写这篇文章的时候只是接触两天MySQL,目前MySQL的知识也只是学习到基础操作,比如:进行增删查改的步骤等操作,便迫不及待的想要进行连接MySQL数据库进行实际操作
本人属于小白操作,若有问题欢迎大家请及时指正!!
目录
前言
MySQL是一款开源免费的关系型数据库管理系统,最初由瑞典公司MySQL AB公司开发,目前隶属于Oracle。它支持多种操作系统(如Windows、Linux、Mac OS等),提供了高效的数据存储和处理功能,可通过水平和垂直两个方向进行扩展,满足不同规模和需求的应用场景。另外还有多种编程语言接口,如 Java、PHP、Python等,非常便捷。
一、准备工作
1、本人的软件环境:
- MySQL版本:5.7.31
- 数据库可视化工具:Navicat Premium 15
- AndroidStudio:Android Studio Dolphin | 2021.3.1,2
2、如何导入适合自己MySQL的jar包
(1)查看自己电脑上的MySQL版本号:
a. 首先Win+R打开命令提示窗口,输入cmd;
b. 输入mysql --version 回车即可;
(2)下载和自己适配的MySQL的jar包
a. MySQL对应jar包的下载大家可以参考 mysql驱动与数据库及jdk各版本对应关系
b. 官方网址下载 MySQL :: Download MySQL Connector/J (Archived Versions)
下载如图1.1所示。
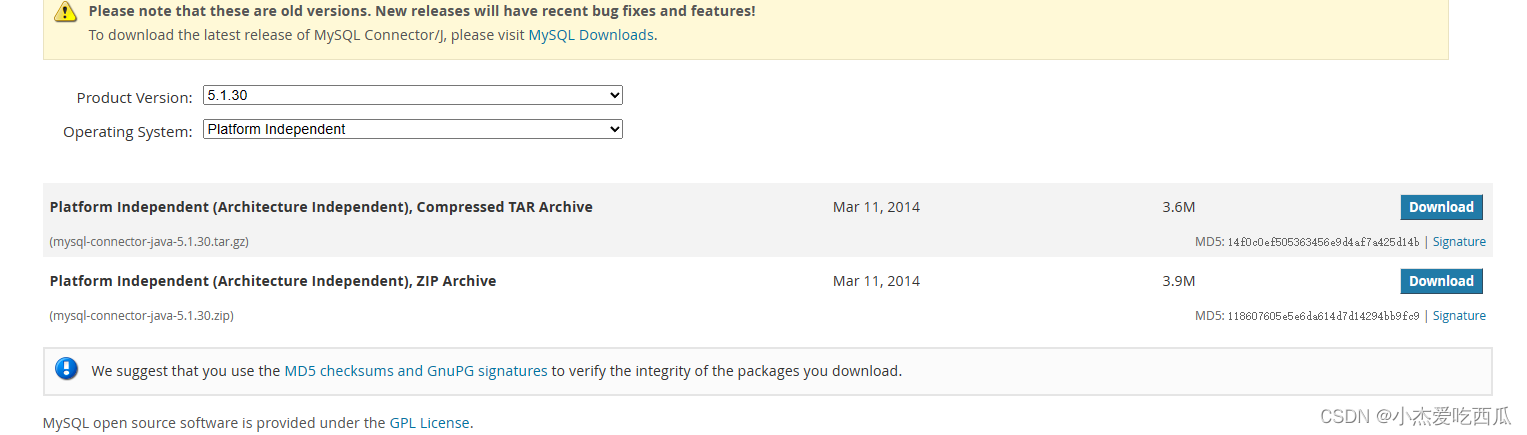
(3)AndroidStudio中导入MySQL的jar包
a. 打开AndroidStudio,切换至project视图下,并将jar包复制到libs目录下如图1.2所示。
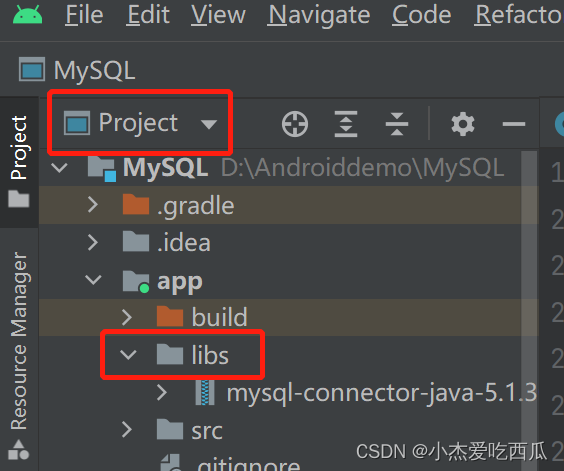
b. 右击add as Library;
二、实际操作步骤(复刻登录)
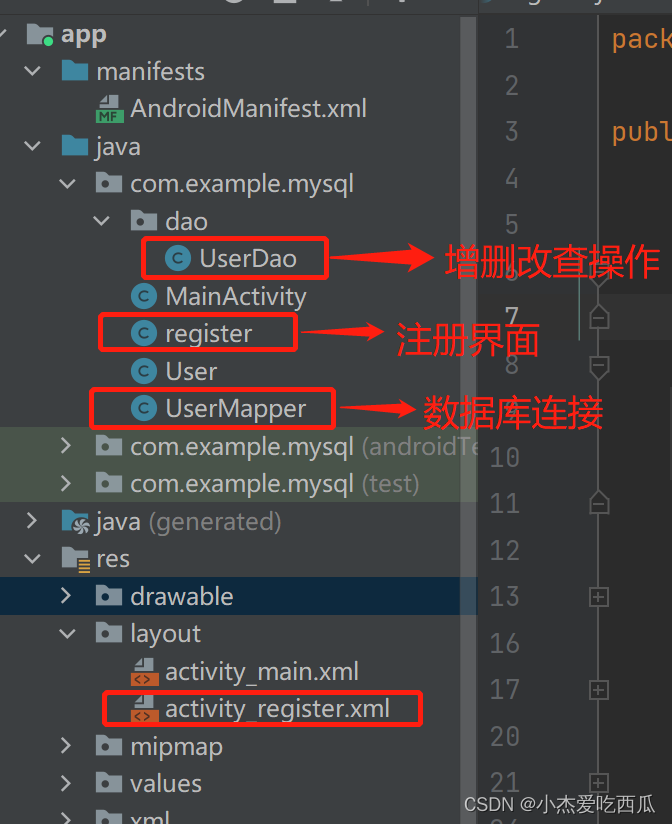
1.新建一个UserMapper类
代码如下:
package com.example.mysql;
import android.util.Log;
import java.sql.Connection;
import java.sql.DriverManager;
public class UserMapper {
private static final String TAG = "mysql-party-JDBCUtils";
private static String driver = "com.mysql.jdbc.Driver";// MySql驱动
private static String dbName = "factory";// 数据库名称
private static String user = "root";// 用户名
private static String password = "请输入你的密码";// 密码
public static Connection getConn() {
Connection connection = null;
try {
Class.forName(driver);// 动态加载类
String ip = "192.168.79.1";// 写成本机地址,不能写成localhost,同时手机和电脑连接的网络必须是同一个
connection = DriverManager.getConnection("jdbc:mysql://" + ip + ":3306/" + dbName,
user, password);
Log.d(TAG, "getConn: 连接成功");
} catch (Exception e) {
e.printStackTrace();
}
return connection;
}
}
2.创建一个实体类
代码如下:
package com.example.mysql;
public class User {
String name ;
String password;
public User(){
}
public User(String name, String password) {
this.name = name;
this.password = password;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
3.创建UserDao类进行增、查操作
package com.example.mysql.dao;
import android.util.Log;
import com.example.mysql.User;
import com.example.mysql.UserMapper;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.HashMap;
public class UserDao {
private static final String TAG = "mysql-factory-UserDao";
HashMap<String, String> map = new HashMap<>();
public int test(String name, String password) {
Connection connection = UserMapper.getConn();
int msg = 0;
try {
// mysql简单的查询语句。这里是根据user表的userAccount字段来查询某条记录
