main()
{
wareHouseMIB MIB;
string name, type;
long id;
int a = 0;
while (true)
{
cout << "---------------------------------------------" << endl;
cout << "目前在架共:"<<MIB.Findgoods() << endl;
cout << "1、入库" << endl << "2、出库" << endl << "3、列表" <<endl<<"4、查找物品"<<endl<<"5、查找ID"<< endl;
cout << "---------------------------------------------" << endl;
cout << "个人制作\n" << "刘旅诚 @JoyerLiu为本程序著作权持有者\n" << "本程序以学习为主,按照GPL开源免费\n";
cout << "---------------------------------------------" << endl;
cout << "输入选项:";
cin >> a;
cout << "---------------------------------------------" << endl;
switch (a)
{
case 1:
cout << "入库" << endl;
cout << "请输入 name type id(空格隔开" << endl;
cin >> name >> type >> id;
MIB.Input(name, type, id);
cout << "完成输入 " << name << " " << type << " " << id << endl;
break;
case 2:
cout << "出库/删除" << endl;
cout << "请输入 id:" ;
cin >> id;
MIB.outWare(id);
break;
case 3:
cout << "列表" << endl;
MIB.OutgoodsList();
break;
case 4:
cout << "查询物品" << endl;
cin >> type >> name;
MIB.Findgoods(type,name);
break;
case 5:
cout << "查询ID" << endl;
cin >> id;
if (MIB.Findgoods(id) != -1)
cout << MIB.goods_[MIB.Findgoods(id)].G_name<<" "<< MIB.goods_[MIB.Findgoods(id)].G_type << endl;
else
cout << "未找到该物品或该物品未上架" << endl;
break;
}
}
}
四、总结
使用 C/C++ 完成了基本仓库管理系统的编写,调试过程中未出现死循环或内存泄漏的 Bug。本次的设计只能算是完成了基础功能,包括出库入库与数据查询功能,但并未有附加功能以及数据库接口。
通过这次课题使我巩固了我的编写程序的能力,使我对数据结构的构建有了更深的认知。
cout << “未找到该物品或该物品未上架” << endl;
break;
}
}
}
# 四、总结
使用 C/C++ 完成了基本仓库管理系统的编写,调试过程中未出现死循环或内存泄漏的 Bug。本次的设计只能算是完成了基础功能,包括出库入库与数据查询功能,但并未有附加功能以及数据库接口。
通过这次课题使我巩固了我的编写程序的能力,使我对数据结构的构建有了更深的认知。