目录
- 1、静态方法
- 2、实例方法
-
- 2.1、at()
- 2.2、charAt()
- 2.3、charCodeAt()
- 2.4、codePointAt()
- 2.5、concat()
- 2.6、endsWith()
- 2.7、includes()
- 2.8、indexOf()
- 2.9、lastIndexOf()
- 2.10、localeCompare()
- 2.11、match()
- 2.12、matchAll()
- 2.13、normalize()
- 2.14、padEnd()
- 2.15、padStart()
- 2.16、repeat()
- 2.17、replace()
- 2.18、replaceAll()
- 2.19、search()
- 2.20、slice()
- 2.21、split()
- 2.22、startsWith()
- 2.23、substring()
- 2.24、toLocaleLowerCase()
- 2.25、toLocaleUpperCase()
- 2.26、toLowerCase()
- 2.27、toString()
- 2.28、toUpperCase()
- 2.29、trim()
- 2.30、trimRight()
- 2.31、trimStart()
- 2.32、valueOf()
- 3、实例属性
- 后记
1、静态方法
1.1、fromCharCode()
1.1.1、概述
静态 String.fromCharCode() 方法返回由指定的 UTF-16 代码单元序列创建的字符串。
1.1.2、语法
String.fromCharCode(num1[, ...[, numN]])
1.1.3、参数
num1, …, numN:一系列 UTF-16 代码单元的数字。范围介于 0 到 65535(0xFFFF)之间。大于 0xFFFF 的数字将被截断。不进行有效性检查。
1.1.4、返回值
一个长度为 N 的字符串,由 N 个指定的 UTF-16 代码单元组成。
1.1.5、示例
String.fromCharCode(65, 66, 67); // 返回 "ABC"
String.fromCharCode(0x2014); // 返回 "—"
String.fromCharCode(0x12014); // 也是返回 "—"; 数字 1 被剔除并忽略
String.fromCharCode(8212); // 也是返回 "—"; 8212 是 0x2014 的十进制表示
1.2、fromCodePoint()
1.2.1、概述
String.fromCodePoint() 静态方法返回使用指定的代码点序列创建的字符串。
1.2.2、语法
String.fromCodePoint(num1[, ...[, numN]])
1.2.3、参数
num1, …, numN:一串 Unicode 编码位置,即“代码点”。
1.2.4、返回值
使用指定的 Unicode 编码位置创建的字符串。
1.2.5、异常
RangeError:如果传入无效的 Unicode 编码,将会抛出一个RangeError (例如: “RangeError: NaN is not a valid code point”)。
1.2.6、示例
String.fromCodePoint(42); // "*"
String.fromCodePoint(65, 90); // "AZ"
String.fromCodePoint(0x404); // "\u0404"
String.fromCodePoint(0x2f804); // "\uD87E\uDC04"
String.fromCodePoint(194564); // "\uD87E\uDC04"
String.fromCodePoint(0x1d306, 0x61, 0x1d307); // "\uD834\uDF06a\uD834\uDF07"
String.fromCodePoint("_"); // RangeError
String.fromCodePoint(Infinity); // RangeError
String.fromCodePoint(-1); // RangeError
String.fromCodePoint(3.14); // RangeError
String.fromCodePoint(3e-2); // RangeError
String.fromCodePoint(NaN); // RangeError
1.2.7、向下兼容
if (!String.fromCodePoint)
(function (stringFromCharCode) {
var fromCodePoint = function (_) {
var codeUnits = [],
codeLen = 0,
result = "";
for (var index = 0, len = arguments.length; index !== len; ++index) {
var codePoint = +arguments[index];
// correctly handles all cases including `NaN`, `-Infinity`, `+Infinity`
// The surrounding `!(...)` is required to correctly handle `NaN` cases
// The (codePoint>>>0) === codePoint clause handles decimals and negatives
if (!(codePoint < 0x10ffff && codePoint >>> 0 === codePoint)) throw RangeError("Invalid code point: " + codePoint);
if (codePoint <= 0xffff) {
// BMP code point
codeLen = codeUnits.push(codePoint);
} else {
// Astral code point; split in surrogate halves
// https://mathiasbynens.be/notes/javascript-encoding#surrogate-formulae
codePoint -= 0x10000;
codeLen = codeUnits.push(
(codePoint >> 10) + 0xd800, // highSurrogate
(codePoint % 0x400) + 0xdc00 // lowSurrogate
);
}
if (codeLen >= 0x3fff) {
result += stringFromCharCode.apply(null, codeUnits);
codeUnits.length = 0;
}
}
return result + stringFromCharCode.apply(null, codeUnits);
};
try {
// IE 8 only supports `Object.defineProperty` on DOM elements
Object.defineProperty(String, "fromCodePoint", {
value: fromCodePoint,
configurable: true,
writable: true
});
} catch (e) {
String.fromCodePoint = fromCodePoint;
}
})(String.fromCharCode);
1.3、raw()
1.3.1、概述
String.raw() 是一个模板字符串的标签函数,它的作用类似于 Python 中的字符串前缀 r 和 C# 中的字符串前缀 @,是用来获取一个模板字符串的原始字符串的,比如说,占位符(例如 ${foo})会被处理为它所代表的其他字符串,而转义字符(例如 \n)不会。
1.3.2、语法
String.raw(callSite, ...substitutions)
String.raw`templateString`
1.3.3、参数
callSite:一个模板字符串的“调用点对象”。类似 { raw: [‘foo’, ‘bar’, ‘baz’] }。
…substitutions:任意个可选的参数,表示任意个内插表达式对应的值。
templateString:模板字符串,可包含占位符(${…})。
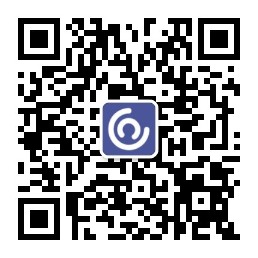
1.3.4、返回值
给定模板字符串的原始字符串。
1.3.5、异常
TypeError:如果第一个参数没有传入一个格式正确的对象,则会抛出 TypeError 异常。
1.3.6、示例
String.raw`Hi\n${
2 + 3}!`;
// 'Hi\\n5!',Hi 后面的字符不是换行符,\ 和 n 是两个不同的字符
String.raw`Hi\u000A!`;
// "Hi\\u000A!",同上,这里得到的会是 \、u、0、0、0、A 6 个字符,
// 任何类型的转义形式都会失效,保留原样输出,不信你试试.length
let name = "Bob";
