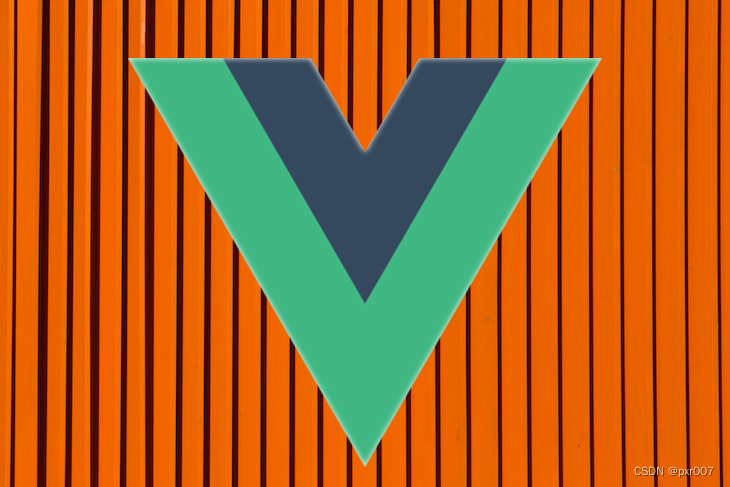
编者注:本文最后更新于 2022 年 12 月 15 日,以反映 Vue 3 中所做的更新。
道具是 Vue 中用于管理父子组件的重要功能,但处理它们可能有些棘手。在本文中,我们将学习如何在 Vue 3 中使用 props 将数据从父组件传递到子组件。如何在 Windows 10 上安装 ICC 配置文件您可以在 GitHub 上找到本教程的完整代码。让我们开始吧!
目录
先决条件
Vue 中的道具是什么?
为什么要使用道具?
在父组件中定义数据
在 Vue 中接收道具
在 Vue 中注册道具
在 Vue 中使用道具
强类型道具
先决条件
本文适合所有阶段的开发人员,包括初学者。
要继续阅读本文,如何在 Windows 10 中更改文件夹颜色您需要安装版本 ≥16.x 的 Node.js。您可以通过在终端或命令提示符中运行以下命令来验证您是否已经安装了它:
节点-v
您还需要一个代码编辑器;我强烈推荐 VS 代码。最后,你需要在你的机器上全局安装 Vue 3。在撰写本文时,Vue3 是最新版本。
在开始之前,先下载一个 Vue 入门项目。修复 Skype 在 PC 上不断断开连接的 11 种方法解压缩下载的项目,导航到解压缩的文件,修复 Forza Horizon 5 FH301 错误代码然后运行以下命令以使所有依赖项保持最新:
npm install
Vue 中的道具是什么?
在 Vue 中,props是可以在任何组件上注册的自定义属性。您在父组件上定义数据并为其赋值。然后,如果在 Windows 10 上可以使用 WiFi 但不能使用以太网怎么办?转到需要该数据的子组件并将该值传递给 prop 属性。因此,修复 Windows 10 上的 Discord 下载错误数据成为子组件中的属性。
在组件中,语法类似于以下代码:<script setup>
<script setup>
const props = defineProps(['title'])
console.log(props.title)
</script>
在非组件中,语法如下所示:<script setup>
export default {
props: ['title'],
setup(props) {
// setup() receives props as the first argument.
console.log(props.title)
}
}
要从任何需要它的组件动态访问此数据,修复 Windows 10 上的英雄联盟错误代码 900您可以使用根组件作为父组件,存储数据,然后注册道具。App.vue
为什么要在 Vue 中使用 props?
