演示视频:
基于SpringBoot vue的电脑商城平台源码和论文含支付宝沙箱支付演示视频
支付宝沙箱:
package com.java.controller;
import java.util.*;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.alibaba.fastjson.JSONObject;
import com.alipay.api.AlipayClient;
import com.alipay.api.DefaultAlipayClient;
import com.alipay.api.request.AlipayTradePagePayRequest;
import com.java.utils.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import com.java.model.*;
import com.java.service.*;
;
@RestController
@RequestMapping("/buy")
public class BuyController {
private BuyService buyService;
public BuyService getBuyService() {
return buyService;
}
@Autowired
private ThingTypeService thingtypeService;
@Autowired
private BuyListService buylistService;
@Autowired
private ClientService clientService;
@Autowired
private ThingType2Service thingtype2Service;
@Autowired
public void setBuyService(BuyService buyService) {
this.buyService = buyService;
}
@Autowired
private ThingService thingService;
@Autowired
private BuyingService buyingService;
@Autowired
private HttpServletRequest request;
@Autowired
private HttpServletResponse response;
@CrossOrigin(origins = "*")
@RequestMapping("/add")
@ResponseBody
public JsonBody add(@RequestBody Buying b) {
if(buyingService.GetCount(b)==0)
return Util.SetMap("您的购物车中无任何商品",false);
Buy bl=new Buy();
List<Buying> buying=buyingService.Get(b);
Buy buy=new Buy();
buy.setClientId(b.getClientId());
int id = buyService.Add(buy);
id=buy.getId();
if(id<1)
return Util.SetMap("提交失败",false);
try {
int total = 0;
int count=0;
for(Buying bi:buying)
{
BuyList buylist=new BuyList();
buylist.setBuyId(id);
buylist.setThingId(bi.getThingId());
buylist.setNum(bi.getNum());
int success=buylistService.Add(buylist);
count+=success;
if(success>0)
buyingService.Del(bi.getId());
Thing thing = thingService.GetByID(bi.getThingId());
total+=thing.getPrice();
}
//商户订单号,商户网站订单系统中唯一订单号,必填
String out_trade_no = UUID.randomUUID().toString();
//获得初始化的AlipayClient
AlipayClient alipayClient = new DefaultAlipayClient(AlipayConfig.gatewayUrl, AlipayConfig.app_id, AlipayConfig.merchant_private_key, "json", AlipayConfig.charset, AlipayConfig.alipay_public_key, AlipayConfig.sign_type);
//设置请求参数
AlipayTradePagePayRequest alipayRequest = new AlipayTradePagePayRequest();
//这里设置支付后跳转的地址
alipayRequest.setReturnUrl(AlipayConfig.return_url);
//alipayRequest.setNotifyUrl(AlipayConfig.notify_url);
//付款金额,必填
String total_amount = String.valueOf(total);
//订单名称,必填
String subject ="用户订单";
//商品描述,可空
String body = "";
// 该笔订单允许的最晚付款时间,逾期将关闭交易。取值范围:1m~15d。m-分钟,h-小时,d-天,1c-当天(1c-当天的情况下,无论交易何时创建,都在0点关闭)。 该参数数值不接受小数点, 如 1.5h,可转换为 90m。
String timeout_express = "5m";
alipayRequest.setBizContent("{\"out_trade_no\":\""+ out_trade_no +"\","
+ "\"total_amount\":\""+ total_amount +"\","
+ "\"subject\":\""+ subject +"\","
+ "\"body\":\""+ body +"\","
+ "\"timeout_express\":\""+ timeout_express +"\","
+ "\"product_code\":\"FAST_INSTANT_TRADE_PAY\"}");
//请求
String result = alipayClient.pageExecute(alipayRequest).getBody();
return Util.SetData(result);
// if(count==buying.size())
// return Util.SetMap("下单成功", true);
// else
// return Util.SetMap("提交失败", false);
} catch (Exception e) {
return Util.SetMap(e.getMessage(), false);
}
}
@CrossOrigin(origins = "*")
@RequestMapping("/list")
@ResponseBody
public JsonBody list(@RequestBody Buy b) {
List<Buy> bs= buyService.Get(b);
for(Buy buy:bs)
{
buy.setClient(clientService.GetByID(buy.getClientId()));
}
return Util.SetData(bs);
}
@CrossOrigin(origins = "*")
@RequestMapping("/del")
@ResponseBody
public JsonBody Del(int id) {
Buy b=new Buy();
b.setId(id);
Buy bl=buyService.GetByID(b);
if(bl.getState()>0)
return Util.SetError("该订单已发出,不能取消");
int r = buyService.Del(id);
if(r>0)
return Util.SetMap("退单成功!", false);
else
return Util.SetMap("退单失败!", false);
}
@CrossOrigin(origins = "*")
@RequestMapping("/state")
@ResponseBody
public JsonBody state(@RequestBody Buy bl) {
int r = buyService.EditState(bl);
if(r>0)
return Util.SetMap("操作成功!", true);
else
return Util.SetMap("操作失败!", false);
}
}
package com.java.controller;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.text.SimpleDateFormat;
import java.util.*;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.ibatis.annotations.Param;
import com.alibaba.fastjson.JSONObject;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.system.ApplicationHome;
import org.springframework.stereotype.Controller;