String sql = "select * from enroll where name = ?";
if (connection != null) {// connection不为null表示与数据库建立了连接
//PreparedStatement 用于执行参数化查询
PreparedStatement ps = connection.prepareStatement(sql);
if (ps != null) {
Log.e(TAG, "账号:" + name);
//根据账号进行查询
ps.setString(1, name);
// 执行sql查询语句并返回结果集
ResultSet rs = ps.executeQuery();
int count = rs.getMetaData().getColumnCount();
System.out.println("count的输出是+" + count);
//将查到的内容储存在map里
while (rs.next()) {
// 注意:下标是从1开始的
for (int i = 1; i <= count; i++) {
String field = rs.getMetaData().getColumnName(i);
map.put(field, rs.getString(field));
System.out.println("Map的输出是+" + map);
}
}
connection.close();
ps.close();
if (map.size() != 0) {
StringBuilder s = new StringBuilder();
//寻找密码是否匹配
for (String key : map.keySet()) {
if (key.equals("password")) {
if (password.equals(map.get(key))) {
msg = 1; //密码正确
} else
msg = 2; //密码错误
break;
}
}
} else {
Log.e(TAG, "查询结果为空");
msg = 3;
}
} else {
msg = 0;
}
} else {
msg = 0;
}
} catch (Exception e) {
e.printStackTrace();
Log.d(TAG, "异常login:" + e.getMessage());
msg = 0;
}
return msg;
}
/**
* register: 注册
*/
public boolean register(User user) {
HashMap<String, String> map = new HashMap<>();
// 根据数据库名称,建立连接
Connection connection = UserMapper.getConn();
try {
String sql = "insert into enroll(name,password) values (?,?)";
if (connection != null) {// connection不为null表示与数据库建立了连接
PreparedStatement ps = connection.prepareStatement(sql);
if (ps != null) {
//将数据插入数据库
ps.setString(1, user.getName());
ps.setString(2, user.getPassword());
// 执行sql查询语句并返回结果集
int rs = ps.executeUpdate();
if (rs > 0)
return true;
else
return false;
} else {
return false;
}
} else {
return false;
}
} catch (Exception e) {
e.printStackTrace();
Log.e(TAG, "异常register:" + e.getMessage());
return false;
}
}
/**
* findUser: 根据账号进行查找该用户是否存在
*/
public User findUser(String userAccount) {
// 根据数据库名称,建立连接
Connection connection = UserMapper.getConn();
User user = null;
try {
String sql = "select * from enroll where name = ?";
if (connection != null) {// connection不为null表示与数据库建立了连接
PreparedStatement ps = connection.prepareStatement(sql);
if (ps != null) {
ps.setString(1, userAccount);
ResultSet rs = ps.executeQuery();
while (rs.next()) {
//注意:下标是从1开始
String userPassword = rs.getString(1);
String userName = rs.getString(2);
user = new User(userName, userPassword);
}
}
}
} catch (Exception e) {
e.printStackTrace();
