百度了一圈,csv大都是导出和下载分开的,然后根据别人的博客改了改,在此感谢大佬们的付出
js以及jsp
<a href="#" onclick="exportData()" class="easyui-linkbutton"
iconCls="icon-save">导出</a>
function exportData(){
location.href=basePath +
"/exportAction.do?action=export";
}
CSV工具包(自己改成了傻瓜式拿来即用的东西)
/**
* @param response
* @param map 对应的列标题
* @param exportData 需要导出的数据
* @param fileds 列标题对应的实体类的属性
* @throws IOException
*/
@SuppressWarnings({ "resource", "rawtypes", "unchecked" })
public static void exportFile(HttpServletResponse response,
HashMap map, List exportData, String fileds[])
throws IOException {
try {
File tempFile = File.createTempFile("vehicle", ".csv");
BufferedWriter csvFileOutputStream = null;
csvFileOutputStream = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(tempFile), "GBK"), 1024);
for (Iterator propertyIterator = map.entrySet().iterator(); propertyIterator.hasNext();) {
java.util.Map.Entry propertyEntry = (java.util.Map.Entry) propertyIterator.next();
csvFileOutputStream.write((String) propertyEntry.getValue() != null ? new String(((String) propertyEntry.getValue()).getBytes("GBK"), "GBK") : "");
if (propertyIterator.hasNext()) {
csvFileOutputStream.write(",");
}
}
csvFileOutputStream.write("\r\n");
for (int j = 0; exportData != null && !exportData.isEmpty()
&& j < exportData.size(); j++) {
Class clazz = exportData.get(j).getClass();
String[] contents = new String[fileds.length];
for (int i = 0; fileds != null && i < fileds.length; i++) {
String filedName = toUpperCaseFirstOne(fileds[i]);
Object obj = null;
try {
Method method = clazz.getMethod(filedName);
method.setAccessible(true);
obj = method.invoke(exportData.get(j));
} catch (Exception e) {
}
String str = String.valueOf(obj);
if (str == null || str.equals("null"))
str = "";
contents[i] = str;
}
for (int n = 0; n < contents.length; n++) {
csvFileOutputStream.write(contents[n]);
csvFileOutputStream.write(",");
}
csvFileOutputStream.write("\r\n");
}
csvFileOutputStream.flush();
/**
* 写入csv结束,写出流
*/
java.io.OutputStream out = response.getOutputStream();
byte[] b = new byte[10240];
java.io.File fileLoad = new java.io.File(tempFile.getCanonicalPath());
response.reset();
response.setContentType("application/csv");
String trueCSVName="导出数据.csv";
response.setHeader("Content-Disposition", "attachment; filename="+ new String(trueCSVName.getBytes("GBK"), "ISO8859-1"));
long fileLength = fileLoad.length();
String length1 = String.valueOf(fileLength);
response.setHeader("Content_Length", length1);
java.io.FileInputStream in = new java.io.FileInputStream(fileLoad);
int n;
while ((n = in.read(b)) != -1) {
out.write(b, 0, n);
}
in.close();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 将第一个字母转换为大写字母并和get拼合成方法
*
* @param origin
* @return
*/
private static String toUpperCaseFirstOne(String origin) {
StringBuffer sb = new StringBuffer(origin);
sb.setCharAt(0, Character.toUpperCase(sb.charAt(0)));
sb.insert(0, "get");
return sb.toString();
}
然后贴一下servlet调用的方法吧
List<RealTimeSO2> exportData = new ArrayList<RealTimeSO2>();
String xhLxComb="output";
String startTime="2017-08-02 09:01:44";
String gnId="21";
exportData = new SO2DataSelService().listSO2Data(xhLxComb, gnId, startTime);
HashMap map = new LinkedHashMap();
map.put("1", "第一列");
map.put("2", "第二列");
map.put("3", "第三列");
map.put("4", "第四列");
String fileds[] = new String[] { "facName", "jzName" , "xhLx","recDate","so2" };
CVSUtils.exportFile(response, map, exportData, fileds);
最后来个图
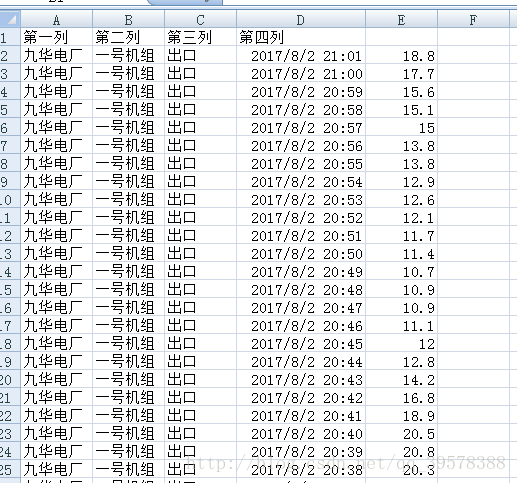
这个真的是最后了
jsp
<a href="#" onclick="exportData()" class="easyui-linkbutton" iconCls="icon-save">导出</a>
js
/**
* 导出功能
*/
function exportData() {
var exportURL=basePath + "/sO2DataSelAction.do?action=export";
var temp1=$("#xhLxComb").combobox('getValue');
var temp2=$("#gnCombobox").combobox('getValue');
var temp3=$('#startTime').datetimebox('getValue');
/**
* 使用form表单来发送请求
* 1.method属性用来设置请求的类型——post还是get
*2.action属性用来设置请求路径
*3.后台可以根据request.getParameter(XXX)来获取对应name的值。
*
*/
var form = $("<form>");
form.attr("style", "display:none");
form.attr("target", "");
form.attr("method", "post");
form.attr("action", exportURL);
var input1 = $("<input>");
input1.attr("type", "hidden");
input1.attr("name", "temp1");
input1.attr("value", temp1);
form.append(input1);
var input2 = $("<input>");
input2.attr("type", "hidden");
input2.attr("name", "temp2");
input2.attr("value", temp2);
form.append(input2);
var input2 = $("<input>");
input2.attr("type", "hidden");
input2.attr("name", "temp3");
input2.attr("value", temp3);
form.append(input2);
$("body").append(form);
form.submit();
form.remove();
}