//代码示例如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<style>
div#slider {
width: 1000px;
height: 358px;
background: url('./images/shutter_1.jpg') no-repeat;
display: flex;
justify-content: space-between;
align-items: center;
}
#arrowLeft,
#arrowRight {
width: 49px;
height: 49px;
background: url("images/shutter_prevBtn.png") no-repeat;
margin: 0 20px;
cursor: pointer;
}
#arrowLeft:hover,
#arrowRight:hover {
background-position: center bottom;
}
#arrowRight {
transform: rotate(180deg);
}
#circle {
list-style: none;
overflow: hidden;
align-self: flex-end;
}
#circle li {
float: left;
width: 10px;
height: 10px;
border-radius: 50%;
background-color: white;
margin: 10px 5px;
cursor: pointer;
}
#circle li:first-child {
background-color: gray;
}
</style>
</head>
<body>
<div id="slider">
<div id="arrowLeft"></div>
<ul id="circle">
<li></li>
<li></li>
<li></li>
<li></li>
</ul>
<div id="arrowRight"></div>
</div>
<script>
let slider = document.querySelector('#slider');
let arrowLeft = document.getElementById('arrowLeft');
let arrowRight = document.getElementById('arrowRight');
let lis = document.querySelectorAll('#circle li');
let imagesArr = ['shutter_1.jpg', 'shutter_2.jpg', 'shutter_3.jpg', 'shutter_4.jpg']
扫描二维码关注公众号,回复:
1560780 查看本文章
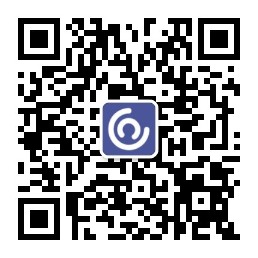
let i = 0;
let timer = setInterval(rightChange, 1000);
// 鼠标移入时暂停计时器
slider.onmouseenter = function () {
clearInterval(timer);
}
// 鼠标移出时重启计时器
slider.onmouseleave = function () {
timer = setInterval(rightChange, 1000)
}
// 点击后向右切换图片
arrowRight.onclick = function () {
rightChange();
}
// 点击后向左切换图片
arrowLeft.onclick = function () {
leftChange();
}
// 四个 li 的点击事件
for (let j = 0; j < lis.length; j++) {
lis[j].onclick = function () {
i = j;
slider.style.background = `url('./images/${imagesArr[i]}') no-repeat`;
circleChange();
}
}
function rightChange() {
i++;
if (i == imagesArr.length) {
i = 0;
}
slider.style.background = `url('./images/${imagesArr[i]}') no-repeat`;
circleChange();
}
function leftChange() {
i--;
if (i == -1) {
i = imagesArr.length - 1;
}
slider.style.background = `url('./images/${imagesArr[i]}') no-repeat`;
circleChange();
}
function circleChange() {
// 四个 li 全变白
for (let k = 0; k < lis.length; k++) {
lis[k].style.backgroundColor = 'white';
}
// 当前 li 变灰
lis[i].style.backgroundColor = 'gray';
}
</script>
</body>
</html>