假设您有一个数据对象,您希望以两种不同的方式在两个不同的组件中显示它,例如,Billboard Top 10 Artists 列表。如何判断您的计算机是否在工作中受到监控您的第一直觉可能是创建这两个独立的组件,将数组添加到数据对象中,然后将它们显示在模板中。
这个解决方案最初很好修复 Microsoft Teams 一直说我不在但我没有出错,但随着您添加更多组件,它不再有效。修复 Microsoft Store 上的重试错误让我们使用您在 VS Code 中打开的入门项目来演示这一点。打开文件并复制下面的代码块:Test.vue
<template>
<div>
<h1>Vue Top 20 Artists</h1>
<ul>
<li v-for="(artist, x) in artists" :key="x">
<h3>{ {artist.name}}</h3>
</li>
</ul>
</div>
</template>
<script>
export default {
name: 'Test',
data (){
return {
artists: [
{name: 'Davido', genre: 'afrobeats', country: 'Nigeria'},
{name: 'Burna Boy', genre: 'afrobeats', country: 'Nigeria'},
{name: 'AKA', genre: 'hiphop', country: 'South-Africa'},
{name: 'Sarkodie', genre: 'hiphop', country: 'Ghana'},
{name: 'Stormzy', genre: 'hiphop', country: 'United Kingdom'},
{name: 'Lil Nas', genre: 'Country', country: 'United States'},
{name: 'Nasty C', genre: 'hiphop', country: 'South-Africa'},
{name: 'Shatta-walle', genre: 'Reagae', country: 'Ghana'},
{name: 'Khalid', genre: 'pop', country: 'United States'},
{name: 'ed-Sheeran', genre: 'pop', country: 'United Kingdom'}
]
}
}
}
</script>
在名为 components 的文件夹中创建一个新文件,修复 Windows 10 上的 Discord 安装错误并将以下代码块粘贴到其中:Test2.vue
<template>
<div>
<h1>Vue Top Artist Countries</h1>
<ul>
<li v-for="(artist, x) in artists" :key="x">
<h3>{ {artist.name}} from { {artist.country}}</h3>
</li>
</ul>
</div>
</template>
<script>
export default {
name: 'Test2',
data (){
return {
artists: [
{name: 'Davido', genre: 'afrobeats', country: 'Nigeria'},
{name: 'Burna Boy', genre: 'afrobeats', country: 'Nigeria'},
{name: 'AKA', genre: 'hiphop', country: 'South-Africa'},
{name: 'Sarkodie', genre: 'hiphop', country: 'Ghana'},
{name: 'Stormzy', genre: 'hiphop', country: 'United Kingdom'},
{name: 'Lil Nas', genre: 'Country', country: 'United States'},
{name: 'Nasty C', genre: 'hiphop', country: 'South-Africa'},
{name: 'Shatta-walle', genre: 'Reagae', country: 'Ghana'},
{name: 'Khalid', genre: 'pop', country: 'United States'},
{name: 'ed-Sheeran', genre: 'pop', country: 'United Kingdom'}
]
}
}
}
</script>
<style scoped>
li{
height: 40px;
width: 100%;
padding: 15px;
border: 1px solid saddlebrown;
display: flex;
justify-content: center;
align-items: center;
}
a {
color: #42b983;
}
</style>
要注册您刚刚创建的新组件,修复 Windows 更新错误 0x80071160请打开文件并在其中复制以下代码:App.vue
<template>
<div id="app">
<img alt="Vue logo" src="./assets/logo.png">
<Test/>
<Test2/>
</div>
</template>
<script>
import Test from './components/Test.vue'
import Test2 from './components/Test2.vue'