Log.d(TAG, "异常findUser:" + e.getMessage());
return null;
}
return user;
}
}
4.MainActivity中的操作
package com.example.mysql;
import androidx.appcompat.app.AppCompatActivity;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.example.mysql.dao.UserDao;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void login(View view) {
EditText EditTextAccount = findViewById(R.id.ed_name);
EditText EditTextPassword = findViewById(R.id.ed_password);
new Thread(){
@Override
public void run() {
UserDao userDao = new UserDao();
int msg = userDao.test(EditTextAccount.getText().toString(),EditTextPassword.getText().toString());
hand1.sendEmptyMessage(msg);
}
}.start();
}
@SuppressLint("HandlerLeak")
final Handler hand1 = new Handler() {
@Override
public void handleMessage(Message msg) {
if (msg.what == 0) {
Toast.makeText(getApplicationContext(), "登录失败", Toast.LENGTH_LONG).show();
} else if (msg.what == 1) {
Toast.makeText(getApplicationContext(), "登录成功", Toast.LENGTH_LONG).show();
} else if (msg.what == 2) {
Toast.makeText(getApplicationContext(), "密码错误", Toast.LENGTH_LONG).show();
} else if (msg.what == 3) {
Toast.makeText(getApplicationContext(), "账号不存在", Toast.LENGTH_LONG).show();
}
}
};
public void start(View view) {
Intent intent = new Intent(MainActivity.this,register.class);
startActivity(intent);
}
}
4.register跳转界面的操作
package com.example.mysql;
import androidx.appcompat.app.AppCompatActivity;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.example.mysql.dao.UserDao;
public class register extends AppCompatActivity {
private static final String TAG = "mysql-factory-register";
EditText name = null;
EditText password = null;
@SuppressLint("MissingInflatedId")
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_register);
name = findViewById(R.id.ed_2_name);
password = findViewById(R.id.ed_2_password);
}
public void login_2(View view) {
String cName = name.getText().toString();
String cPassword = password.getText().toString();
User user = new User();
user.setName(cName);
user.setPassword(cPassword);
new Thread() {
@Override
public void run() {
int msg = 0;
UserDao userDao = new UserDao();
User uu = userDao.findUser(user.getName());
if (uu != null) {
msg = 1;
} else {
boolean flag = userDao.register(user);
if (flag) {
msg = 2;
}
}
hand.sendEmptyMessage(msg);
}
}.start();
}
@SuppressLint("HandlerLeak")
final Handler hand = new Handler() {
public void handleMessage(Message msg) {
if (msg.what == 0) {
