接上一个程序,我们的增加修改的功能还没有实现,只是完成了页面跳转和传参和存放model
接下来我们就来模拟下对数据进行操作
首先说上在页面上出现http -400 报错原因,可能是你带了代了错误的参数或参数名有错,所以出现了400错误,一定要认真检查下你的参数拼写哦
特别提示:在add.jsp页面中不要加上那个id的属性输入框哦,因为添加的时候没有用到id这个属性,如果添加了的话会报错400错滴。
1.add.jsp和update.jsp分别为:也就是添加了将操作提交给某个请求
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <form action="${pageContext.request.contextPath }/student/save.do" method="post"> <table> <tr> <th col colspan="2">学生添加</th> </tr> <tr> <td>姓名:</td> <td><input type="text" name="name"></td> </tr> <tr> <td>年龄:</td> <td><input type="text" name="age"></td> </tr> <tr> <td colspan="2"><input type="submit" value="添加"></td> </tr> </table> </form> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>update</title> </head> <body> <form action="${pageContext.request.contextPath }/student/save.do" method="post"> <table> <tr> <th col colspan="2">学生修改</th> </tr> <tr> <td>姓名:</td> <td><input type="text" name="name" value="${student.name }"></td> </tr> <tr> <td>年龄:</td> <td><input type="text" name="age" value="${student.age }"></td> </tr> <tr> <td><input type="hidden" name="id" value="${student.id}"></td> </tr> <tr> <td colspan="2"><input type="submit" value="确定修改"></td> </tr> </table> </form> </body> </html>
2.在studentController下新增了添加/更新的方法:(因为是模拟的,所以程序在id方面会有点bug哦)
@RequestMapping("/save") public String save(Student student) { if(student.getId()!=0) { Student s= studentList.get(student.getId()-1); s.setName(student.getName()); s.setAge(student.getAge()); }else { studentList.add(student); } return "redirect:/student/list.do "; }
因为更新和新增,就是存在是否有id这个参数了,所以用id来区别使用哪个操作。这里的体现了springmvc对对象的自动封装(这个和struts2有点像,但是用起来更厉害哦,不过你要注意不要把input里的name属性写错了哦)。
还有return "redirect:/student/list.do ";
这个return的是什么呢?因为我们的目的是添加后就执行list()方法,所以就用到了springmvc的内部转发或者重定向了
区别是内部转发路径会发生改变,重定向路径不回发生改变
重定向使用方法就是redirect:+请求的路径
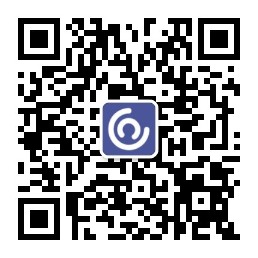
内部转发就是forward:+请求的路径了
3.做到这一步,你们要添加功能试一试用中文name,你会发现有乱码哦,还是springmvc封装了处理乱码的filter
我们直接在web.xml文件里面再贴上以下代码就可以了哦:(这里再敲以下重点哦,这也是个我们的新get到的技能)
<!-- 解决工程编码过滤器 --> <filter> <filter-name>characterEncodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> <init-param> <param-name>forceEncoding</param-name> <param-value>true</param-value> </init-param> </filter> <filter-mapping> <filter-name>characterEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
4.现在我们再添加一个删除操作,修改list.jsp,这里你也会发现我们只是添加了一个连接而已了哦
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>test</title> </head> <body> <a href="${pageContext.request.contextPath }/student/preSave.do">添加学生</a> <table> <tr> <th>编号</th> <th>姓名</th> <th>学生</th> <th>操作</th> </tr> <c:forEach var="student" items="${ studentList}"> <tr> <td>${student.id }</td> <td>${student.name }</td> <td>${student.age }</td> <td> <a href="${pageContext.request.contextPath }/student/preSave.do?id=${student.id}">修改</a> <a href="${pageContext.request.contextPath }/student/delete.do?id=${student.id}">删除</a> </td> </tr> </c:forEach> </table> </body> </html>
5.然后当然还是新增一个新的delete方法来接收对删除操作的请求处理了
@RequestMapping("/delete") public String delete(@RequestParam("id") int id) { studentList.remove(id-1); return "redirect:/student/list.do "; }
删除的时候我们真的是id是必须要知道的了。
做到这里我们再次总结一下:
从中我们学到(使用)了什么呢?
1.springmvc的对象自动封装
2.springmvc的乱码处理过滤器
3.springmvc的内部转发和重定向
最后还是重新贴下总的controller的代码吧:
package com.controller; import java.util.ArrayList; import java.util.List; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.servlet.ModelAndView; import com.entity.Student; @Controller @RequestMapping("/student") public class StudentController { public static List<Student> studentList = new ArrayList<Student>(); static { studentList.add(new Student(1,"zhu",15)); studentList.add(new Student(2,"芳芳",16)); studentList.add(new Student(3,"咩咩",17)); } @RequestMapping("/list") public ModelAndView list() { ModelAndView mav = new ModelAndView(); mav.setViewName("student/list"); mav.addObject("studentList", studentList); return mav; } @RequestMapping("/preSave") public ModelAndView preSave(@RequestParam(value="id",required=false) String id ) { ModelAndView mav = new ModelAndView(); if(id!=null) { mav.addObject("student",studentList.get(Integer.parseInt(id)-1)); mav.setViewName("student/update"); }else { mav.setViewName("student/add"); } return mav; } @RequestMapping("/save") public String save(Student student) { if(student.getId()!=0) { Student s= studentList.get(student.getId()-1); s.setName(student.getName()); s.setAge(student.getAge()); }else { studentList.add(student); } return "redirect:/student/list.do "; } @RequestMapping("/delete") public String delete(@RequestParam("id") int id) { studentList.remove(id-1); return "redirect:/student/list.do "; } }