String.raw`Hi\n${
name}!`;
// "Hi\nBob!",内插表达式还可以正常运行
// 正常情况下,你也许不需要将 String.raw() 当作函数调用。
// 但是为了模拟 `t${0}e${1}s${2}t` 你可以这样做:
String.raw({
raw: "test" }, 0, 1, 2); // 't0e1s2t'
// 注意这个测试,传入一个 string,和一个类似数组的对象
// 下面这个函数和 `foo${2 + 3}bar${'Java' + 'Script'}baz` 是相等的。
String.raw(
{
raw: ["foo", "bar", "baz"]
},
2 + 3,
"Java" + "Script"
); // 'foo5barJavaScriptbaz'
2、实例方法
2.1、at()
2.1.1、概述
at() 方法接受一个整数值,并返回一个新的 String,该字符串由位于指定偏移量处的单个 UTF-16 码元组成。该方法允许正整数和负整数。负整数从字符串中的最后一个字符开始倒数。
2.1.2、语法
at(index)
2.1.3、参数
index:要返回的字符串字符的索引(位置)。当传递负数时,支持从字符串末端开始的相对索引;也就是说,如果使用负数,返回的字符将从字符串的末端开始倒数。
2.1.4、返回值
由位于指定位置的单个 UTF-16 码元组成的 String。如果找不到指定的索引,则返回 undefined 。
2.1.5、示例
// A function which returns the last character of a given string
function returnLast(arr) {
return arr.at(-1);
}
let invoiceRef = "myinvoice01";
console.log(returnLast(invoiceRef));
// Logs: '1'
invoiceRef = "myinvoice02";
console.log(returnLast(invoiceRef));
// Logs: '2'
2.2、charAt()
2.2.1、概述
charAt() 方法从一个字符串中返回指定的字符。
2.2.2、语法
str.charAt(index)
2.2.3、参数
index:一个介于 0 和字符串长度减 1 之间的整数。(0~length-1) 如果没有提供索引,charAt() 将使用 0。
2.2.4、示例
var anyString = "Brave new world";
console.log("The character at index 0 is '" + anyString.charAt(0) + "'");
console.log("The character at index 1 is '" + anyString.charAt(1) + "'");
console.log("The character at index 2 is '" + anyString.charAt(2) + "'");
console.log("The character at index 3 is '" + anyString.charAt(3) + "'");
console.log("The character at index 4 is '" + anyString.charAt(4) + "'");
console.log("The character at index 999 is '" + anyString.charAt(999) + "'");
结果:
The character at index 0 is 'B'
The character at index 1 is 'r'
The character at index 2 is 'a'
The character at index 3 is 'v'
The character at index 4 is 'e'
The character at index 999 is ''
2.3、charCodeAt()
2.3.1、概述
charCodeAt() 方法返回 0 到 65535 之间的整数,表示给定索引处的 UTF-16 代码单元
2.3.2、语法
str.charCodeAt(index)
2.3.3、参数
index:一个大于等于 0,小于字符串长度的整数。如果不是一个数值,则默认为 0。
2.3.4、返回值
指定 index 处字符的 UTF-16 代码单元值的一个数字;如果 index 超出范围,charCodeAt() 返回 NaN。
2.3.5、示例
"ABC".charCodeAt(0); // returns 65:"A"
"ABC".charCodeAt(1); // returns 66:"B"
"ABC".charCodeAt(2); // returns 67:"C"
"ABC".charCodeAt(3); // returns NaN
2.4、codePointAt()
2.4.1、概述
codePointAt() 方法返回 一个 Unicode 编码点值的非负整数。
2.4.2、语法
str.codePointAt(pos)
2.4.3、参数
pos:这个字符串中需要转码的元素的位置。
2.4.4、返回值
返回值是在字符串中的给定索引的编码单元体现的数字,如果在索引处没找到元素则返回 undefined 。
2.4.5、示例
"ABC".codePointAt(1); // 66
"\uD800\uDC00".codePointAt(0); // 65536
"XYZ".codePointAt(42); // undefined
2.4.6、向下兼容
/*! http://mths.be/codepointat v0.1.0 by @mathias */
if (!String.prototype.codePointAt) {
(function () {
"use strict"; // 严格模式,needed to support `apply`/`call` with `undefined`/`null`
var codePointAt = function (position) {
if (this == null) {
throw TypeError();
}
var string = String(this);
var size = string.length;
// 变成整数
var index = position ? Number(position) : 0;
if (index != index) {
// better `isNaN`
index = 0;
}
// 边界
if (index < 0 || index >= size) {
return undefined;
}
// 第一个编码单元
var first = string.charCodeAt(index);
var second;
if (
// 检查是否开始 surrogate pair
first >= 0xd800 &&
first <= 0xdbff && // high surrogate
size > index + 1 // 下一个编码单元
) {
second = string.charCodeAt(index + 1);
if (second >= 0xdc00 && second <= 0xdfff) {
// low surrogate
// http://mathiasbynens.be/notes/javascript-encoding#surrogate-formulae
return (first - 0xd800) * 0x400 + second - 0xdc00 + 0x10000;
}
}
return first;
};
if (Object.defineProperty) {
Object.defineProperty(String.prototype, "codePointAt", {
value: codePointAt,
configurable: true,
writable: true
});
} else {
String.prototype.codePointAt = codePointAt;
}
})();
}
2.5、concat()
2.5.1、概述
concat() 方法将一个或多个字符串与原字符串连接合并,形成一个新的字符串并返回。
2.5.2、语法
str.concat(str2, [, ...strN])
2.5.3、参数
str2 [, …strN]:需要连接到 str 的字符串。
2.5.4、返回值
一个新的字符串,包含参数所提供的连接字符串。
2.5.5、性能
强烈建议使用赋值操作符(+, +=)代替 concat 方法。
2.5.6、示例
let hello = "Hello, ";
console.log(hello.concat("Kevin", ". Have a nice day."));
// Hello, Kevin. Have a nice day.
let greetList = ["Hello", " ", "Venkat", "!"];
"".concat(...greetList); // "Hello Venkat!"
