1.open
open, creat - open and possibly create a file or device
打开一个文件,也可能创建一个文件,返回文件描述符
//头文件
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
//接口
int open(const char *pathname, int flags);
int open(const char *pathname, int flags, mode_t mode);
open有两套接口可供使用,下面介绍一下open的参数:
- pathname:文件路径(当前文件夹为默认路径)。
- flags:文件的打开方式;包括O_RDONLY(只读)、O_WRONLY(只写)、O_RDWR(读写)、O_APPEND(追加)、O_TRUNC(清空)。
- mode:八进制权限,如0666表示(rw-rw-rw-),mode一般配合umask函数使用。
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
int main()
{
umask(0);
int fd = open("log.txt", O_WRONLY | O_CREAT, 0666);
printf("open success, fd: %d\n", fd);
return 0;
}
执行程序之后,就在当前路径下创建了一个权限为rw-rw-rw的log.txt。
系统中也有默认的umask,如果程序中设置umask值就会使用程序中设置的(就近原则)。
2.close
关闭文件
//头文件
#include <unistd.h>
//接口
int close(int fd);
RETURN VALUE:
close() returns zero on success. On error, -1 is returned, and errno is set appropriately.
3.write
write - write to a file descriptor
//头文件
#include <unistd.h>
//接口
ssize_t write(int fd, const void *buf, size_t count);
现在我们已经打开了一个文件,那么直接使用write向fd中写入:
const char* str = "hello write\n";
ssize_t s = write(fd, str, strlen(str));
log.txt:
扫描二维码关注公众号,回复:
14615025 查看本文章
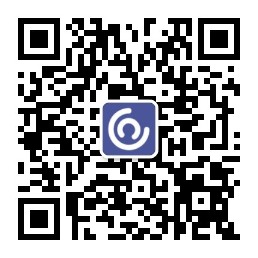
write时要注意打开方式,打开时可以是O_APPEND,也可以是O_TRUNC。
现在log.txt已经写入了"hello write",那么我们看一下O_APPEND和O_TRUNC的不同点:
O_APPEND:
O_TRUNC:
open函数的第二个参数加上O_APPEND选项后,写入的数据会在文件的末尾追加;加上O_TRUNC之后打开文件会先清空文件,然后再向文件中写入。
面试题
写一段程序,清空文件中的数据。
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd = open("log.txt", O_RDWR | O_TRUNC);
close(fd);
return 0;
}
4.read
read - read from a file descriptor
//头文件
#include <unistd.h>
//接口
ssize_t read(int fd, void *buf, size_t count);
使用read函数时open函数的第二个参数flags需要改变,O_WRONLY需要改为O_RDONLY或者O_RDWR;这样才能在文件中读取数据。
int main()
{
umask(0);
int fd = open("log.txt", O_RDWR | O_CREAT, 0666);
// const char* str = "aa\n";
// ssize_t s = write(fd, str, strlen(str));
printf("open success, fd: %d\n", fd);
char buffer[64];
memset(buffer, '\0', sizeof(buffer));
read(fd, buffer, sizeof(buffer));
printf("%s\n", buffer);
close(fd);
return 0;
}
把文件中的数据读取到buffer中: