闲话少叙,直接上干货,
生成的图表的数据是从数据库中查询出来的,有需要的朋友请自己调整一下
package test;
import com.lowagie.text.Document;
import com.lowagie.text.DocumentException;
import com.lowagie.text.Rectangle;
import com.lowagie.text.pdf.*;
import org.apache.commons.math3.distribution.NormalDistribution;
import org.apache.commons.math3.stat.descriptive.moment.Mean;
import org.apache.commons.math3.stat.descriptive.moment.Variance;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.chart.plot.XYPlot;
import org.jfree.chart.renderer.xy.XYLineAndShapeRenderer;
import org.jfree.data.xy.XYDataset;
import org.jfree.data.xy.XYSeries;
import org.jfree.data.xy.XYSeriesCollection;
import java.awt.*;
import java.awt.geom.Rectangle2D;
import java.io.*;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Random;
public class TestSaveChartAsPDF {
static int size = 148612;
/**
* Saves a chart to a PDF file.
*
* @param file
* the file.
* @param chart
* the chart.
* @param width
* the chart width.
* @param height
* the chart height.
*/
public static void saveChartAsPDF(File file, JFreeChart chart, int width,int height, FontMapper mapper) throws IOException {
OutputStream out = new BufferedOutputStream(new FileOutputStream(file));
writeChartAsPDF(out, chart, width, height, mapper);
out.close();
}
/**
* Writes a chart to an output stream in PDF format.
*
* @param out
* the output stream.
* @param chart
* the chart.
* @param width
* the chart width.
* @param height
* the chart height.
*
*/
public static void writeChartAsPDF(OutputStream out, JFreeChart chart,
int width, int height, FontMapper mapper) throws IOException {
Rectangle pagesize = new Rectangle(width, height);
Document document = new Document(pagesize, 50, 50, 50, 50);
try {
PdfWriter writer = PdfWriter.getInstance(document, out);
document.addAuthor("JFreeChart");
document.addSubject("Demonstration");
document.open();
PdfContentByte cb = writer.getDirectContent();
PdfTemplate tp = cb.createTemplate(width, height);
Graphics2D g2 = tp.createGraphics(width, height, mapper);
Rectangle2D r2D = new Rectangle2D.Double(0, 0, width, height);
chart.draw(g2, r2D);
g2.dispose();
cb.addTemplate(tp, 0, 0);
} catch (DocumentException de) {
System.err.println(de.getMessage());
}
document.close();
}
/**
* Creates a dataset, consisting of two series of monthly data. * *
*
* @return the dataset.
*/
public static XYDataset createDataset() {
Random random = new Random();
NormalDistribution normalDistribution = new NormalDistribution();
XYSeries s1 = new XYSeries("正态分布");
//double s1 = normalDistribution.cumulativeProbability(常数项+变量1赋值x变量1系数+...+变量N赋值x变量N系数);
//均值为0,方差为1的随机数
//random.nextGaussian()
//均值为a,方差为b的随机数
//Math.sqrt(b)*random.nextGaussian()+a;
//随机产生高斯数进行正太分布
/*for(int i=1;i<=1000;i++){
double a = random.nextGaussian();
double c = ((double)1/Math.sqrt(Math.PI*2))*(Math.pow(Math.E,-(double)a*a/2));
s1.add(a, c);
}
*/
List<Long> list = getResponseTime();
Date d1 = new Date();
double average = 10d;//getAverage(list);
double variance = 20d;//getVariance(list,average);
System.out.println("average ========"+average);
System.out.println("variance ========"+variance);
//--------------------------------
double[] values = new double[list.size()];
for(int i=0;i<list.size();i++){
values[i]=list.get(i);
}
Mean mean2 = new Mean(); // 算术平均值
Variance variance2 = new Variance();// 方差
double mean = mean2.evaluate(values);
System.out.println("数据公共包mean: " + mean);