"".concat({
}); // [object Object]
"".concat([]); // ""
"".concat(null); // "null"
"".concat(true); // "true"
"".concat(4, 5); // "45"
2.6、endsWith()
2.6.1、概述
endsWith()方法用来判断当前字符串是否是以另外一个给定的子字符串“结尾”的,根据判断结果返回 true 或 false。
2.6.2、语法
str.endsWith(searchString[, length])
2.6.3、参数
searchString:要搜索的子字符串。
length【可选】:作为 str 的长度。默认值为 str.length。
2.6.4、返回值
如果传入的子字符串在搜索字符串的末尾则返回true;否则将返回 false。
2.6.5、示例
var str = "To be, or not to be, that is the question.";
alert(str.endsWith("question.")); // true
alert(str.endsWith("to be")); // false
alert(str.endsWith("to be", 19)); // true
2.6.6、向下兼容
if (!String.prototype.endsWith) {
String.prototype.endsWith = function (search, this_len) {
if (this_len === undefined || this_len > this.length) {
this_len = this.length;
}
return this.substring(this_len - search.length, this_len) === search;
};
}
2.7、includes()
2.7.1、概述
includes() 方法用于判断一个字符串是否包含在另一个字符串中,根据情况返回 true 或 false。
2.7.2、语法
str.includes(searchString[, position])
2.7.3、参数
searchString:要在此字符串中搜索的字符串。
position【可选】:从当前字符串的哪个索引位置开始搜寻子字符串,默认值为 0。
2.7.4、返回值
如果当前字符串包含被搜寻的字符串,就返回 true;否则返回 false。
2.7.5、示例
var str = "To be, or not to be, that is the question.";
console.log(str.includes("To be")); // true
console.log(str.includes("question")); // true
console.log(str.includes("nonexistent")); // false
console.log(str.includes("To be", 1)); // false
console.log(str.includes("TO BE")); // false
2.7.6、示例
if (!String.prototype.includes) {
String.prototype.includes = function (search, start) {
"use strict";
if (typeof start !== "number") {
start = 0;
}
if (start + search.length > this.length) {
return false;
} else {
return this.indexOf(search, start) !== -1;
}
};
}
2.8、indexOf()
2.8.1、概述
indexOf() 方法返回调用它的 String 对象中第一次出现的指定值的索引,从 fromIndex 处进行搜索。如果未找到该值,则返回 -1。
2.8.2、语法
str.indexOf(searchValue [, fromIndex])
2.8.3、参数
searchValue:要被查找的字符串值。如果没有提供确切地提供字符串,searchValue 会被强制设置为 “undefined”, 然后在当前字符串中查找这个值。举个例子:‘undefined’.indexOf() 将会返回 0,因为 undefined 在位置 0 处被找到,但是 ‘undefine’.indexOf() 将会返回 -1 ,因为字符串 ‘undefined’ 未被找到。
fromIndex 【可选】:数字表示开始查找的位置。可以是任意整数,默认值为 0。如果 fromIndex 的值小于 0,或者大于 str.length ,那么查找分别从 0 和str.length 开始。举个例子,‘hello world’.indexOf(‘o’, -5) 返回 4 ,因为它是从位置0处开始查找,然后 o 在位置4处被找到。另一方面,‘hello world’.indexOf(‘o’, 11) (或 fromIndex 填入任何大于11的值)将会返回 -1 ,因为开始查找的位置11处,已经是这个字符串的结尾了。
2.8.4、返回值
查找的字符串 searchValue 的第一次出现的索引,如果没有找到,则返回 -1。
2.8.5、示例
var anyString = "Brave new world";
console.log("The index of the first w from the beginning is " + anyString.indexOf("w"));
// logs 8
console.log("The index of the first w from the end is " + anyString.lastIndexOf("w"));
// logs 10
console.log("The index of 'new' from the beginning is " + anyString.indexOf("new"));
// logs 6
console.log("The index of 'new' from the end is " + anyString.lastIndexOf("new"));
// logs 6
2.9、lastIndexOf()
2.9.1、概述
lastIndexOf() 方法返回调用String 对象的指定值最后一次出现的索引,在一个字符串中的指定位置 fromIndex处从后向前搜索。如果没找到这个特定值则返回-1 。
2.9.2、语法
str.lastIndexOf(searchValue[, fromIndex])
2.9.3、参数
searchValue:一个字符串,表示被查找的值。如果searchValue是空字符串,则返回fromIndex。
fromIndex【可选】:待匹配字符串 searchValue 的开头一位字符从 str 的第 fromIndex 位开始向左回向查找。fromIndex默认值是 +Infinity。如果 fromIndex >= str.length ,则会搜索整个字符串。如果 fromIndex < 0 ,则等同于 fromIndex == 0。
2.9.4、返回值
返回指定值最后一次出现的索引 (该索引仍是以从左至右 0 开始记数的),如果没找到则返回-1。
2.9.5、示例
var anyString = "Brave new world";
console.log("The index of the first w from the beginning is " + anyString.indexOf("w"));
// Displays 8
console.log("The index of the first w from the end is " + anyString.lastIndexOf("w"));
// Displays 10
console.log("The index of 'new' from the beginning is " + anyString.indexOf("new"));
// Displays 6
console.log("The index of 'new' from the end is " + anyString.lastIndexOf("new"));
// Displays 6
2.10、localeCompare()
2.10.1、概述
localeCompare() 方法返回一个数字来指示一个参考字符串是否在排序顺序前面或之后或与给定字符串相同。
2.10.2、语法
referenceStr.localeCompare(compareString[, locales[, options]])
2.10.3、参数
2.10.3.1、compareString
用来比较的字符串
2.10.3.2、locales
可选。用来表示一种或多种语言或区域的一个符合 BCP 47 标准的字符串或一个字符串数组。locales 参数的一般形式与解释,详情请参考 Intl page。 下列的 Unicode 扩展关键词是允许的:co为了某些地域多样的排序规则。可能的值包括: “big5han”, “dict”, “direct”, “ducet”, “gb2312”, “phonebk”, “phonetic”, “pinyin”, “reformed”, “searchjl”, “stroke”, “trad”, “unihan”。 “standard” 和"search" 这两个值是被忽略的;它们被 options 的属性 usage 代替 (往下看)。kn指定数值排序是否应该被使用,像是这样 “1” < “2” < “10”。可能的值是 “true” 和 “false”。这个选项能被通过options 属性设置或通过 Unicode 扩展。假如两个都被设置了,则 options 优先。(“language-region-u-kn-true|false”)kf 指定是否优先对大写字母或小写字母排序。可能的值有 “upper”, “lower”, 或 “false” (use the locale’s default)。这个选项能被通过 options 属性设置或通过 Unicode 扩展。假如两个都被设置了,则 options 优先。(“language-region-u-kf-upper|lower|false”)
2.10.3.3、options
可选。支持下列的一些或全部属性的一个对象:
localeMatcher
地域匹配算法的使用。可能的值是 “lookup” 和 “best fit”; 默认的值是 “best fit”。更多相关的资料,请参考 Intl page.