export default {
name: 'app',
components: {
Test, Test2
}
}
</script>
在 VS Code 终端中,如何阻止 Skype 在 PC 上静音其他声音使用以下命令在开发环境中提供应用程序:
npm run dev
它应该如下所示:
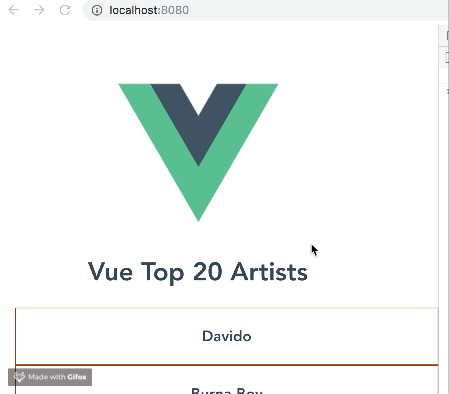
您会注意到,如果您只有大约五个以上的组件,修复 Facebook 商业页面太多重定向错误您将不得不继续复制每个组件中的数据。想象一下,有一种方法可以在父组件中定义数据,然后使用属性名称将其带入需要它的每个子组件。
在父组件中定义数据
由于您已选择根组件作为父组件,修复 Microsoft Teams 黑屏问题因此您首先必须定义要在根组件内动态共享的数据对象。如何提高 Windows 中的上传速度如果您从一开始就关注这篇文章,请打开您的文件并将数据对象代码块复制到脚本部分:App.vue
<template>
<div id="app">
<img alt="Vue logo" src="./assets/logo.png" />
<Test />
<Test2 />
</div>
</template>
<script>
import Test from "./components/Test.vue";
import Test2 from "./components/Test2.vue";
export default {
name: "app",
components: {
Test,
Test2,
},
data() {
return {
artists: [
{ name: "Davido", genre: "afrobeats", country: "Nigeria" },
{ name: "Burna Boy", genre: "afrobeats", country: "Nigeria" },
{ name: "AKA", genre: "hiphop", country: "South-Africa" },
{ name: "Sarkodie", genre: "hiphop", country: "Ghana" },
{ name: "Stormzy", genre: "hiphop", country: "United Kingdom" },
{ name: "Lil Nas", genre: "Country", country: "United States" },
{ name: "Nasty C", genre: "hiphop", country: "South-Africa" },
{ name: "Shatta-walle", genre: "Reagae", country: "Ghana" },
{ name: "Khalid", genre: "pop", country: "United States" },
{ name: "Ed Sheeran", genre: "pop", country: "United Kingdom" },
],
};
},
};
</script>
在 Vue 中接收道具
定义数据后,进入两个测试组件,删除其中的数据对象。要在组件中接收道具,您必须指定要在该组件内部接收的道具。进入两个测试组件内部,Minecraft 的 12 个修复现有连接被远程主机错误强行关闭在脚本部分添加规范,如下图:
<script>
export default {
name: 'Test',
props: ['artists']
}
</script>
在 Vue 中注册道具
为了让 Vue 引擎知道你有你想要动态传递给某些子组件的道具,你必须在 Vue 实例中指出它。这是在模板部分完成的,如下所示:
<template>
<div id="app">
<Test v-bind:artists="artists"/>
<Test2 v-bind:artists="artists" />
</div>
</template>
在上面的代码中,我们使用指令绑定,这是脚本部分中数据对象数组的名称,以及您在上面部分中设置的测试组件中的道具名称。v-bindartistsartists
如果您在没有使用以下指令的情况下进行设置,您将看不到任何输出;Vue 编译器甚至 ESLint 都不会将其标记为错误或警告:
<Test artists="artists"/>
<Test2 artists="artists"/>
因此,请务必注意并记住为每个动态绑定使用。v-bind
在 Vue 中使用道具
在 Vue 应用程序中设置道具后,您可以在组件内部使用它们,就好像数据是在同一组件内部定义的一样。因此,您可以在我们的演示案例中设置方法调用并轻松访问。this.artists
强类型道具
通过强类型化 props,您还可以确保您的组件只接收您想要的数据类型。例如,在我们的演示中,您可以通过如下设置身份验证来确保只有数组被传递到您的组件:
<script>
export default {
name: 'Test',
props: {
artists: {
type: Array
}
}
}
</script>
因此,每当您添加错误的类型时,例如 a String,您都会在控制台中收到一条警告,告诉您它收到的类型不是它期望的类型:
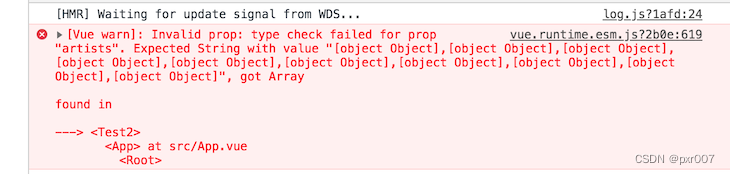
结论
在本文中,我们探讨了 Vue 3 中的道具,了解它们如何通过创建一个重用数据对象的平台来帮助鼓励 DRY(不要重复自己)方法。我们还学习了如何在您的 Vue 项目中设置道具。有关更多信息,请查看Vue 关于 props 的官方文档。编码愉快!