System.out.println("数据公共包variance:" + variance2.evaluate(values));
variance2.setBiasCorrected(false);
double varianceV = variance2.evaluate(values);
double stdDev = Math.sqrt(varianceV);
System.out.println("数据公共包variance:" + varianceV);
Date d2 = new Date();
System.out.println("计算耗时:==========="+(d2.getTime()-d1.getTime()));
//--------------------------------
double prop = 0d;
for(int i=0;i<list.size();i++){
double a = (double)list.get(i);
double c = ((double)1/(Math.sqrt(Math.PI*2)*variance))*(Math.pow(Math.E,-Math.pow(((double)a-average),2)/(2*variance*variance)));
s1.add(a, c);
if(a >= (mean+stdDev))
prop = a * c;
}
System.out.println("大于均值加方差的总概率:==========="+prop);
//TimeSeriesCollection dataset = new TimeSeriesCollection();
XYSeriesCollection dataset = new XYSeriesCollection();
dataset.addSeries(s1);
/* dataset.addSeries(s2);
dataset.addSeries(s3); */
return dataset;
}
public static List<Long> getResponseTime(){
//String sql ="SELECT elapsed FROM keeper_web_s10_span_201707_t WHERE accepted_Time BETWEEN 1499875200000 AND 1500480000000 ;";
String sql ="SELECT elapsed FROM keeper_web_801_span_201708_t UNION ALL SELECT elapsed FROM keeper_web_801_span_exception_201708_t UNION ALL SELECT elapsed FROM keeper_web_801_span_fault_201708_t;";
DBHelper db1 = new DBHelper(sql);
List<Long> list = new ArrayList<Long>();
try {
ResultSet rst = db1.pst.executeQuery();
while(rst.next()){
Long responseTime = rst.getLong(1);
list.add(responseTime);
}
rst.close();
db1.close();
} catch (SQLException e) {
e.printStackTrace();
}
System.out.println("list.length======="+list.size());
System.out.println(list.get(0));
System.out.println(list.get(1));
return list;
}
//取均值
public static double getAverage(List<Long> l){
long sum = 0;
for(int i=0;i<l.size();i++){
sum = sum + l.get(i);
}
double result = (double)sum/l.size();
return result;
}
//取方差
public static double getVariance(List<Long> l,double average){
double sum = 0;
for(int i=0;i<l.size();i++){
sum = sum + Math.pow(((double)l.get(i)-average),2);
}
double result = sum/l.size();
//return Math.sqrt(result);
return result;
}
public static void main(String[] args) {
try {
// create a chart...
XYDataset dataset = createDataset();
//JFreeChart chart = ChartFactory.createTimeSeriesChart("Legal & General Unit Trust Prices", "Date","Price Per Unit", dataset, true, true, false);
// some additional chart customisation here...
JFreeChart chart = ChartFactory.createXYLineChart("Keeper 10 Days Span Normal Distribution", "X:response_time(ms)",
"Y:probability", dataset, PlotOrientation.VERTICAL, true, true,
false);
XYPlot plot = chart.getXYPlot();
XYLineAndShapeRenderer renderer = new XYLineAndShapeRenderer();
renderer.setSeriesPaint(0, Color.GREEN);
plot.setRenderer(2, renderer);
// XYLineAndShapeRenderer renderer = (XYLineAndShapeRenderer) plot.getRenderer();
// renderer.setShapesVisible(true);
// DateAxis axis = (DateAxis) plot.getDomainAxis();
// axis.setDateFormatOverride(new SimpleDateFormat("MMM-yyyy"));
// write the chart to a PDF file...
File file = new File("");
File fileName = new File(file.getCanonicalPath()+"\\src\\test\\"+"jfreechart_8011.pdf");
saveChartAsPDF(fileName, chart, 800, 600, new DefaultFontMapper());
} catch (IOException e) {
System.out.println(e.getMessage());
}
}
}
其中用到了三个jar包:
jfreechart-1.0.19.jar
iText-2.1.7.jar
commons-math3-3.0.jar
生成图片如下图:
收工
扫描二维码关注公众号,回复:
14773846 查看本文章
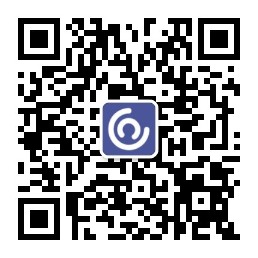