usage
指定比较的目标是排序或者是搜索。可能的值是 “sort” 和 “search”;默认是 “sort”.
sensitivity
指定排序程序的敏感度(Which differences in the strings should lead to non-zero result values.)可能的有:
- “base”: 只有不同的字母字符串比较是不相等的。举个例子: a ≠ b, a = á, a = A.
- “accent”: 只有不同的字母或读音比较是不相等的。举个例子: a ≠ b, a ≠ á, a = A.
- “case”: 只有不同的字母或大小写比较是不相等的。举个例子: a ≠ b, a = á, a ≠ A.
- “variant”: 不同的字母或读音及其它有区别的标志或大小写都是不相等的, 还有其它的差异可能也会考虑到。举个例子: a ≠ b,
a ≠ á, a ≠ A. The default is “variant” for usage “sort”; it’s locale
dependent for usage “search”。
ignorePunctuation
指定是否忽略标点。可能的值是 true and false; 默认为 false.
numeric
是否指定使用数字排序,像这样 “1” < “2” < “10”。可能的值是 true 和 false;默认为 false。这个选项能被通过options 属性设置或通过 Unicode 扩展。假如两个都被设置了,则 options 优先。实现不用必须支持这个属性。
caseFirst
指定大小写有限排序。可能的值有 “upper”、“lower” 或 “false” (use the locale’s default); 默认为 “false”. 这个选项能被通过 options 属性设置或通过 Unicode 扩展。假如两个都被设置了,则 options 优先。实现不用必须支持这个属性。
2.10.4、返回值
如果引用字符存在于比较字符之前则为负数; 如果引用字符存在于比较字符之后则为正数; 相等的时候返回 0
2.10.5、示例
// The letter "a" is before "c" yielding a negative value
"a".localeCompare("c");
// -2 or -1 (or some other negative value)
// Alphabetically the word "check" comes after "against" yielding a positive value
"check".localeCompare("against");
// 2 or 1 (or some other positive value)
// "a" and "a" are equivalent yielding a neutral value of zero
"a".localeCompare("a");
// 0
2.11、match()
2.11.1、概述
match() 方法检索返回一个字符串匹配正则表达式的结果。
2.11.2、语法
str.match(regexp)
2.11.3、参数
regexp:一个正则表达式对象。如果传入一个非正则表达式对象,则会隐式地使用 new RegExp(obj) 将其转换为一个 RegExp 。如果你没有给出任何参数并直接使用 match() 方法 ,你将会得到一 个包含空字符串的 Array :[“”] 。
2.11.4、示例
- 如果使用 g 标志,则将返回与完整正则表达式匹配的所有结果,但不会返回捕获组。
- 如果未使用 g 标志,则仅返回第一个完整匹配及其相关的捕获组(Array)。 在这种情况下,返回的项目将具有如下所述的其他属性。
2.11.5、示例
var str = "For more information, see Chapter 3.4.5.1";
var re = /see (chapter \d+(\.\d)*)/i;
var found = str.match(re);
console.log(found);
// logs [ 'see Chapter 3.4.5.1',
// 'Chapter 3.4.5.1',
// '.1',
// index: 22,
// input: 'For more information, see Chapter 3.4.5.1' ]
// 'see Chapter 3.4.5.1' 是整个匹配。
// 'Chapter 3.4.5.1' 被'(chapter \d+(\.\d)*)'捕获。
// '.1' 是被'(\.\d)'捕获的最后一个值。
// 'index' 属性 (22) 是整个匹配从零开始的索引。
// 'input' 属性是被解析的原始字符串。
2.12、matchAll()
2.12.1、概述
matchAll() 方法返回一个包含所有匹配正则表达式的结果及分组捕获组的迭代器。
2.12.2、语法
str.matchAll(regexp)
2.12.3、参数
regexp:正则表达式对象。如果所传参数不是一个正则表达式对象,则会隐式地使用 new RegExp(obj) 将其转换为一个 RegExp 。
RegExp必须是设置了全局模式g的形式,否则会抛出异常TypeError。
2.12.4、返回值
一个迭代器(不可重用,结果耗尽需要再次调用方法,获取一个新的迭代器)。
2.12.5、示例
const regexp = RegExp("foo[a-z]*", "g");
const str = "table football, foosball";
let match;
while ((match = regexp.exec(str)) !== null) {
console.log(`Found ${
match[0]} start=${
match.index} end=${
regexp.lastIndex}.`);
// expected output: "Found football start=6 end=14."
// expected output: "Found foosball start=16 end=24."