Toast.makeText(getApplicationContext(), "注册失败", Toast.LENGTH_LONG).show();
} else if (msg.what == 1) {
Toast.makeText(getApplicationContext(), "该账号已经存在,请换一个账号", Toast.LENGTH_LONG).show();
} else if (msg.what == 2) {
Toast.makeText(getApplicationContext(), "注册成功", Toast.LENGTH_LONG).show();
Intent intent = new Intent();
//将想要传递的数据用putExtra封装在intent中
intent.putExtra("a", "注册");
setResult(RESULT_CANCELED, intent);
finish();
}
}
};
}
5.MainActivity登录界面设计
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:orientation="vertical"
android:layout_marginTop="40dp"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:paddingLeft="20dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="账号:"
android:textSize="25sp"/>
<EditText
android:id="@+id/ed_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
</LinearLayout>
<LinearLayout
android:layout_marginTop="40dp"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:paddingLeft="20dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="密码:"
android:textSize="25sp"/>
<EditText
android:id="@+id/ed_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
<LinearLayout
android:gravity="center"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:text="登录"
android:onClick="login"
android:layout_width="200dp"
android:layout_height="wrap_content"/>
</LinearLayout>
<LinearLayout
android:gravity="center"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:text="注册"
android:onClick="start"
android:layout_width="200dp"
android:layout_height="wrap_content"/>
</LinearLayout>
</LinearLayout>
6.注册界面的设计
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".register">
<LinearLayout
android:orientation="vertical"
android:layout_marginTop="40dp"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:text="账号:"
android:textSize="20sp"
android:paddingLeft="20dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<EditText
android:id="@+id/ed_2_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:text="密码:"
android:textSize="20sp"
android:paddingLeft="20dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<EditText
android:id="@+id/ed_2_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
<Button
android:layout_gravity="center"
android:text="注册"
android:onClick="login_2"
android:layout_marginTop="10dp"
android:layout_width="200dp"
android:layout_height="wrap_content"/>
</LinearLayout>
</LinearLayout>
7.整体界面图
总结
以上就是今天要讲的内容,本文仅仅简单介绍了Android中MySQL数据库的使用,欢迎大家批评指正