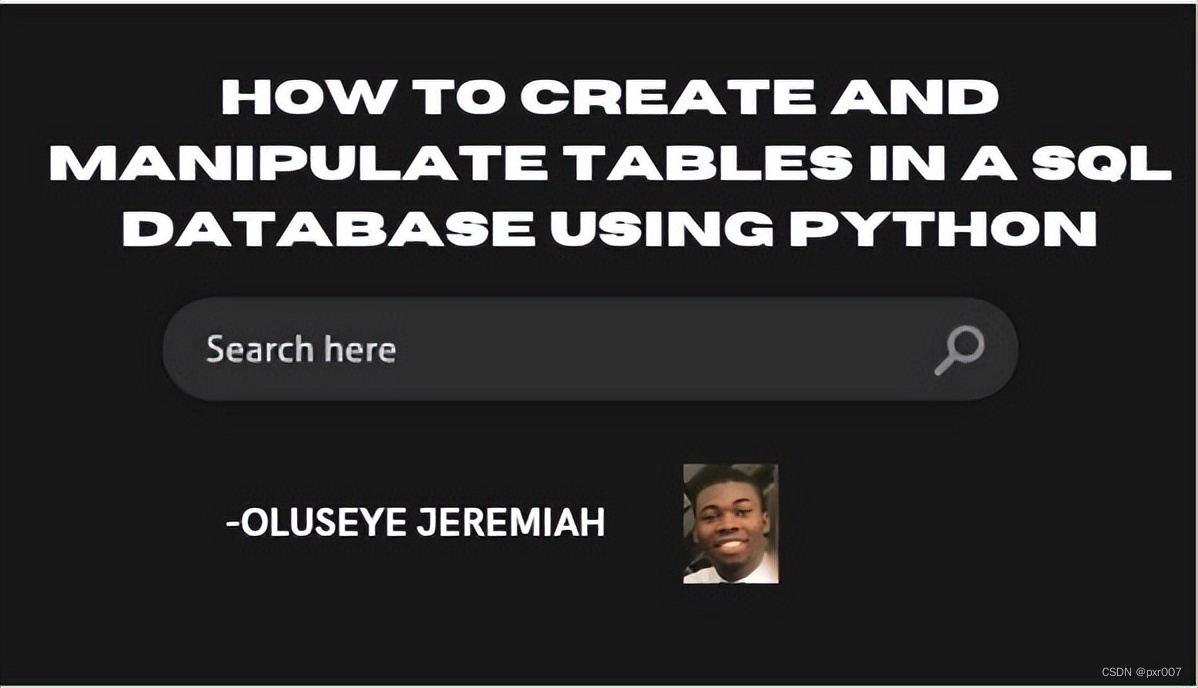
Python 是一种功能强大的编程语言,可用于与 SQL 数据库进行交互。在 Python 的帮助下,您可以创建、操作 SQL 数据库中的表并与之交互。
在本教程中,我们将讨论如何使用 Python 在 SQL 数据库中创建和操作表。
先决条件:
要学习本教程,您需要具备以下条件:
Python 的应用知识。
SQL 数据库。对于本教程,我们将使用 SQLite。
如何使用 Python 在 SQL 数据库中创建表
要使用 Python 在 SQL 数据库中创建表,我们首先需要建立与数据库的连接。对于本教程,我们将使用 SQLite 数据库。如何在 Windows 中禁用(和启用)用户帐户SQLite 是一个轻量级的无服务器数据库,非常适合小型项目。
要连接到 SQLite 数据库,我们将使用 Python 中内置的 SQLite3 模块。
下面是使用 Python 在我们的 SQLite 数据库中创建表的代码:
import sqlite3
# connect to the database
conn = sqlite3.connect('example.db')
# create a cursor object
c = conn.cursor()
# create a table
c.execute('''CREATE TABLE employees
(id INT PRIMARY KEY NOT NULL,
name TEXT NOT NULL,
age INT NOT NULL)''')
# save the changes
conn.commit()
# close the connection
conn.close()
connect在上面的代码中,我们首先使用模块的方法建立了与SQLite数据库的连接sqlite3。然后我们使用该方法创建了一个游标对象cursor。
接下来,我们执行 SQL 查询以创建一个名为employees三列的表:id、name和age。该id列被设置为主键,这意味着表中的每条记录都将具有唯一的id值。和列设置为,name这意味着它们不能为空。ageNOT NULL
最后,我们使用 方法保存更改commit并使用 方法关闭连接close。
如何向表中插入数据
现在我们已经创建了一个表,我们可以向其中插入数据。下面是向表中插入数据的代码employees:
import sqlite3
# connect to the database
conn = sqlite3.connect('example.db')
# create a cursor object
c = conn.cursor()
# insert data into the table
c.execute("INSERT INTO employees (id, name, age) VALUES (1, 'John Doe', 30)")
c.execute("INSERT INTO employees (id, name, age) VALUES (2, 'Jane Doe', 25)")
# save the changes
conn.commit()
# close the connection
conn.close()
在上面的代码中,我们employees使用execute方法向表中插入了两条记录。我们传入了两个 SQL 查询,每条记录一个。
如何从表中选择数据
现在我们已经将数据插入到employees表中,我们可以使用语句检索它SELECT。这是从表中选择数据的代码employees:
import sqlite3
# connect to the database
conn = sqlite3.connect('example.db')
# create a cursor object
c = conn.cursor()
# select data from the table
c.execute("SELECT * FROM employees")
# fetch all the records
records = c.fetchall()
# print the records
for record in records:
print(record)
# close the connection
conn.close()
employees在上面的代码中,我们使用语句从表中选择了所有记录SELECT。然后我们使用该方法获取所有记录fetchall并使用 for 循环打印它们。
如何更新表中的数据
要更新表中的数据,我们使用UPDATE语句。下面是更新表中数据的代码employees:
import sqlite3
# connect to the database
conn = sqlite3.connect('example.db')
# create a cursor object
c = conn.cursor()
# update data in the table
c.execute("UPDATE employees SET age = 35 WHERE name = 'John Doe'")
# save the changes
conn.commit()
# select data from the table
c.execute("SELECT * FROM employees")
# fetch all the records
records = c.fetchall()
# print the records
for record in records:
print(record)
# close the connection
conn.close()
在上面的代码中,我们使用语句将名称为“John Doe”的记录的年龄更新为 35 UPDATE。然后我们使用该方法保存更改commit。
如何从表中删除数据
要从表中删除数据,我们使用DELETE语句。下面是从表中删除数据的代码employees:
import sqlite3
# connect to the database
conn = sqlite3.connect('example.db')
# create a cursor object
c = conn.cursor()
# delete data from the table
c.execute("DELETE FROM employees WHERE name = 'Jane Doe'")
# save the changes
conn.commit()
# select data from the table
c.execute("SELECT * FROM employees")
# fetch all the records
records = c.fetchall()
# print the records
for record in records:
print(record)
# close the connection
conn.close()
employees在上面的代码中,我们使用语句从表中删除了名为“Jane Doe”的记录DELETE。然后我们使用该方法保存更改commit。
结论
在本文中,我们讨论了如何使用 Python 在 SQL 数据库中创建和操作表。
我们介绍了如何创建表、向其中插入数据、从中选择数据、更新其中的数据以及从中删除数据。我们还提供了完成这些任务所需的代码。
按照本教程中概述的步骤,您可以使用 Python 在任何 SQL 数据库中创建和操作表。