}
2.13、normalize()
2.13.1、概述
normalize() 方法会按照指定的一种 Unicode 正规形式将当前字符串正规化。(如果该值不是字符串,则首先将其转换为一个字符串)。
2.13.2、语法
str.normalize([form])
2.13.3、参数
form 【可选】
四种 Unicode 正规形式(Unicode Normalization Form)“NFC”、“NFD”、“NFKC”,或 “NFKD” 其中的一个,默认值为 “NFC”。
这四个值的含义分别如下:
- “NFC”
Canonical Decomposition, followed by Canonical Composition.
- “NFD”
Canonical Decomposition.
- “NFKC”
Compatibility Decomposition, followed by Canonical Composition.
- “NFKD”
Compatibility Decomposition.
2.13.4、返回值
含有给定字符串的 Unicode 规范化形式的字符串。
2.13.5、异常
RangeError:如果给 form 传入了上述四个字符串以外的参数,则会抛出 RangeError 异常。
2.13.6、示例
// Initial string
// U+1E9B: LATIN SMALL LETTER LONG S WITH DOT ABOVE
// U+0323: COMBINING DOT BELOW
var str = "\u1E9B\u0323";
// Canonically-composed form (NFC)
// U+1E9B: LATIN SMALL LETTER LONG S WITH DOT ABOVE
// U+0323: COMBINING DOT BELOW
str.normalize("NFC"); // "\u1E9B\u0323"
str.normalize(); // same as above
// Canonically-decomposed form (NFD)
// U+017F: LATIN SMALL LETTER LONG S
// U+0323: COMBINING DOT BELOW
// U+0307: COMBINING DOT ABOVE
str.normalize("NFD"); // "\u017F\u0323\u0307"
// Compatibly-composed (NFKC)
// U+1E69: LATIN SMALL LETTER S WITH DOT BELOW AND DOT ABOVE
str.normalize("NFKC"); // "\u1E69"
// Compatibly-decomposed (NFKD)
// U+0073: LATIN SMALL LETTER S
// U+0323: COMBINING DOT BELOW
// U+0307: COMBINING DOT ABOVE
str.normalize("NFKD"); // "\u0073\u0323\u0307"
2.14、padEnd()
2.14.1、概述
padEnd() 方法会用一个字符串填充当前字符串(如果需要的话则重复填充),返回填充后达到指定长度的字符串。从当前字符串的末尾(右侧)开始填充。
2.14.2、语法
str.padEnd(targetLength [, padString])
2.14.3、参数
targetLength
当前字符串需要填充到的目标长度。如果这个数值小于当前字符串的长度,则返回当前字符串本身。
padString 【可选】
填充字符串。如果字符串太长,使填充后的字符串长度超过了目标长度,则只保留最左侧的部分,其他部分会被截断。此参数的缺省值为 " "(U+0020)。
2.14.4、返回值
在原字符串末尾填充指定的填充字符串直到目标长度所形成的新字符串。
2.14.5、示例
"abc".padEnd(10); // "abc "
"abc".padEnd(10, "foo"); // "abcfoofoof"
"abc".padEnd(6, "123456"); // "abc123"
"abc".padEnd(1); // "abc"
2.14.6、向下兼容
// https://github.com/uxitten/polyfill/blob/master/string.polyfill.js
// https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/padEnd
if (!String.prototype.padEnd) {
String.prototype.padEnd = function padEnd(targetLength, padString) {
targetLength = targetLength >> 0; //floor if number or convert non-number to 0;
padString = String(typeof padString !== "undefined" ? padString : "");
if (this.length > targetLength) {
return String(this);
} else {
targetLength = targetLength - this.length;
if (targetLength > padString.length) {
padString += padString.repeat(targetLength / padString.length); //append to original to ensure we are longer than needed
}
return String(this) + padString.slice(0, targetLength);
}
};
}
2.15、padStart()
2.15.1、概述
padStart() 方法用另一个字符串填充当前字符串 (如果需要的话,会重复多次),以便产生的字符串达到给定的长度。从当前字符串的左侧开始填充。
2.15.2、语法
str.padStart(targetLength [, padString])
2.15.3、参数
targetLength
当前字符串需要填充到的目标长度。如果这个数值小于当前字符串的长度,则返回当前字符串本身。
padString 【可选】
填充字符串。如果字符串太长,使填充后的字符串长度超过了目标长度,则只保留最左侧的部分,其他部分会被截断。此参数的默认值为 " "(U+0020)。
2.15.4、返回值
在原字符串开头填充指定的填充字符串直到目标长度所形成的新字符串。
2.15.5、示例
"abc".padStart(10); // " abc"
"abc".padStart(10, "foo"); // "foofoofabc"
"abc".padStart(6, "123465"); // "123abc"
"abc".padStart(8, "0"); // "00000abc"
"abc".padStart(1); // "abc"
2.15.6、向下兼容
// https://github.com/uxitten/polyfill/blob/master/string.polyfill.js
// https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/padStart
if (!String.prototype.padStart) {
String.prototype.padStart = function padStart(targetLength, padString) {
targetLength = targetLength >> 0; //floor if number or convert non-number to 0;
padString = String(typeof padString !== "undefined" ? padString : " ");
if (this.length > targetLength) {
return String(this);
} else {
targetLength = targetLength - this.length;
if (targetLength > padString.length) {
padString += padString.repeat(targetLength / padString.length); //append to original to ensure we are longer than needed
}
return padString.slice(0, targetLength) + String(this);
}
};
}
2.16、repeat()
2.16.1、概述
repeat() 构造并返回一个新字符串,该字符串包含被连接在一起的指定数量的字符串的副本。
2.16.2、语法
str.repeat(count)
2.16.3、参数
count
介于 0 和 +Infinity 之间的整数。表示在新构造的字符串中重复了多少遍原字符串。