import org.springframework.util.FileCopyUtils;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartHttpServletRequest;
import com.alibaba.druid.support.logging.Resources;
import com.java.utils.CommonUtils;
import com.java.utils.JsonBody;
import com.java.utils.StringUtil;
@RestController
public class UploadController {
String comp=StringUtil.GetProject();
@Autowired
private HttpServletRequest request;
//上传文件
@CrossOrigin(origins = "*")
@RequestMapping("/upload")
@ResponseBody
public JsonBody uploadFile(@RequestBody MultipartFile files) throws IOException {
try {
//E:\my_task\202206\wx19611600068\springboot\src\main\resources\META-INF\resources\file
ApplicationHome applicationHome = new ApplicationHome(this.getClass());
String absolutePath = applicationHome.getDir().getParentFile()
.getParentFile().getAbsolutePath()+"\\src\\main\\resources\\META-INF\\resources\\file\\";
File path = new File(absolutePath);
if (!path.exists()) {
path.mkdirs();//文件夹不存在,创建文件夹
}
String originalFilename = files.getOriginalFilename();//获得原始的文件名 "a.jpg"
int index = originalFilename.lastIndexOf(".");
String newName = originalFilename.substring(0, index) + "_" + UUID.randomUUID().toString() + originalFilename.substring(index);
files.transferTo(new File(absolutePath + newName));//不出现异常,就是文件上传成功
JsonBody jb=Util.SetMap("上传成功",true);
jb.setData("/file/" + newName);
return jb;
} catch (Exception e) {
return Util.SetMap("上传失败,请检查上传接口配置",false);
}
}
private void copyFileUsingFileStreams(File source,File datedirs,String newfilename)
throws IOException {
comp+=comp.toLowerCase().indexOf("webroot")<0?"/WebRoot/file/":"";//同时复制附件到项目下面
//新文件
File newFile = new File(comp+File.separator+datedirs+File.separator+newfilename);
//判断目标文件所在的目录是否存在
if(!newFile.getParentFile().exists()) {
//如果目标文件所在的目录不存在,则创建父目录
newFile.getParentFile().mkdirs();
}
InputStream input = null;
OutputStream output = null;
try {
input = new FileInputStream(source);
output = new FileOutputStream(newFile);
byte[] buf = new byte[1024];
int bytesRead;
while ((bytesRead = input.read(buf)) > 0) {
output.write(buf, 0, bytesRead);
}
} finally {
input.close();
output.close();
}
}
}
扫描二维码关注公众号,回复:
15533676 查看本文章
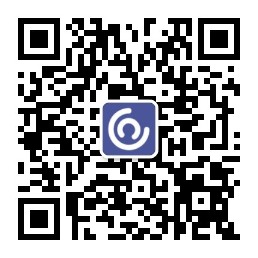
package com.java.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.alibaba.fastjson.JSONObject;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import com.java.model.*;
import com.java.service.*;
import com.java.utils.CommonUtils;
import com.java.utils.JsonBody;
import com.java.utils.PageList;
import com.java.utils.ResponseUtil;;
@RestController
@RequestMapping("/thing")
public class ThingController {
private ThingService thingService;
public ThingService getThingService() {
return thingService;
}
@Autowired
public void setThingService(ThingService thingService) {
this.thingService = thingService;
}
@Autowired
private ThingTypeService thingtypeService;
@Autowired
private ThingType2Service thingtype2Service;
@Autowired
private PingLunService pinglunService;
@Autowired
private HttpServletRequest request;
@Autowired
private HttpServletResponse response;
@CrossOrigin(origins = "*")
@ResponseBody
@RequestMapping("getbyid")
public JsonBody getbyid(int id) {
Map<String,Object> map=new HashMap<String,Object>();
map.put("thingtype", thingtypeService.Get());
map.put("thingtype2", thingtype2Service.Get());
Thing thing=thingService.GetByID(id);
if(id>0)
{
thing.setThingtype(thingtypeService.GetByID(thing.getThingtypeId()));
thing.setThingtype2(thingtype2Service.GetByID(thing.getThingtype2Id()));
}
map.put("add", thing);
return Util.SetData(map);
}
@CrossOrigin(origins = "*")
@ResponseBody
@RequestMapping("add")
public JsonBody add(@RequestBody Thing s) {
int count=0;
if(s.getId()==0)
count = thingService.Add(s);
else
count=thingService.Edit(s);
if(count>0)
return Util.SetMap("操作成功", true);
else
return Util.SetMap("操作失败",false);
}
@CrossOrigin(origins = "*")
@ResponseBody
@RequestMapping("list")
public JsonBody Get(@RequestBody Thing h) {
List<Thing> list = thingService.Get(h);
for(int i=0;i<list.size();i++)
{
ThingType tt=thingtypeService.GetByID(list.get(i).getThingtypeId());
ThingType2 tt2=thingtype2Service.GetByID(list.get(i).getThingtype2Id());
list.get(i).setThingtype(tt);
list.get(i).setThingtype2(tt2);
}
return Util.SetData(list);
}
@CrossOrigin(origins = "*")
@ResponseBody
@RequestMapping("del")
public JsonBody Del(int id) {
if(thingService.Del(id)>0)
return Util.SetMap("删除成功", true);
else
return Util.SetMap("删除失败", false);
}
}