2.16.4、返回值
包含指定字符串的指定数量副本的新字符串。
2.16.5、异常
RangeError:重复次数不能为负数。
RangeError:重复次数必须小于 infinity,且长度不会大于最长的字符串。
2.16.6、示例
"abc".repeat(-1); // RangeError: repeat count must be positive and less than inifinity
"abc".repeat(0); // ""
"abc".repeat(1); // "abc"
"abc".repeat(2); // "abcabc"
"abc".repeat(3.5); // "abcabcabc" 参数 count 将会被自动转换成整数。
"abc"
.repeat(1 / 0)(
// RangeError: repeat count must be positive and less than inifinity
{
toString: () => "abc", repeat: String.prototype.repeat }
)
.repeat(2);
//"abcabc",repeat 是一个通用方法,也就是它的调用者可以不是一个字符串对象。
2.16.7、向下兼容
if (!String.prototype.repeat) {
String.prototype.repeat = function (count) {
"use strict";
if (this == null) {
throw new TypeError("can't convert " + this + " to object");
}
var str = "" + this;
count = +count;
if (count != count) {
count = 0;
}
if (count < 0) {
throw new RangeError("repeat count must be non-negative");
}
if (count == Infinity) {
throw new RangeError("repeat count must be less than infinity");
}
count = Math.floor(count);
if (str.length == 0 || count == 0) {
return "";
}
// 确保 count 是一个 31 位的整数。这样我们就可以使用如下优化的算法。
// 当前(2014 年 8 月),绝大多数浏览器都不能支持 1 << 28 长的字符串,所以:
if (str.length * count >= 1 << 28) {
throw new RangeError("repeat count must not overflow maximum string size");
}
var rpt = "";
for (;;) {
if ((count & 1) == 1) {
rpt += str;
}
count >>>= 1;
if (count == 0) {
break;
}
str += str;
}
return rpt;
};
}
2.17、replace()
2.17.1、概述
replace() 方法返回一个由替换值(replacement)替换部分或所有的模式(pattern)匹配项后的新字符串。模式可以是一个字符串或者一个正则表达式,替换值可以是一个字符串或者一个每次匹配都要调用的回调函数。如果pattern是字符串,则仅替换第一个匹配项。
原字符串不会改变。
2.17.2、语法
str.replace(regexp|substr, newSubStr|function)
2.17.3、参数
regexp (pattern)
一个RegExp 对象或者其字面量。该正则所匹配的内容会被第二个参数的返回值替换掉。
substr (pattern)
一个将被 newSubStr 替换的 字符串。其被视为一整个字符串,而不是一个正则表达式。仅第一个匹配项会被替换。
newSubStr (replacement)
用于替换掉第一个参数在原字符串中的匹配部分的字符串。该字符串中可以内插一些特殊的变量名。参考下面的使用字符串作为参数。
function (replacement)
一个用来创建新子字符串的函数,该函数的返回值将替换掉第一个参数匹配到的结果。参考下面的指定一个函数作为参数。
2.17.4、返回值
一个部分或全部匹配由替代模式所取代的新的字符串。
2.17.5、示例
var str = "Twas the night before Xmas...";
var newstr = str.replace(/xmas/i, "Christmas");
console.log(newstr); // Twas the night before Christmas...
2.18、replaceAll()
2.18.1、概述
replaceAll() 方法返回一个新字符串,新字符串所有满足 pattern 的部分都已被replacement 替换。pattern可以是一个字符串或一个 RegExp, replacement可以是一个字符串或一个在每次匹配被调用的函数。
原始字符串保持不变。
2.18.2、语法
const newStr = str.replaceAll(regexp|substr, newSubstr|function)
2.18.3、参数
regexp (pattern)
带有全局标志的RegExp对象或文字。匹配将被替换为newSubstr或指定函数返回的值。不带全局(“g”)标志的RegExp将抛出TypeError: “replaceAll must be called with a global RegExp”.
substr (pattern)
一个将被 newSubStr 替换的 字符串。其被视为一整个字符串,而不是一个正则表达式。仅第一个匹配项会被替换。
newSubStr (replacement)
用于替换掉第一个参数在原字符串中的匹配部分的字符串。该字符串中可以内插一些特殊的变量名。参考下面的使用字符串作为参数。
function (replacement)
一个用来创建新子字符串的函数,该函数的返回值将替换掉第一个参数匹配到的结果。
2.18.4、返回值
一个新字符串,所有匹配都被替换掉。
2.18.5、示例
"aabbcc".replaceAll("b", ".");
// 'aa..cc'
2.19、search()
2.19.1、概述
search() 方法执行正则表达式和 String 对象之间的一个搜索匹配。
2.19.2、语法
str.search(regexp)
2.19.3、参数
regexp:一个正则表达式(regular expression)对象。如果传入一个非正则表达式对象 regexp,则会使用 new RegExp(regexp) 隐式地将其转换为正则表达式对象。
2.19.4、返回值
如果匹配成功,则 search() 返回正则表达式在字符串中首次匹配项的索引;否则,返回 -1。
2.19.5、示例
var str = "hey JudE";
var re = /[A-Z]/g;
var re2 = /[.]/g;
console.log(str.search(re)); // returns 4, which is the index of the first capital letter "J"
console.log(str.search(re2)); // returns -1 cannot find '.' dot punctuation
2.20、slice()
2.20.1、概述
slice() 方法提取某个字符串的一部分,并返回一个新的字符串,且不会改动原字符串。
2.20.2、语法
str.slice(beginIndex[, endIndex])
2.20.3、参数
beginIndex
从该索引(以 0 为基数)处开始提取原字符串中的字符。如果值为负数,会被当做 strLength + beginIndex 看待,这里的strLength 是字符串的长度(例如, 如果 beginIndex 是 -3 则看作是:strLength - 3)
endIndex
可选。在该索引(以 0 为基数)处结束提取字符串。如果省略该参数,slice() 会一直提取到字符串末尾。如果该参数为负数,则被看作是 strLength + endIndex,这里的 strLength 就是字符串的长度 (例如,如果 endIndex 是 -3,则是,strLength - 3)。
2.20.4、返回值
返回一个从原字符串中提取出来的新字符串
2.20.5、示例
var str1 = "The morning is upon us.", // str1 的长度 length 是 23。
str2 = str1.slice(1, 8),
str3 = str1.slice(4, -2),
str4 = str1.slice(12),
str5 = str1.slice(30);
console.log(str2); // 输出:he morn
console.log(str3); // 输出:morning is upon u
console.log(str4); // 输出:is upon us.
console.log(str5); // 输出:""
给 slice() 传入负值索引
var str = "The morning is upon us.";
str.slice(-3); // 返回 'us.'
str.slice(-3, -1); // 返回 'us'
str.slice(0, -1); // 返回 'The morning is upon us'
2.21、split()
2.21.1、概述
split() 方法使用指定的分隔符字符串将一个String对象分割成子字符串数组,以一个指定的分割字串来决定每个拆分的位置。
2.21.2、语法
str.split([separator[, limit]])
2.21.3、参数
separator
指定表示每个拆分应发生的点的字符串。separator 可以是一个字符串或正则表达式。 如果纯文本分隔符包含多个字符,则必须找到整个字符串来表示分割点。如果在 str 中省略或不出现分隔符,则返回的数组包含一个由整个字符串组成的元素。如果分隔符为空字符串,则将 str 原字符串中每个字符的数组形式返回。
limit
一个整数,限定返回的分割片段数量。当提供此参数时,split 方法会在指定分隔符的每次出现时分割该字符串,但在限制条目已放入数组时停止。如果在达到指定限制之前达到字符串的末尾,它可能仍然包含少于限制的条目。新数组中不返回剩下的文本。
2.21.4、返回值
返回源字符串以分隔符出现位置分隔而成的一个 Array
2.21.5、示例
function splitString(stringToSplit, separator) {
var arrayOfStrings = stringToSplit.split(separator);
console.log('The original string is: "' + stringToSplit + '"');
console.log('The separator is: "' + separator + '"');
console.log("The array has " + arrayOfStrings.length + " elements: ");
for (var i = 0; i < arrayOfStrings.length; i++) console.log(arrayOfStrings[i] + " / ");
}
var tempestString = "Oh brave new world that has such people in it.";
var monthString = "Jan,Feb,Mar,Apr,May,Jun,Jul,Aug,Sep,Oct,Nov,Dec";
var space = " ";
var comma = ",";
splitString(tempestString, space);
splitString(tempestString);
splitString(monthString, comma);
结果为:
The original string is: "Oh brave new world that has such people in it."
The separator is: " "
The array has 10 elements: Oh / brave / new / world / that / has / such / people / in / it. /
The original string is: "Oh brave new world that has such people in it."
The separator is: "undefined"
The array has 1 elements: Oh brave new world that has such people in it. /
The original string is: "Jan,Feb,Mar,Apr,May,Jun,Jul,Aug,Sep,Oct,Nov,Dec"
The separator is: ","
The array has 12 elements: Jan / Feb / Mar / Apr / May / Jun / Jul / Aug / Sep / Oct / Nov / Dec /
2.22、startsWith()
2.22.1、概述
startsWith() 方法用来判断当前字符串是否以另外一个给定的子字符串开头,并根据判断结果返回 true 或 false。
2.22.2、语法
str.startsWith(searchString[, position])
2.22.3、参数
searchString
要搜索的子字符串。
position 【可选】
在 str 中搜索 searchString 的开始位置,默认值为 0。
2.22.4、返回值
如果在字符串的开头找到了给定的字符则返回true;否则返回false。
2.22.5、示例
var str = "To be, or not to be, that is the question.";
alert(str.startsWith("To be")); // true
alert(str.startsWith("not to be")); // false
alert(str.startsWith("not to be", 10)); // true
2.22.6、向下兼容
if (!String.prototype.startsWith) {
Object.defineProperty(String.prototype, "startsWith", {
value: function (search, pos) {
pos = !pos || pos < 0 ? 0 : +pos;
return this.substring(pos, pos + search.length) === search;
}
});
}
2.23、substring()
2.23.1、概述
substring() 方法返回一个字符串在开始索引到结束索引之间的一个子集,或从开始索引直到字符串的末尾的一个子集。
2.23.2、语法
str.substring(indexStart[, indexEnd])
2.23.3、参数
indexStart
需要截取的第一个字符的索引,该索引位置的字符作为返回的字符串的首字母。
indexEnd
可选。一个 0 到字符串长度之间的整数,以该数字为索引的字符不包含在截取的字符串内。
2.23.4、返回值
包含给定字符串的指定部分的新字符串。
2.23.5、示例
var anyString = "Mozilla";
// 输出 "Moz"
console.log(anyString.substring(0, 3));
console.log(anyString.substring(3, 0));
console.log(anyString.substring(3, -3));
console.log(anyString.substring(3, NaN));
console.log(anyString.substring(-2, 3));
console.log(anyString.substring(NaN, 3));
// 输出 "lla"
console.log(anyString.substring(4, 7));
console.log(anyString.substring(7, 4));
// 输出 ""
console.log(anyString.substring(4, 4));
// 输出 "Mozill"
console.log(anyString.substring(0, 6));
// 输出 "Mozilla"
console.log(anyString.substring(0, 7));
console.log(anyString.substring(0, 10));
2.24、toLocaleLowerCase()
2.24.1、概述
toLocaleLowerCase()方法根据任何指定区域语言环境设置的大小写映射,返回调用字符串被转换为小写的格式。
2.24.2、语法
str.toLocaleLowerCase()
str.toLocaleLowerCase(locale)
str.toLocaleLowerCase([locale, locale, ...])
2.24.3、参数
locale 【可选】
参数 locale 指明要转换成小写格式的特定语言区域。 如果以一个数组 Array 形式给出多个 locales, 最合适的地区将被选出来应用(参见best available locale)。默认的 locale 是主机环境的当前区域 (locale) 设置。
2.24.4、返回值
根据任何特定于语言环境的案例映射规则将被调用字串转换成小写格式的一个新字符串。
2.24.5、异常
- 如果locale参数不是有效的语言标记,则抛出RangeError (“invalid language tag: xx yy”)。
- 如果数组元素不是string类型,则抛出TypeError(“locale参数中的无效元素”)。
2.24.6、示例
"ALPHABET".toLocaleLowerCase(); // 'alphabet'
"\u0130".toLocaleLowerCase("tr") === "i"; // true
"\u0130".toLocaleLowerCase("en-US") === "i"; // false
let locales = ["tr", "TR", "tr-TR", "tr-u-co-search", "tr-x-turkish"];
"\u0130".toLocaleLowerCase(locales) === "i"; // true
2.25、toLocaleUpperCase()
2.25.1、概述
toLocaleUpperCase() 方法根据本地主机语言环境把字符串转换为大写格式,并返回转换后的字符串。
2.25.2、语法
str.toLocaleUpperCase()
str.toLocaleUpperCase(locale)
str.toLocaleUpperCase([locale, locale, ...])
2.25.3、参数
locale 【可选】
参数 locale 指明要转换成大写格式的特定语言区域。 如果以一个数组 Array 形式给出多个 locales, 最合适的地区将被选出来应用。默认的 locale 是主机环境的当前区域 (locale) 设置。
2.25.4、返回值
根据任何特定于语言环境的案例映射规则将被调用字串转换成大写格式的一个新字符串。
2.25.5、异常
- 如果locale参数不是有效的语言标记,则抛出RangeError (“invalid language tag: xx yy”)。
- 如果数组元素不是string类型,则抛出TypeError(“locale参数中的无效元素”)。
2.25.6、示例
"alphabet".toLocaleUpperCase(); // 'ALPHABET'
"Gesäß".toLocaleUpperCase(); // 'GESÄSS'
"i\u0307".toLocaleUpperCase("lt-LT"); // 'I'
let locales = ["lt", "LT", "lt-LT", "lt-u-co-phonebk", "lt-x-lietuva"];
"i\u0307".toLocaleUpperCase(locales); // 'I'
2.26、toLowerCase()
2.26.1、概述
toLowerCase() 会将调用该方法的字符串值转为小写形式,并返回。
2.26.2、语法
str.toLowerCase()
2.26.3、返回值
一个新的字符串,表示转换为小写的调用字符串。
2.26.4、示例
console.log('中文简体 zh-CN || zh-Hans'.toLowerCase());
// 中文简体 zh-cn || zh-hans
console.log( "ALPHABET".toLowerCase() );
// "alphabet"
2.27、toString()
2.27.1、概述
toString() 方法返回指定对象的字符串形式。
2.27.2、语法
str.toString()
2.27.3、返回值
一个表示调用对象的字符串。
2.27.4、示例
var x = new String("Hello world");
alert(x.toString()); // 输出 "Hello world"
2.28、toUpperCase()
2.28.1、概述
toUpperCase() 方法将调用该方法的字符串转为大写形式并返回(如果调用该方法的值不是字符串类型会被强制转换)。
2.28.2、语法
str.toUpperCase()
2.28.3、返回值
一个新的字符串,表示转换为大写的调用字符串。
2.28.4、异常
TypeError(类型错误):在 null 或 undefined类型上调用,例如:String.prototype.toUpperCase.call(undefined).
2.28.5、示例
console.log("alphabet".toUpperCase()); // 'ALPHABET'
2.29、trim()
2.29.1、概述
trim() 方法会从一个字符串的两端删除空白字符。在这个上下文中的空白字符是所有的空白字符 (space, tab, no-break space 等) 以及所有行终止符字符(如 LF,CR 等)。
2.29.2、语法
str.trim()
2.29.3、返回值
一个代表调用字符串两端去掉空白的新字符串。
2.29.4、示例
var orig = " foo ";
console.log(orig.trim()); // 'foo'
// 另一个 .trim() 例子,只从一边删除
var orig = "foo ";
console.log(orig.trim()); // 'foo'
2.29.5、向下兼容
if (!String.prototype.trim) {
String.prototype.trim = function () {
return this.replace(/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g, '');
};
}
2.30、trimRight()
2.30.1、概述
trimEnd() 方法从一个字符串的末端移除空白字符。trimRight() 是这个方法的别名。
2.30.2、语法
str.trimEnd();
str.trimRight();
2.30.3、返回值
一个新字符串,表示从调用字串的末(右)端除去空白。
2.30.4、示例
var str = " foo ";
alert(str.length); // 8
str = str.trimRight(); // 或写成 str = str.trimEnd();
console.log(str.length); // 6
console.log(str); // ' foo'
2.31、trimStart()
2.31.1、概述
trimStart() 方法从字符串的开头删除空格。trimLeft() 是此方法的别名。
2.31.2、语法
str.trimStart();
str.trimLeft();
2.31.3、返回值
一个新字符串,表示从其开头(左端)除去空格的调用字符串。
2.31.4、示例
var str = " foo ";
console.log(str.length); // 8
str = str.trimStart(); // 等同于 str = str.trimLeft();
console.log(str.length); // 5
console.log(str); // "foo "
2.32、valueOf()
2.32.1、概述
valueOf() 方法返回 String 对象的原始值
2.32.2、语法
str.valueOf()
2.32.3、返回值
给定string对象的原语值的字符串。
2.32.4、示例
var x = new String("Hello world");
console.log(x.valueOf()); // Displays 'Hello world'
3、实例属性
3.1、length
3.1.1、概述
length 属性表示一个字符串的长度。
3.1.2、描述
静态属性 String.length 返回 1。
3.1.3、示例
var x = "Mozilla";
var empty = "";
console.log("Mozilla is " + x.length + " code units long");
/* "Mozilla is 7 code units long" */
console.log("The empty string is has a length of " + empty.length);
/* "The empty string is has a length of 0" */
后记
如果你感觉文章不咋地
//(ㄒoㄒ)//
,就在评论处留言,作者继续改进;o_O???
如果你觉得该文章有一点点用处,可以给作者点个赞;\\*^o^*//
如果你想要和作者一起进步,可以微信扫描二维码,关注前端老L;~~~///(^v^)\\\~~~
谢谢各位读者们啦(^_^)∠※
!!!